Exploring NodeJS Express Logging: A Comprehensive Guide about winston logger
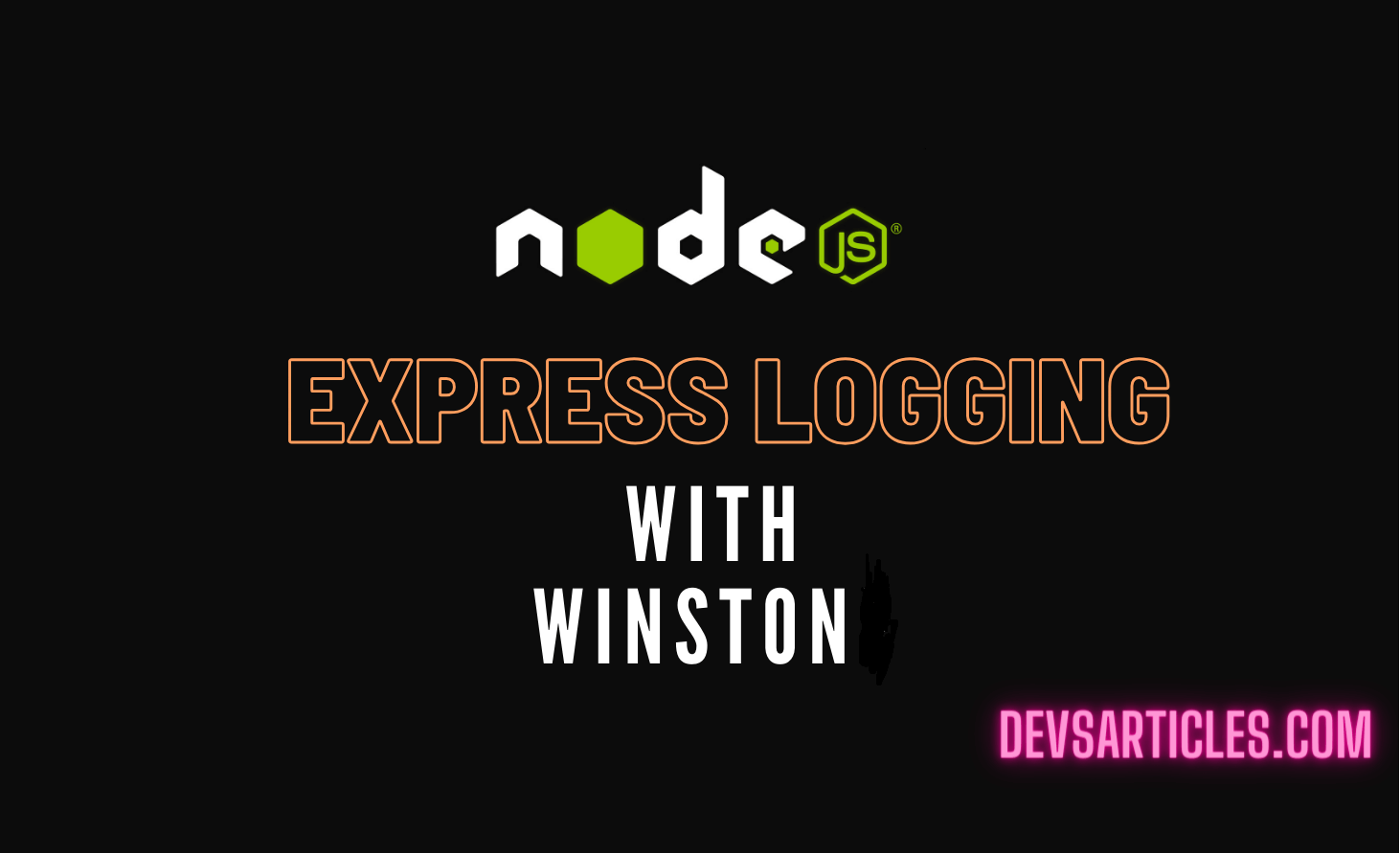
Express Logging is not just a detail but a important element for web applications. Knowing how important logging is, exploring the different types of logs and understanding the challenges of fixing issues without proper logs including error logs is essential for developers.
