Node.js Development Trends For Present
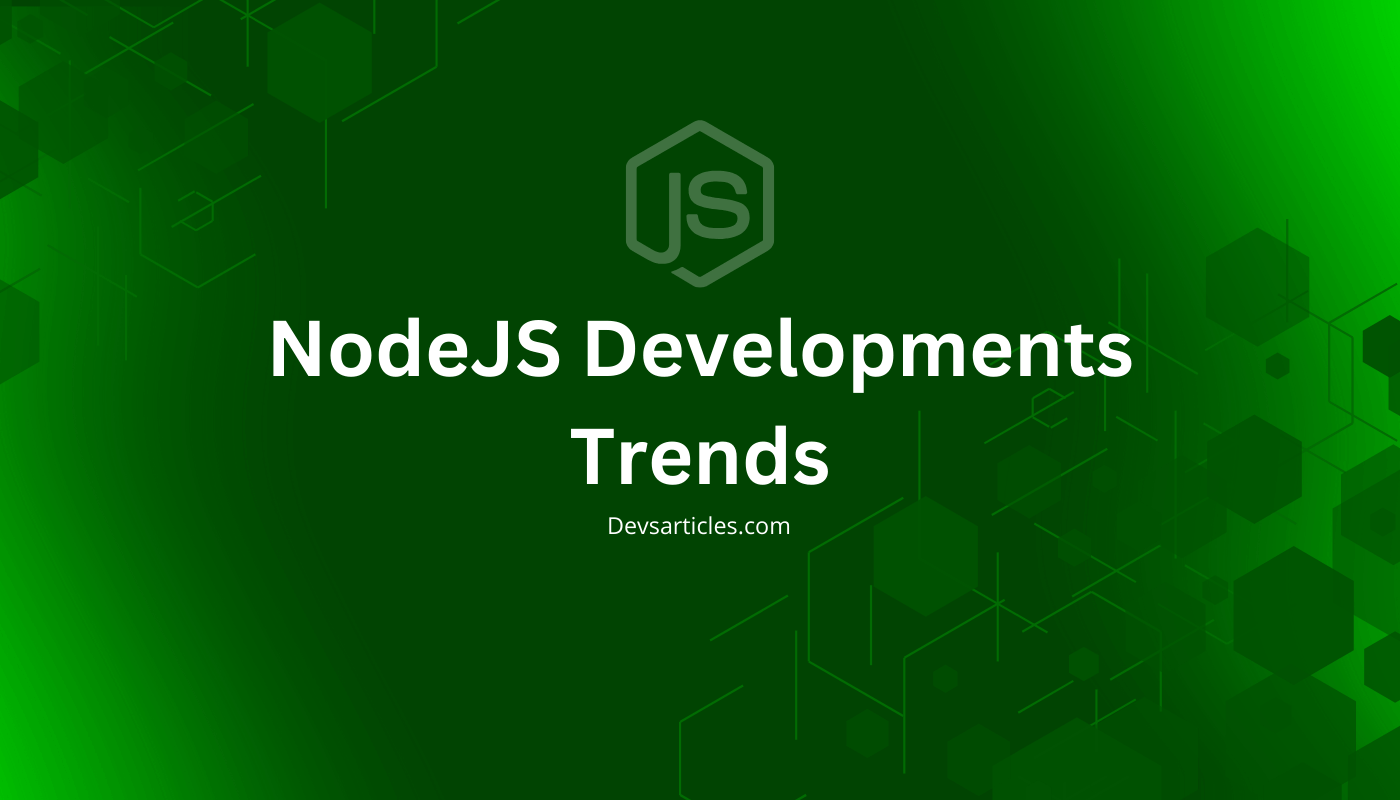
Node.js continues to be a fundamental technology in the web development landscape. Its non-blocking, event-driven architecture makes it an ideal choice for building scalable and efficient applications. This year, several trends are shaping the evolution of Node.js, including the integration of AI, the adoption of TypeScript, and the growing popularity of real-time applications. Let’s explore these trends in detail and understand how they are transforming the development process.
Table of Contents
1. Integration of AI and Machine Learning
Artificial Intelligence (AI) and machine learning are no longer confined to research labs; they have become integral components of modern applications. Node.js is playing a important role in this transformation by enabling developers to integrate AI capabilities seamlessly into their applications.
With libraries like TensorFlow.js, developers can run machine learning models directly in Node.js applications. This allows for real-time data processing and decision-making, which is essential for applications that require instant insights and personalized user experiences. For instance, e-commerce platforms can use AI to offer personalized product recommendations based on user behavior, while customer support systems can automate responses using natural language processing.
