Scaling Node.js Applications with the Cluster Module: A Comprehensive Guide
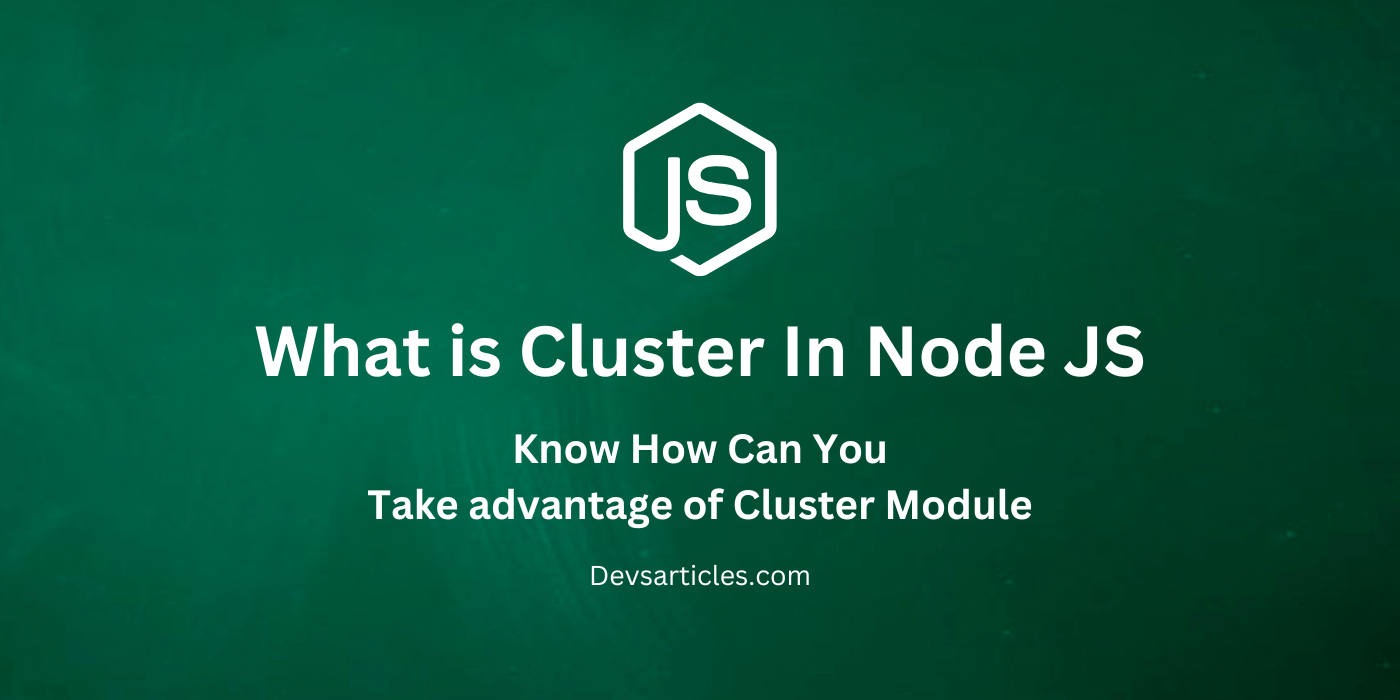
Introduction
In the world of Node.js development, performance and scalability are important. While Node.js is known for its efficiency in handling asynchronous operations, it’s naturally single-threaded. This is where the Cluster module comes in, offering a powerful solution to harness the full potential of multi-core systems. In this guide, we’ll explore how the Cluster module can transform your Node.js applications, making them more robust and capable of handling increased loads.
Table of Contents
What is the Cluster Module?
The Cluster module is a built-in Node.js module that allows you to create child processes, called workers, which run simultaneously and share the same server port. This approach enables your Node.js application to distribute incoming connections or requests across multiple CPU cores, significantly improving performance and reliability.
Key Benefits of Using the Cluster Module:
- Improved performance on multi-core systems
- Better resource utilization
- Increased application reliability
- Simplified scaling of Node.js applications
How the Cluster Module Works
The Cluster module operates on a master-worker pattern. Here’s a breakdown of the process:
- The master process is responsible for forking worker processes.
- Worker processes are instances of your Node.js application.
- When a new connection comes in, the master process distributes it to a worker using a round-robin algorithm by default.
Let’s look at a basic example:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers.
