NodeMailer : Complete Mastering Email Sending in NodeJS
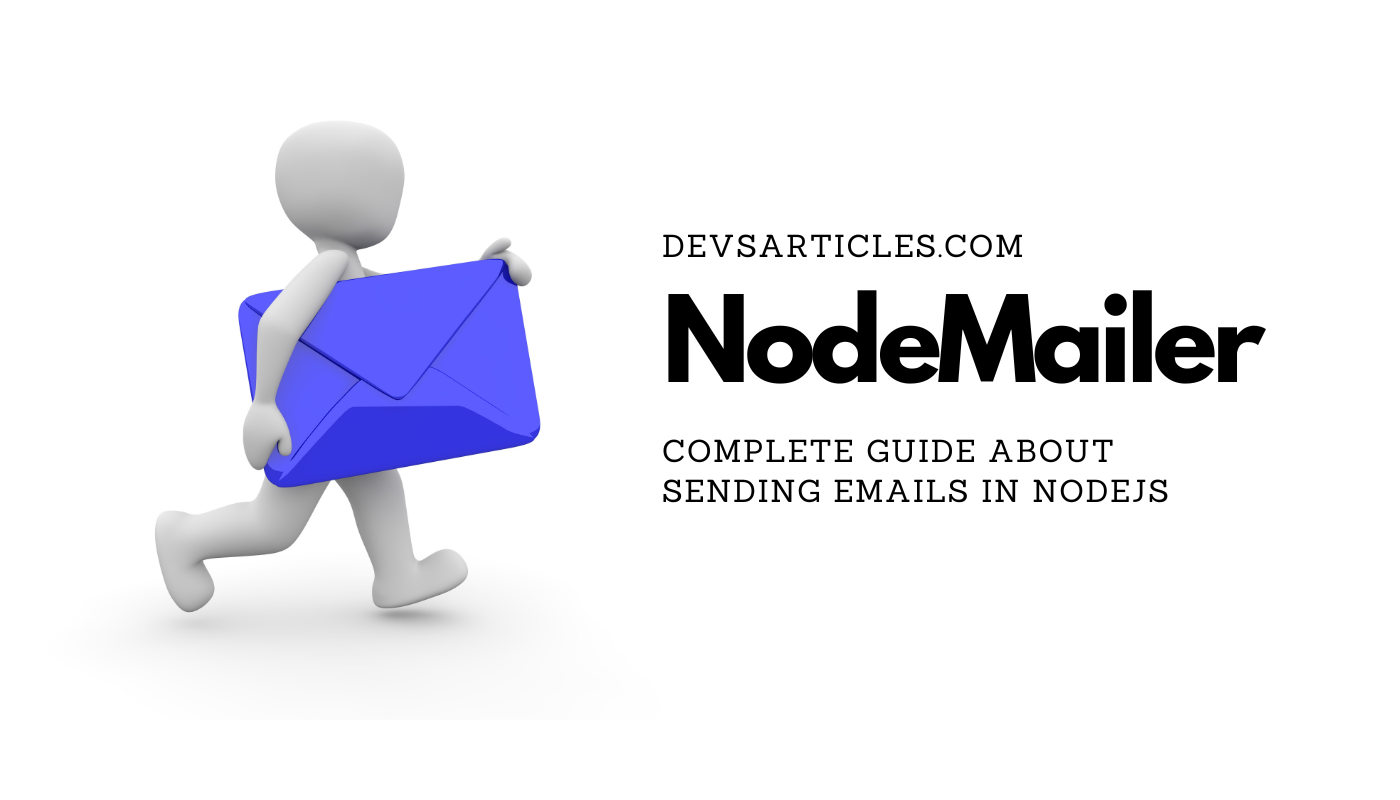
Introduction To NodeMailer
Sending emails is a fundamental requirement for many web applications, and NodeJS provides a powerful solution through the Nodemailer module. Nodemailer is an adaptable email sending library that supports a wide range of email service providers and offers advanced features for customizing and improves your email sending capabilities.
In this complete guide, we’ll explore Nodemailer in-depth, covering everything from basic setup and configuration to advanced options and best practices. Whether you’re new to email sending in NodeJS or looking to take your skills to the next level, this guide will provide you with the knowledge and tools you need to master email sending with Nodemailer.
