Mastering cloudinary Image Upload in Next.JS: A Comprehensive Guide
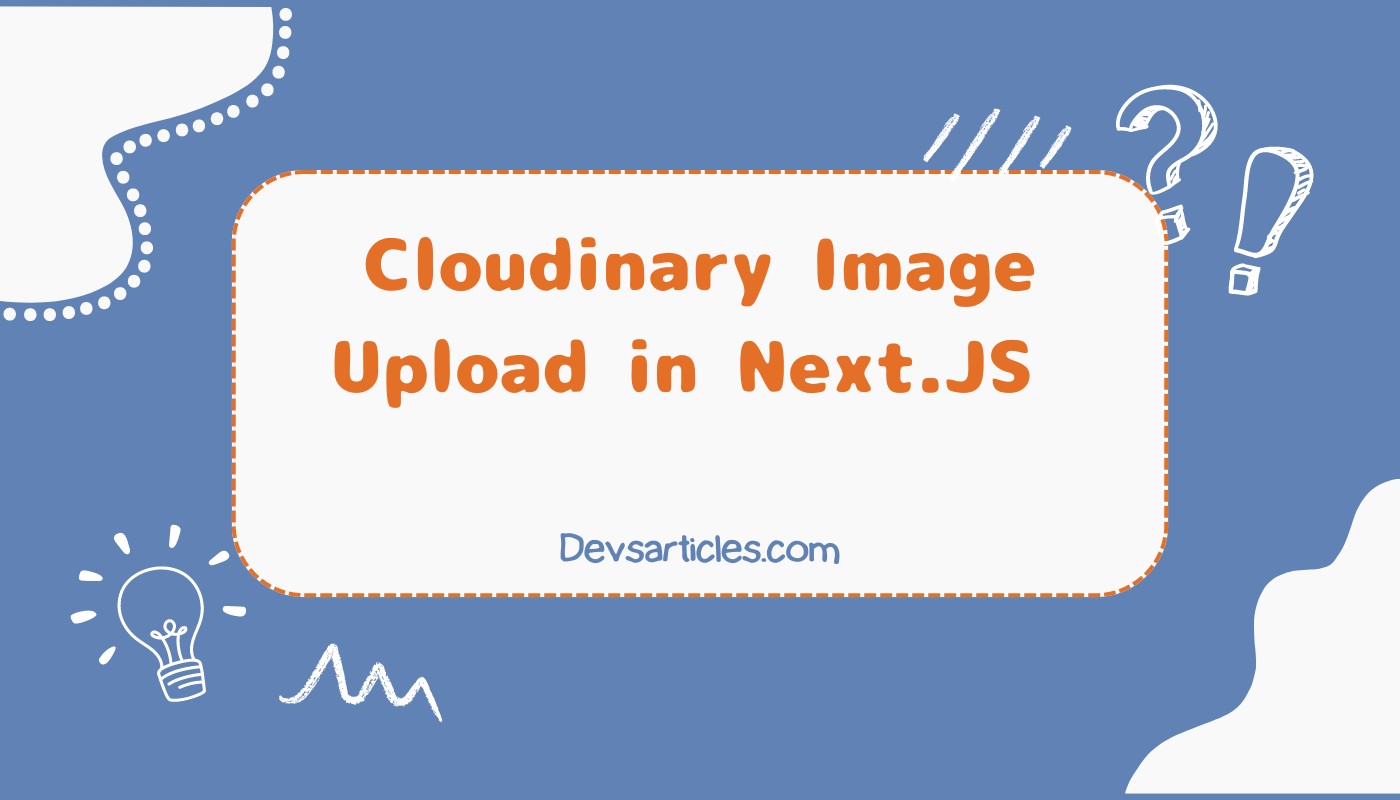
Introduction
In the world of web development, efficiently handling image uploads is important for creating dynamic and engaging applications. This comprehensive guide will walk you through integrating Cloudinary’s powerful image management capabilities into your Next.js project. Whether you’re building an e-commerce platform, a social media app, or any project requiring robust image handling, this tutorial has got you covered.