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`worker ${worker.process.pid} died`);
});
} else {
// Workers can share any TCP connection
// In this case, it is an HTTP server
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
In this example, the master process creates worker processes equal to the number of CPU cores. Each worker then sets up an HTTP server listening on port 8000.
Advantages of Using the Cluster Module
- Improved Performance: By distributing the workload across multiple cores, your application can handle more requests simultaneously.
- Increased Reliability: If a worker process crashes, the master can spawn a new one, ensuring your application remains available.
- Simplified Scaling: The Cluster module makes it easy to scale your application horizontally on a single machine.
- Load Balancing: The master process automatically load balances incoming connections across workers.
Implementing the Cluster Module in Your Application
To implement the Cluster module effectively, follow these steps:
Determine the Number of Workers: Usually, this is set to the number of CPU cores available:
const numCPUs = require('os').cpus().length;
Create Worker Processes: Use a loop to fork worker processes:
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
Handle Worker Events: Set up event listeners for worker lifecycle events:
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
cluster.fork(); // Replace the dead worker
});
Implement Your Server Logic: In the worker processes, implement your server logic as you normally would.’
Best Practices for Using the Cluster Module
Graceful Shutdown: Implement a graceful shutdown mechanism to ensure all requests are processed before the server stops:
process.on('SIGTERM', () => {
console.log('Graceful shutdown initiated');
server.close(() => {
console.log('Server closed');
process.exit(0);
});
});
Sticky Sessions: For applications requiring session persistence, implement sticky sessions to ensure requests from a client always go to the same worker:
const cluster = require('cluster');
if (cluster.isMaster) {
cluster.schedulingPolicy = cluster.SCHED_RR;
}
Monitor Worker Health: Regularly check the health of your worker processes and restart them if necessary.
Use Environment Variables: Use environment variables to pass configuration to worker processes instead of command-line arguments.
Advanced Features of the Cluster Module
Inter-Process Communication (IPC)
The Cluster module allows communication between the master and worker processes:
if (cluster.isMaster) {
const worker = cluster.fork();
worker.send('Hi there');
} else {
process.on('message', (msg) => {
console.log('Message from master:', msg);
});
}
Zero-Downtime Restarts
Implement zero-downtime restarts by gradually replacing old workers with new ones:
if (cluster.isMaster) {
let workerCount = 0;
cluster.on('exit', (worker, code, signal) => {
if (!worker.exitedAfterDisconnect) {
cluster.fork();
}
});
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
process.on('SIGUSR2', () => {
const workers = Object.values(cluster.workers);
const restartWorker = (workerIndex) => {
const worker = workers[workerIndex];
if (!worker) return;
worker.on('exit', () => {
if (!worker.exitedAfterDisconnect) return;
cluster.fork().on('listening', () => {
restartWorker(workerIndex + 1);
});
});
worker.disconnect();
};
restartWorker(0);
});
}
Comparing Cluster Module with Worker Threads
While both the Cluster module and Worker Threads enable parallel processing in Node.js, they serve different purposes:\
- Cluster Module: Ideal for scaling Node.js servers and distributing incoming network connections.
- Worker Threads: Better for CPU-intensive tasks and parallel processing within a single Node.js process.
Choose the Cluster module when you need to scale your HTTP server, and Worker Threads when you have CPU-bound tasks that can run in parallel.
Conclusion
The Cluster module is a powerful tool in the Node.js ecosystem, enabling developers to build scalable and reliable applications that can fully utilize multi-core systems. By distributing the workload across multiple processes, you can significantly improve your application’s performance and resilience.
Remember, while the Cluster module is excellent for scaling Node.js servers, it’s not a one-size-fits-all solution. Always consider your specific use case and requirements when deciding between the Cluster module, Worker Threads, or other multithreading approaches in Node.js.
With the knowledge gained from this guide, you’re now equipped to implement the Cluster module in your Node.js applications, taking a significant step towards building more scalable and efficient server-side JavaScript applications.
FAQs
Does the Cluster module work with all types of Node.js applications?
The Cluster module is most beneficial for network servers, particularly HTTP servers. It may not provide significant benefits for CPU-bound applications.
How does the Cluster module handle shared resources?
Each worker runs in its own process with its own memory space. Shared resources should be managed carefully, often through a shared database or cache.
Can I use the Cluster module in combination with Worker Threads?
Yes, you can use the Cluster module to scale across multiple cores and then use Worker Threads within each worker for CPU-intensive tasks.