Step by step guide for Sending mail in nodeJS using NodeMailer
Setting up Nodemailer:
Before we dive into the details, let’s start by installing Nodemailer in your NodeJS project:
npm install nodemailer
Once installed, you can import Nodemailer into your NodeJS file:
const nodemailer = require('nodemailer');
Configuring the Transporter:
The first step in using Nodemailer is to create a transporter, which handles the connection to the email service provider and the email sending process. Here’s an example of how to configure a transporter for Gmail:
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your-email@gmail.com',
pass: 'your-password'
}
});
In this example, we’re using the createTransport method from Nodemailer and providing it with an object that contains the email service provider (service: ‘gmail’) and the authentication details (auth).
If you’re using a different email service provider, you’ll need to modify the service value and potentially provide additional configuration options. Consult the Nodemailer documentation for more information on configuring different email service providers.
Sending Emails:
Once you have your transporter set up, you can send emails using the sendMail method. Here’s an example of how to send a simple email with various options:
const mailOptions = {
from: 'your-email@gmail.com',
to: 'recipient@example.com',
subject: 'Hello from Node.js',
text: 'This is a test email sent from Node.js using Nodemailer.',
html: '<h1>Hello from Node.js</h1><p>This is an HTML email sent using Nodemailer.</p>',
attachments: [
{
filename: 'logo.png',
path: './assets/logo.png',
cid: 'logo'
}
],
alternatives: [
{
contentType: 'text/html',
content: '<h1>Hello from Node.js</h1><p>This is an HTML email sent using Nodemailer.</p><img src="cid:logo" alt="Logo">',
encoding: 'utf-8'
}
]
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.log('Error occurred:', error.message);
} else {
console.log('Email sent successfully:', info.response);
}
});
In this example, we create an object called mailOptions that contains various options for the email we want to send:
- from : The email address of the sender.
- to : The email address of the recipient.
- subject : The subject line of the email.
- text : The plain text content of the email.
- html : The HTML content of the email.
- attachments : An array of attachments to include with the email. In this example, we’re attaching a logo file.
- alternatives : An array of alternative content representations for the email. This is useful when you want to send both plain text and HTML content.
The sendMail method takes two arguments: the mailOptions object and a callback function. The callback function will be executed once the email has been sent or if an error occurs during the sending process.
Full Example With NodeMailer
const nodemailer = require('nodemailer');
const handlebars = require('handlebars');
const fs = require('fs');
const path = require('path');
// Read email template
const emailTemplate = fs.readFileSync(path.join(__dirname, 'templates', 'email.hbs'), 'utf-8');
// Configure transporter with SMTP settings
const transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false, // Use TLS
auth: {
user: 'your-email@example.com',
pass: 'your-password'
},
dkim: {
domainName: 'your-domain.com',
keySelector: 'your-key-selector',
privateKey: 'your-private-key'
}
});
// Compile Handlebars template
const template = handlebars.compile(emailTemplate);
// Email options
const mailOptions = {
from: 'your-email@example.com',
to: 'recipient@example.com',
subject: 'Hello from Node.js',
html: template({
name: 'John Doe',
message: 'This is an email sent using Nodemailer with a Handlebars template.'
}),
attachments: [
{
filename: 'logo.png',
path: './assets/logo.png',
cid: 'logo'
}
],
alternatives: [
{
contentType: 'text/plain',
content: 'This is a plain text version of the email.'
}
]
};
// Send email
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.log('Error occurred:', error.message);
} else {
console.log('Email sent successfully:', info.response);
transporter.on('bounce', (event) => {
console.log('Email bounced:', event);
});
}
});
Advanced Configuration Options for NodeMailer
Nodemailer provides a variety of advanced configuration options that allow you to customize and fine-tune your email sending process. Here are a few examples:
SMTP Configuration
Instead of using a pre-configured email service provider, you can configure Nodemailer to use an SMTP server directly. This is useful if you have a custom SMTP server or if you want to use a third-party email service provider that is not natively supported by Nodemailer.
const transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false,
auth: {
user: 'your-email@example.com',
pass: 'your-password'
}
});
Sending Bulk Emails
Nodemailer supports sending emails in bulk by using the sendMultiple method. This can be useful for sending newsletters or marketing campaigns.
const emails = [
{
from: 'your-email@gmail.com',
to: 'recipient1@example.com',
subject: 'Hello from Node.js',
text: 'This is a test email sent from Node.js using Nodemailer.'