By the end of this guide, you’ll have a fully functional image upload system that use the power of Next.js and Cloudinary, complete with server-side and client-side implementations.
Table of Contents
Prerequisites
Before we dive in, Make sure you have:
- Node.js and npm installed on your system
- A basic understanding of Next.js and React
- Familiarity with TypeScript
- A Cloudinary account (free tier is sufficient)
Let’s start this exciting journey to supercharge your Next.js application with Cloudinary’s image management capabilities!
Setting Up Your Next.JS Project
If you haven’t already, let’s start by creating a new Next.JS project with TypeScript support:
npx create-next-app@latest my-cloudinary-app --typescript
cd my-cloudinary-app
Follow the prompts to set up your project. For this guide, we’ll use the App Router.
Setting Up Cloudinary in Your Next.JS Project
Step 1: Installing the Cloudinary Package
First, let’s add the Cloudinary package to your project. Open your terminal and run:
npm install cloudinary@2.0.0
This command installs the latest 2.x version of Cloudinary, which is compatible with Next.js 14 and TypeScript.
Step 2: Configuring Cloudinary
Create a new file called lib/cloudinary.ts in your project:
import { v2 as cloudinary } from "cloudinary";
cloudinary.config({
cloud_name: process.env.NEXT_PUBLIC_CLOUDINARY_CLOUD_NAME,
api_key: process.env.CLOUDINARY_API_KEY,
api_secret: process.env.CLOUDINARY_API_SECRET,
});
export { cloudinary };
This configuration centralizes your Cloudinary setup, making it easy to import and use throughout your project.
Step 3: Setting Up Environment Variables
Create a .env.local file in your project root and add your Cloudinary credentials:
NEXT_PUBLIC_CLOUDINARY_CLOUD_NAME=your_cloud_name
CLOUDINARY_API_KEY=your_api_key
CLOUDINARY_API_SECRET=your_api_secret
Remember to add .env.local to your .gitignore file to prevent exposing sensitive information.
Creating an API Route for Image Upload
Now that we’ve set up Cloudinary, let’s create an API route to handle image uploads.
Step 1: Implementing the Upload Helper Function
Create a new file called lib/uploadHelper.ts:
import { cloudinary } from "./cloudinary";
import { UploadApiResponse, UploadApiErrorResponse } from "cloudinary";
type UploadResponse =
{ success: true; result?: UploadApiResponse } |
{ success: false; error: UploadApiErrorResponse };
export const uploadToCloudinary = (
fileUri: string,
fileName: string
): Promise<UploadResponse> => {
return new Promise((resolve, reject) => {
cloudinary.uploader
.upload(fileUri, {
invalidate: true,
resource_type: "auto",
filename_override: fileName,
folder: "next-cloudinary-uploads",
use_filename: true,
})
.then((result) => {
resolve({ success: true, result });
})
.catch((error) => {
reject({ success: false, error });
});
});
};
This helper function encapsulates the Cloudinary upload process, making it reusable across your application.
Step 2: Creating the API Route Handler
Create a new file app/api/upload/route.ts:
import { NextRequest, NextResponse } from 'next/server';
import { uploadToCloudinary } from '@/lib/uploadHelper';
export async function POST(req: NextRequest) {
if (req.method !== 'POST') {
return NextResponse.json({ message: 'Method Not Allowed' }, { status: 405 });
}
try {
const formData = await req.formData();
const file = formData.get('file') as File | null;
if (!file) {
return NextResponse.json({ message: 'No file provided' }, { status: 400 });
}
const bytes = await file.arrayBuffer();
const buffer = Buffer.from(bytes);
const fileUri = `data:${file.type};base64,${buffer.toString('base64')}`;
const fileName = `upload_${Date.now()}_${file.name}`;
const uploadResult = await uploadToCloudinary(fileUri, fileName);
if (uploadResult.success && uploadResult.result) {
return NextResponse.json({
message: 'Upload successful',
imageUrl: uploadResult.result.secure_url
}, { status: 200 });
} else {
return NextResponse.json({ message: 'Upload failed' }, { status: 500 });
}
} catch (error) {
console.error('Upload error:', error);
return NextResponse.json({ message: 'Internal Server Error' }, { status: 500 });
}
}
This API route handler processes the incoming file upload request and uses our helper function to upload the image to Cloudinary.