In this blog we will discuss about node express logging
Table of Contents
important role in express logging
- Error Detection and Troubleshooting : Imagine logging as a watchful guard protecting your application. When things go wrong while the app is running at that time logs act like detailed reporters that giving instant information about the problem. This is quick way to find and fix issues during development is super valuable.
- Performance Insights : Logging is not only about finding mistakes. It becomes a performance expert. It carefully keeps an eye on important measures like how fast the app responds, database queries and calls to external services. By looking into these detailed logs so the developers get a detailed understanding and finding chances to make things work better and making sure users have a smooth experience.
- Security Auditing : Think of logging as a record-keeper for your application’s safety. It keeps track of everything that happens. Making it super usefull for checking how secure your app is. Whether it’s spotting someone trying to get in without permission or looking closely at things that seem suspicious,.Detailed logs are really important for keeping your app safe.
Trying to find your way without the right logs
- Limited View Challenge : Without the right logs developers are like people trying to go through a maze with their eyes covered. Not having a clear understanding of how the app works on the inside makes it tough to follow what the code is doing. This limited view makes fixing problems take much longer and needing more work to figure out and solve issues.
- Issue Reproduction Complexity : Dealing with bugs without good logs is like trying to solve a puzzle without all the pieces. Making bugs happen again becomes a tricky job because developers don’t have a clear record of what the app was like in certain situations. Detailed logs are like the missing pieces that giving a structured way to recreate conditions for better fixing bugs and solving problems.
- Time-Consuming Debugging : Trying to debug without good logs is like looking for a needle in a haystack. Developers end up checking things manually, trying different things and making guesses. On the other hand with organized logs are like a map that giving a structured way to look at what the app is doing and cutting down the time it takes to find and fix problems.
Understanding the log types
- Application Logs : Record information about the application’s execution, including errors, warnings and information messages
- Access Logs : Capture details about incoming requests and providing insights into user interactions and resource utilization.
- Error Logs : Specifically focus on recording details about errors and exceptions that can help developers in pinpointing and resolving issues.
- Security Logs : Concentrate on recording security-related events that helping detect and respond to potential threats.
winston for nodeJS express logging
When it comes to NodeJS logging and ExpressJS projects. Developers have a bunch of library options. But Winston is a popular choice because it has a lot of cool features and can adapt well to different needs. Let’s take a quick look at some other libraries before we go deep into why Winston is special.
Logging Library Options
- Bunyan
- Bunyan is known for putting a lot of importance on organized logs. Which is great for projects that need logs to be well-structured and easy for machines to read.
- It’s also famous for being able to handle a lot of data that making it a good choice for big applications and systems spread across multiple places.
- Pino :
- Pino is well-liked for being fast and not using up a lot of resources that making it a good fit for projects that want to keep things simple.
- Just like Bunyan Pino is all about creating logs in a JSON format. Which makes it easy to work with different log analysis tools.
Why Winston Stands Out for express logging
- Customizable Choices : Winston’s modular design lets developers create their own logging setups by picking and using only the parts they need. This flexibility makes it a good fit for different kinds of projects.
- Detailed Settings : Winston’s various logging levels provide detailed control over log messages that helping to sort and prioritize them efficiently according to their severity.
- Always Improving : With a big and engaged community Winston keeps getting better through ongoing development and support. This means it stays up-to-date with the best ways of doing things and adapts to new trends in logging.
- Personalized Log Style : With Winston developers can create log messages in their own custom formats. This gives the freedom to make logs to specific needs or smoothly fit them into existing systems.
- Keeping it Quick : Winston’s ability to handle asynchronous logging is important for keeping your application running smoothly. It ensures that log actions don’t slow down the main part of the program.