},
{
from: 'your-email@gmail.com',
to: 'recipient2@example.com',
subject: 'Hello from Node.js',
text: 'This is a test email sent from Node.js using Nodemailer.'
}
];
transporter.sendMultiple(emails, (error, info) => {
if (error) {
console.log('Error occurred:', error.message);
} else {
console.log('Emails sent successfully:', info.response);
}
});
Email Templates
Nodemailer supports using email templates, which can help you maintain consistent branding and formatting across your emails. You can use third-party template engines like Handlebars or EJS, or create your own custom templating solution.
Handling Delivery Failures
Nodemailer allows you to handle email delivery failures by listening for specific events, such as failed or bounce. This can help you implement strategies for retrying failed emails or logging delivery issues.
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.log('Error occurred:', error.message);
} else {
console.log('Email sent successfully:', info.response);
transporter.on('bounce', (event) => {
console.log('Email bounced:', event);
});
}
});
Best Practices For Using NodeMailer in NodeJS
When working with Nodemailer and email sending in NodeJS, it’s important to follow best practices to provide reliable and secure email delivery. Here are a few best practices to keep in mind:
- Use Environment Variables: Store sensitive information like email credentials and API keys in environment variables, rather than hardcoding them in your code. This helps maintain security and makes it easier to manage different configurations across different environments (development, staging, production).
- Implement Rate Limiting: Email service providers often have limits on the number of emails you can send within a specific timeframe. Implement rate limiting in your application to avoid hitting these limits and possible account suspension.
- Handle Errors and Retries: Be prepared to handle errors and implement retry mechanisms for failed email deliveries. Nodemailer provides various events and error-handling mechanisms to help you with this.
- Use Email Whitelists: If you’re sending emails to a large number of recipients, consider using email whitelists or confirmed opt-in lists to ensure your emails are delivered to legitimate recipients and to avoid being marked as spam.
- Monitor and Log: Implement monitoring and logging mechanisms to track email sending success rates, delivery failures, bounces, and other metrics. This will help you identify and resolve issues proactively.
Conclusion
Nodemailer is a powerful and flexible email sending library for NodeJS applications. This complete guide has covered everything from basic setup and configuration to advanced features like email templates, attachments, secure connections, and email deliverability best practices.
By using Nodemailer’s robust capabilities, you can easily send plain text or HTML emails, add attachments, use templates, and implement email authentication methods for improved security and reputation management. Additionally, following best practices such as using opt-in lists, maintaining email list hygiene, and monitoring delivery metrics can significantly improve the deliverability and effectiveness of your email communications.
With its extensive documentation and active community, Nodemailer provides a solid foundation for integrating reliable and secure email sending capabilities into your NodeJS applications. Whether you’re building a simple notification system or a complex email marketing platform, Nodemailer equips you with the necessary tools to deliver seamless email communications.
Can I send HTML-formatted emails with Nodemailer?
Yes, Nodemailer supports sending HTML-formatted emails. You can provide the HTML content in the html option of the mailOptions object when sending an email.
How do I attach files to emails with Nodemailer?
To attach files to your emails, you need to specify the attachments option in the mailOptions object. The attachments option accepts an array of attachment objects, each containing properties like filename, path, and cid (for embedding images).
How do I enable secure connections (TLS/SSL) with Nodemailer?
To enable secure connections with Nodemailer, you can set the secure option to true when configuring the transporter, or specify the appropriate port for secure connections (e.g., 465 for SSL, 587 for TLS).
Can I send bulk emails with Nodemailer?
Yes, Nodemailer supports sending bulk emails by using the sendMultiple method. This method allows you to send multiple emails in a single operation, which can be useful for sending newsletters or marketing campaigns.