Designing Efficient MongoDB Schemas for High Performance Applications
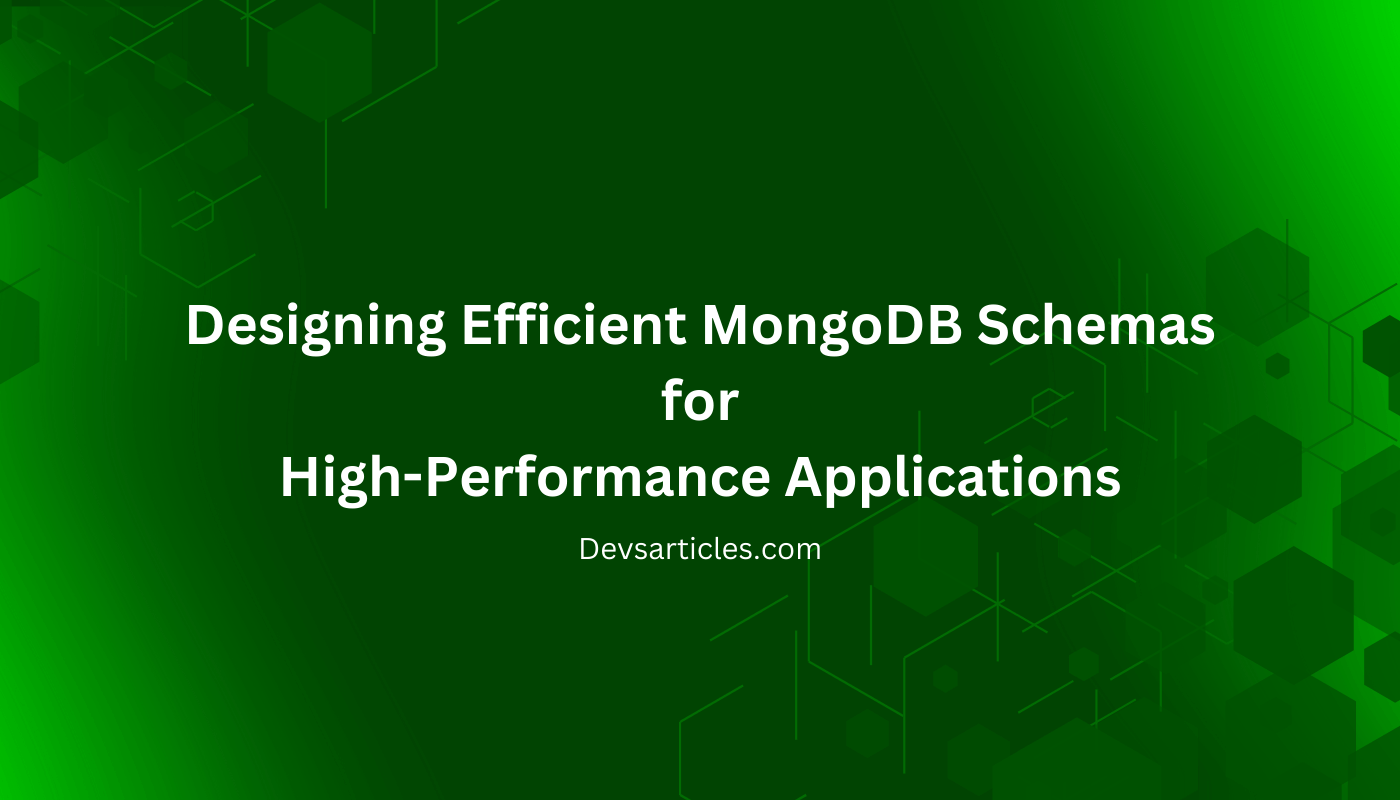
MongoDB, a popular NoSQL database, has gained significant traction in recent years due to its flexibility, scalability, and ability to handle large volumes of unstructured data. However, designing an efficient schema is important for high performance, optimal resource utilization, and seamless scalability. In this complete blog post, we’ll dive deep into the best practices and techniques for designing MongoDB schemas that cater to high-performance applications.
Table of Contents
Understanding MongoDB Schema Design Principles
Before exploring specific techniques, let’s establish a solid foundation by understanding the fundamental principles of MongoDB schema design:
1. Embedding vs. Referencing
MongoDB offers two primary approaches for modeling relationships between data, embedding and referencing.
Embedding involves nesting related data within a single document. This approach provides excellent read performance and data locality, as all the required information is readily available within the same document. Embedding is suitable when the embedded data is not frequently updated and has a one-to-one or one-to-many relationship with the parent document.
// Embedding
{
"_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e5f"),
"name": "John Doe",
"address": {
"street": "123 Main St",
"city": "New York",
"country": "USA"
},
"orders": [
{
"orderId": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6b"),
"productName": "iPhone 12",
"quantity": 1
},
{
"orderId": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6c"),
"productName": "MacBook Pro",
"quantity": 1
}
]
}
On the other hand, referencing involves storing references to related data in separate documents. This approach enables flexibility and avoids data duplication, as the referenced data can be shared across multiple documents. Referencing is suitable when the referenced data is frequently updated, has a many-to-many relationship, or when the embedded data grows unbounded.
// Referencing
{
"_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e5f"),
"name": "John Doe",
"address_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6a")
}
{
"_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6a"),
"street": "123 Main St",
"city": "New York",
"country": "USA"
}
{
"_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6b"),
"userId": ObjectId("5f1f5a5d9c5d5e5f5a5d5e5f"),
"productName": "iPhone 12",
"quantity": 1
}
{
"_id": ObjectId("5f1f5a5d9c5d5e5f5a5d5e6c"),
"userId": ObjectId("5f1f5a5d9c5d5e5f5a5d5e5f"),
"productName": "MacBook Pro",
"quantity": 1
}
2. Denormalization
Denormalization is a technique that involves duplicating data across multiple documents to optimize read performance. By denormalizing data, you can reduce the need for expensive joins and improve query efficiency. Denormalization is particularly useful when the denormalized data is frequently accessed together and rarely updated.