- Smooth Connection : Winston easily fits into ExpressJS using middleware that automatically logging HTTP requests and responses. This improves the ability to debug and monitor Express logging applications.
setting-up winston for express logging
Logging is a important part of keeping Express applications in good shape and Winston is a reliable library for it. This guide takes you through the simple steps of installing and setting up Winston in your nodeJS Express application to make sure strong and effective logging. I hope that you have basic knowledge of setting up express server
install Winston
Open your terminal and navigate to your Express project directory. Run the following command to install Winston using npm:
npm install winston
This command installs the Winston library and its dependencies into your project.
configure express logger
It’s not mandatory creating a separate configuration file for Winston settings.But setting up separate file can enhance manageability. Create a new file e.g. winston-config.js
and set up your logging configurations. For example:
// winston-config.js
const { createLogger, transports, format } = require('winston');
const logger = createLogger({
transports: [
new transports.Console({
format: format.combine(
format.colorize(),
format.simple()
),
}),
new transports.File({
filename: 'logs/error.log',
level: 'error',
format: format.combine(
format.timestamp(),
format.json()
),
}),
// Add more transports as needed
],
});
module.exports = logger;
This configuration includes a console transport for displaying logs in the console and a file transport for saving error logs to a file.
Integrate Winston with Express For logging
In your Express application file (e.g. app.js
or index.js
) require the Winston configuration and integrate it into your application. Add the following lines at the top of your file:
const express = require('express');
const logger = require('./winston-config'); // Adjust the path as needed
const app = express();
// ... (your existing Express.js setup)
// Use the Winston logger middleware for Express
app.use((req, res, next) => {
logger.info(`${req.method} ${req.url}`);
next();
});
// ... (the rest of your Express.js routes and middleware)
// Error handling middleware
app.use((err, req, res, next) => {
logger.error(`${err.status || 500} - ${err.message} - ${req.originalUrl} - ${req.method} - ${req.ip}`);
// Handle the error and send an appropriate response to the client
res.status(err.status || 500).send('Internal Server Error');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Now Winston is integrated into the Express application as middleware. Requests and errors are logged using the configured Winston Express logger.
time to test our express logging function
Run your Express logger application and make some requests. Check the console for log outputs and the specified log file for error logs.
That’s it. You successfully set up Winston in your Express application that providing a robust logging solution for better debugging and monitoring capabilities. Adjust the configurations as needed for your specific project requirements.
winston transport option for your express logging
Winston a flexible logging library that provides various transport options to suit different logging requirements in NodeJS applications. Transports decide where log messages go and giving you the freedom to adapt. Let’s check out the main Winston transport choices like console, file and database and talk about how to choose the best one for your particular needs.
Console Transport
The console transport is the simplest and most straightforward option. It outputs log messages directly to the console that making it convenient for development and debugging purposes.
- Ideal for debugging and development as logs are readily visible in the console
- Offers immediate insights into the application’s behaviour during development.
const { createLogger, transports } = require('winston');
const logger = createLogger({
transports: [
new transports.Console(),
],
});
file transport
The file transport directs log messages to a specified file. This option is very good for long-term storage and reference. Specially in production environments.
- Suitable for logging in production environments where long-term storage and analysis are required.
- good for meeting compliance requirements or auditing purposes.
const { createLogger, transports } = require('winston');
const logger = createLogger({
transports: [
new transports.File({
filename: 'logs/application.log',
}),
],
});
database transport
Winston also allows sending log messages to databases that make centralization and easy integration with other systems. This transport often involves using a custom database transport or integrating with existing database systems.
- Suitable for scenarios where logs need to be stored centrally for multiple applications or microservices.
- Useful when logs need to be stored alongside other application data in a database.
const { createLogger, transports } = require('winston');
const customDatabaseTransport = new transports.CustomDatabaseTransport({
// Configure the custom database transport options
});
const logger = createLogger({
transports: [
customDatabaseTransport,
],
});
things before Selecting the Right Transport for express logger
- Development vs Production :
- Choose the console transport for development to quickly view logs.
- Use file or database transports in production for long-term storage and analysis.
- Storage Requirements :
- File transport is suitable for scenarios requiring log file storage.
- Database transport is beneficial for centralized storage in a database.
- Visibility and Accessibility :
- Console transport provides immediate visibility during development.
- File or database transports enable easy access and analysis of logs over time.
- Integration Requirements :
- Consider database transport when logs need to integrate with existing database systems.