Implementing Client-Side File Upload
Now that our server-side code is ready, let’s implement the client-side file upload functionality.
Creating a File Upload Component
Create a new file components/FileUpload.tsx:
'use client';
import React, { useState } from 'react';
const FileUpload: React.FC = () => {
const [file, setFile] = useState<File | null>(null);
const [uploading, setUploading] = useState(false);
const [uploadedImageUrl, setUploadedImageUrl] = useState<string | null>(null);
const [error, setError] = useState<string | null>(null);
const handleFileChange = (e: React.ChangeEvent<HTMLInputElement>) => {
if (e.target.files) {
setFile(e.target.files[0]);
setError(null);
}
};
const handleUpload = async () => {
if (!file) {
setError('Please select a file to upload.');
return;
}
setUploading(true);
setError(null);
const formData = new FormData();
formData.append('file', file);
try {
const response = await fetch('/api/upload', {
method: 'POST',
body: formData,
});
const data = await response.json();
if (response.ok) {
setUploadedImageUrl(data.imageUrl);
} else {
setError(data.message || 'Upload failed');
}
} catch (error) {
console.error('Upload error:', error);
setError('An unexpected error occurred. Please try again.');
} finally {
setUploading(false);
}
};
return (
<div className="max-w-md mx-auto mt-10">
<input
type="file"
onChange={handleFileChange}
className="mb-4 p-2 w-full border rounded"
/>
<button
onClick={handleUpload}
disabled={!file || uploading}
className="w-full bg-blue-500 text-white p-2 rounded disabled:bg-gray-300"
>
{uploading ? 'Uploading...' : 'Upload'}
</button>
{error && <p className="text-red-500 mt-2">{error}</p>}
{uploadedImageUrl && (
<div className="mt-4">
<p className="mb-2">Uploaded Image:</p>
<img src={uploadedImageUrl} alt="Uploaded" className="max-w-full rounded shadow" />
</div>
)}
</div>
);
};
export default FileUpload;
This component provides a user interface for selecting and uploading files, and displays the uploaded image once the process is complete.
Integrating the File Upload Component
Now, let’s use our FileUpload component in a page. Update your app/page.tsx:
import FileUpload from '@/components/FileUpload';
export default function Home() {
return (
<main className="flex min-h-screen flex-col items-center justify-between p-24">
<h1 className="text-4xl font-bold mb-8">Cloudinary Image Upload</h1>
<FileUpload />
</main>
);
}
Testing Your Implementation
- Start your Next.js development server:
- Open your browser and navigate to http://localhost:3000.
- You should see the file upload interface. Select an image and click the “Upload” button.
- If everything is set up correctly, you’ll see the uploaded image displayed on the page, and it will be stored in your Cloudinary account.
Conclusion
Congratulations! You’ve successfully implemented a complete Cloudinary image upload functionality in your Next.js application. This powerful integration allows you to effortlessly manage and serve images, improves your app’s performance and user experience.
By following this guide, you’ve learned how to:
- Set up a Next.js project with TypeScript
- Configure Cloudinary in a Next.js environment
- Create a secure API route for handling image uploads
- Implement a reusable client-side file upload component
- Handle errors and provide user feedback during the upload process
Remember to implement proper authentication for your API routes in a production environment, and consider optimizing your uploads further by compressing images before sending them to Cloudinary.