Here’s a simple example of using TensorFlow.js with Node.js to predict a value based on input data:
const tf = require('@tensorflow/tfjs-node');
// Define a simple model
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [1] }));
// Compile the model
model.compile({ optimizer: 'sgd', loss: 'meanSquaredError' });
// Generate synthetic data
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
// Train the model
model.fit(xs, ys, { epochs: 250 }).then(() => {
// Use the model to predict a value
model.predict(tf.tensor2d([5], [1, 1])).print();
});
This code snippet demonstrates how to create a simple linear regression model using TensorFlow.js in a Node.js environment. Such integrations allow developers to use the power of AI without leaving the Node.js ecosystem.
2. Adoption of TypeScript
TypeScript has emerged as a game-changer for Node.js developers. As a superset of JavaScript, TypeScript introduces optional static typing, which helps catch errors at compile time and improves code quality. Its features, such as interfaces and type annotations, provide a more structured programming approach, making it easier to manage complex Node.js projects.
The adoption of TypeScript is driven by the need for scalability and maintainability in large-scale applications. By using TypeScript, developers can make sure that their code is more robust and less prone to runtime errors, which is important for building reliable applications.
Here’s a simple example of a Node.js application using TypeScript:
// Import necessary modules
import express, { Request, Response } from 'express';
const app = express();
const port = 3000;
// Define a simple route
app.get('/', (req: Request, res: Response) => {
res.send('Hello, TypeScript with Node.js!');
});
// Start the server
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
In this example, TypeScript’s type annotations provide clarity and help prevent common errors, such as passing incorrect data types to functions. This structured approach is particularly beneficial for teams working on large projects, as it improves collaboration and code readability.
3. Real-Time Applications
The demand for real-time applications is soaring, and Node.js is perfectly suited to meet this demand. Its non-blocking, event-driven architecture allows it to handle multiple connections efficiently, making it ideal for applications that require instant updates.
Real-time applications are prevalent in various sectors, from gaming to finance. For example, online multiplayer games rely on real-time data exchange to provide a seamless gaming experience, while financial platforms use real-time data to update stock prices and execute trades.
Node.js, combined with libraries like Socket.io, enables developers to create chat applications, live notifications, and collaborative tools with ease. Here’s a basic example of a real-time chat application using Node.js and Socket.io:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('chat message', (msg) => {
io.emit('chat message', msg);
});
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
This example demonstrates how to set up a simple chat application that allows users to send and receive messages in real-time. By taking Node.js’s capabilities, developers can create applications that provide instant feedback and enhance user engagement.
4. Serverless Architecture
The serverless architecture is gaining momentum, allowing developers to focus on writing code without worrying about server management. Node.js is a popular choice for serverless environments due to its lightweight nature and fast execution.
In a serverless setup, developers write functions that are triggered by events, such as HTTP requests or database changes. This approach reduces operational costs and complexity, as you only pay for the compute time you use. Serverless platforms like AWS Lambda and Azure Functions support Node.js, making it easy to deploy and scale applications without managing infrastructure.
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify('Hello from Node.js Lambda!'),
};
return response;
};
This code snippet shows a basic Lambda function that responds to HTTP requests. By using serverless architecture, developers can build applications that are highly scalable and cost-effective, allowing them to focus on delivering value to users.
5. Microservices Architecture
Microservices architecture is another trend where Node.js excels. By breaking down applications into small, independent services, developers can achieve greater scalability and flexibility. This architecture improves fault tolerance and makes it easier to update and maintain applications.
Node.js’s lightweight and efficient nature make it ideal for developing microservices that handle high loads and complex interactions. This architecture allows teams to deploy and update services independently, improving development speed and reliability.
Here’s a simple example of a microservice using Node.js and Express:
const express = require('express');
const app = express();
const port = 3001;
app.get('/service', (req, res) => {
res.json({ message: 'Hello from the microservice!' });
});
app.listen(port, () => {
console.log(`Microservice running at http://localhost:${port}`);
});
In this example, the microservice runs independently and can be scaled or updated without affecting other parts of the application. This modular approach is particularly beneficial for large organizations with multiple development teams, as it allows for parallel development and faster deployment cycles.
Conclusion
Node.js continues to evolve, and these trends highlight its adaptability and relevance in modern web development. By embracing these trends, developers can build innovative and efficient applications that meet the demands of today’s users. Whether you’re integrating AI, adopting TypeScript, or exploring real-time applications, Node.js offers the tools and capabilities to bring your ideas to life.
FAQs
Why is TypeScript becoming popular among Node.js developers?
TypeScript is gaining popularity because it offers optional static typing, which helps catch errors at compile time and improves code quality. Its structured approach, including features like interfaces and type annotations, makes it easier to manage large-scale Node.js projects, enhancing maintainability and scalability.