- Compliance and Auditing :
- File or database transport is preferable for compliance and auditing purposes.
By understanding these Winston transport options and considering the specific needs of your application. you can effectively choose the right log destination for your express logging requirements.
Customizing Log Formats with Winston that makes express logging readable
Winston offers strong formatting features that allowing developers to personalize log messages based on their preferences and project needs. Customizing log formats improves how easily log data can be read, analyzed and understood. Let’s explore how you can use Winston’s formatting capabilities to create more personalized and readable log outputs.
Basic Log Formatting
Winston allows you to set up basic formatting options to include essential information such as timestamps, log levels and the actual log message content.
const { createLogger, format, transports } = require('winston');
const logger = createLogger({
format: format.combine(
format.timestamp(),
format.simple()
),
transports: [
new transports.Console(),
],
});
logger.info('Customizing log formats for better readability.');
In this example format.timestamp()
adds a timestamp to each log entry and format.simple()
simplifies the log message format.
Adding Colors on express logging
Adding colors to log messages can enhance visual separation and emphasize different parts of the log entry.
const { createLogger, format, transports } = require('winston');
const logger = createLogger({
format: format.combine(
format.timestamp(),
format.simple(),
format.printf(info => {
return `${info.timestamp} ${info.level}: \x1b[36m${info.message}\x1b[0m`;
})
),
transports: [
new transports.Console(),
],
});
logger.info('Adding colors for emphasis.');
In this example format.printf
allows you to define a custom output format using colors.\x1b[36m
and \x1b[0m
add cyan color to the log message.
Structuring Log Entries
You can structure log entries using JSON formatting for better organization and easier parsing.
const { createLogger, format, transports } = require('winston');
const logger = createLogger({
format: format.combine(
format.timestamp(),
format.json()
),
transports: [
new transports.Console(),
],
});
logger.info('Structuring log entries for improved readability.', { key: 'value' });
In this example format.json()
structures log entries in JSON format that accommodating additional data passed as an object.
Customizing Further with winston.format.label
Winston’s winston.format.label
allows you to add labels to log entries and providing additional context.
const { createLogger, format, transports } = require('winston');
const logger = createLogger({
format: format.combine(
format.timestamp(),
format.label({ label: 'Custom Label' }),
format.simple()
),
transports: [
new transports.Console(),
],
});
logger.info('Customizing log formats with additional labels.');
By combining these formatting options. Developers can create customized log formats that suit their preferences and project requirements. Whether it’s adding colors, structuring log entries or incorporating additional context with labels. Winston’s formatting options provide a flexible and powerful way to enhance the readability of log messages in NodeJS express logging in applications.
summary
Effective nodeJS logging is vital for web applications particularly in NodeJS and Express development. Winston stands out as a flexible and detailed logging library that offers customizable choices and robust features.
To get Winston ready to use. you just have to do a few easy things. First put it on your system. Then set it up the way you like it. If you’re using Express make sure Winston is part of it.
Winston has two handy features. one for when you are working on your project and want to see what’s going on right in front of you (console) and another for when you’re ready to share it with the world and need to keep records (file). It’s a simple process that helps you see what’s happening during development and saves important info for later when your project is live.
Winston’s formatting options allow developers to personalize log messages, add colors, structure entries and include context with labels. This makes log readable and quicker issue resolution and improved application understanding.
Choosing Winston for NodeJS Express logging ensures a streamlined and efficient development process and empowering developers to troubleshoot errors and gain performance insights and maintain application security. Winston is not just a logging tool. it’s a necessity for building reliable and secure web applications.
I hope you like this Express Logging deep guide
fAQs
Why is Winston a popular choice for NodeJS Express logging ?
Winston’s modular design, detailed settings, ongoing development and personalized log style make it a standout choice. Its flexibility allows developers to make logging setups that ensuring compatibility with various project needs and enhancing debugging capabilities.
What are the transport options in Winston for Express logging ?
Winston provides various transport options. Including Console Transport (for immediate visibility), File Transport (for long-term storage) and Database Transport (for centralizing logs). The choice depends on development versus production needs, storage requirements, visibility, integration and compliance.