However, it’s essential to strike a balance and avoid excessive denormalization, as it can lead to data inconsistency and increased storage requirements. Carefully evaluate the read-to-write ratio and the data consistency requirements of your application before applying denormalization.
3. Data Locality
Data locality refers to the principle of organizing data that is frequently accessed together in the same document or closely related documents. By ensuring data locality, you can minimize disk I/O and network latency, resulting in faster query performance.
When designing your schema, consider the common access patterns and group related data together. This approach allows MongoDB to efficiently retrieve the required data in fewer operations, improving overall performance.
Optimizing Schema Design for High-Performance Applications
With a solid understanding of the fundamental principles, let’s explore specific techniques for designing high-performance MongoDB schemas:
1. Indexing Strategies
Indexes play a crucial role in optimizing query performance in MongoDB. By creating indexes on frequently queried fields, you can significantly reduce the time required to locate and retrieve data. MongoDB supports various types of indexes, including single-field, compound, multikey, geospatial, and text indexes.
When designing your schema, identify the fields that are commonly used in queries and create appropriate indexes. Use single-field indexes for queries that filter on a single field and compound indexes for queries that involve multiple fields.
// Creating a single-field index on the "name" field
db.users.createIndex({ name: 1 })
// Creating a compound index on "name" and "age" fields
db.users.createIndex({ name: 1, age: 1 })
However, be cautious when indexing large datasets, as excessive indexing can impact write performance and consume significant storage space. Regularly monitor and analyze query patterns to identify the most beneficial indexes for your application.
2. Minimizing Data Duplication
While denormalization can improve read performance, it’s require to minimize excessive data duplication. Duplicating data across multiple documents can lead to increased storage requirements and potential data inconsistency issues.
When designing your schema, consider the trade-offs between embedding and referencing. Use embedding when the embedded data is not frequently updated and has a one-to-one or one-to-many relationship with the parent document. Use referencing when the referenced data is frequently updated or grows unbounded.
3. Designing for Efficient Queries
Efficient query design is crucial for high-performance applications. When structuring your schema, consider the common query patterns and optimize the schema accordingly.
If certain fields are frequently queried together, consider embedding them within the same document. This approach reduces the need for additional queries and improves query performance.
// Efficient query using embedded data
db.users.find({ "address.city": "New York" })
For complex queries and data transformations, take advantage of the power of MongoDB’s aggregation framework. The aggregation pipeline allows you to perform advanced data processing, filtering, grouping, and transformation operations efficiently.
// Aggregation pipeline example
db.orders.aggregate([
{ $match: { status: "completed" } },
{ $group: { _id: "$userId", totalSpent: { $sum: "$amount" } } },
{ $sort: { totalSpent: -1 } },
{ $limit: 10 }
])
4. Sharding and Partitioning
As your application scales and the data volume grows, sharding becomes essential to distribute the data across multiple machines. Sharding allows you to horizontally partition your data based on a shard key, enabling better scalability and performance.
When designing your schema for sharding, consider the following:
- Choose a shard key that aligns with your query patterns and ensures even data distribution across shards.
- Avoid using monotonically increasing shard keys, as they can lead to data imbalance and hotspots.
- Consider the cardinality of the shard key to ensure effective data distribution.
Common Pitfalls and Mistakes to Avoid
While designing MongoDB schemas, be aware of these common pitfalls and mistakes:
- Overembedding: Embedding too much data within a single document can lead to document size limitations (16MB per document) and performance issues. Be cautious when embedding large arrays or deeply nested structures.
- Inefficient Indexing: Creating unnecessary indexes or indexing on large fields can impact write performance and consume significant storage space. Regularly review and optimize your indexes based on the actual query patterns.
- Unbounded Array Growth: Avoid designing schemas that allow unbounded array growth within documents. Large and growing arrays can cause performance degradation and make data management challenging. Consider alternative approaches, such as using separate documents or capped collections.
- Neglecting Data Consistency: While denormalization can improve read performance, it’s crucial to maintain data consistency. Implement appropriate mechanisms, such as atomic operations or data validation, to ensure data integrity across denormalized fields.
Conclusion
Designing efficient MongoDB schemas is a important aspect of building high-performance applications. By understanding the principles of embedding, referencing, denormalization, and data locality, you can optimize your schema for specific query patterns and scalability requirements.
Remember to carefully consider indexing strategies, minimize data duplication, and design for efficient queries. Regularly monitor and analyze query performance to identify areas for optimization and make informed decisions about schema evolution.
By following these best practices, avoiding common pitfalls, and continuously refining your schema design, you can get the full potential of MongoDB and build applications that scale seamlessly while delivering exceptional performance.
FAQs
How do indexes improve query performance in MongoDB?
Indexes in MongoDB help optimize query performance by allowing the database to quickly locate and retrieve data without scanning the entire collection. By creating indexes on frequently queried fields, you can significantly reduce the time required to execute queries. However, be mindful of the impact on write performance and storage space when creating indexes.
What is the difference between embedding and referencing in MongoDB schema design?
Embedding involves nesting related data within a single document, providing better read performance and data locality. Referencing involves storing references to related data in separate documents, enabling flexibility and avoiding data duplication. Embedding is suitable for one-to-one or one-to-many relationships, while referencing is preferred for frequently updated data or many-to-many relationships.