How to rewrite URL in Next.jS ?
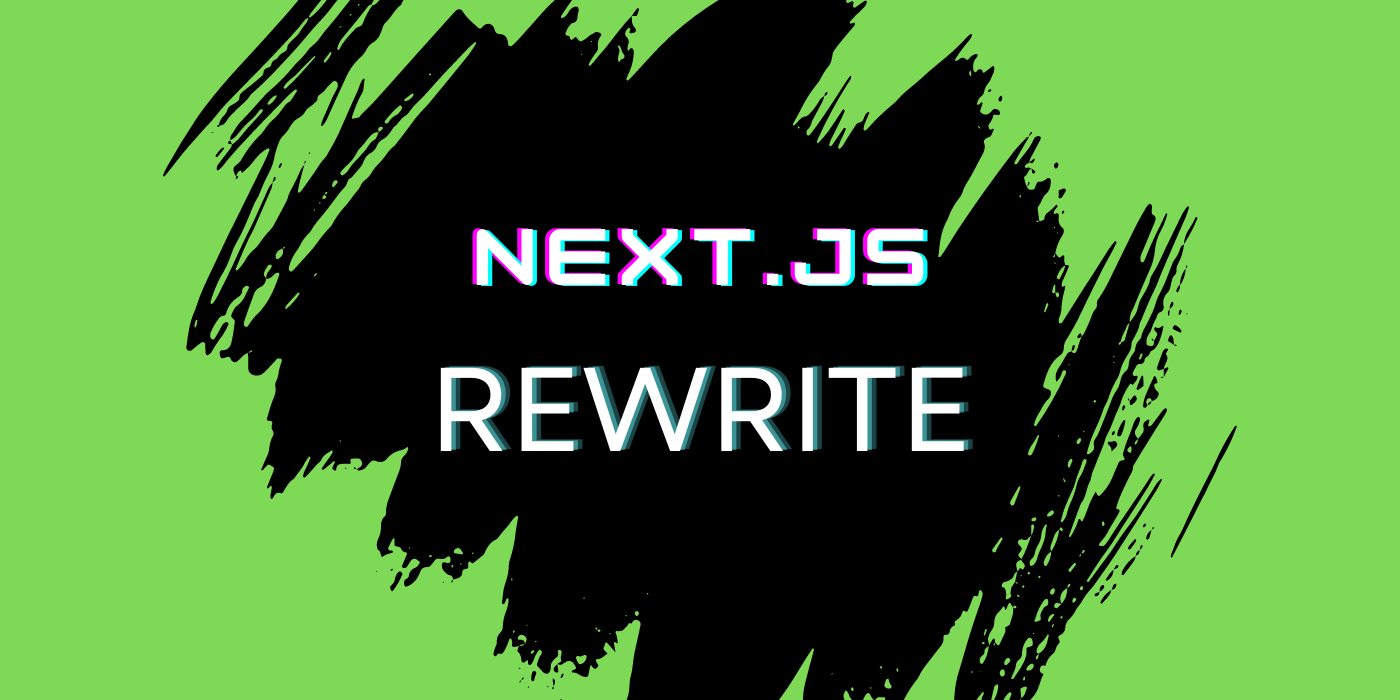
Introduction
Next.js is the popular React framework for building server-rendered and static websites, offers a powerful feature called Rewrites. Rewrites allow you to map on incoming requests to different destinations, allows server-side rendering (SSR), static site generation (SSG), and dynamic imports. In this complete guide, we’ll explore Next.js Rewrites in depth, covering everything from setup to advanced techniques and real-world use cases.
Understanding Rewrites
A Next.js Rewrite is a configuration that maps an incoming request path to a different destination. This destination can be another path within your Next.js application or an external URL. Rewrites are different from redirects in that they don’t change the browser’s URL. instead, they handle the request on the server-side and serve the appropriate content.
Setting Up Rewrites In Next.JS App
To configure Rewrites in Next.js, you’ll need to create or modify the next.config.js file in the root of your project. Inside this file, you can define an array of rewrites under the rewrites key. Each rewrite object should have a source and destination property.
// next.config.js
module.exports = {
rewrites: async () => [
{
source: '/old-path',
destination: '/new-path',
},
],
}
In this example, any request to /old-path will be rewritten to /new-path within your Next.js application.
Basic Rewrite Examples
1. Simple Path Rewrite
{
source: '/blog/:slug',
destination: '/articles/:slug',
}
This rewrite maps requests from /blog/my-post to /articles/my-post.
2. Rewriting with Dynamic Parameters
{
source: '/products/:category/:id',
destination: '/:category/:id',
}
This rewrite maps requests like /products/electronics/123 to /electronics/123, preserving the dynamic parameters.
3. Rewriting to an External URL
{
source: '/external',
destination: 'https://example.com',
}
This rewrite maps requests to /external to the external URL https://example.com.
Advanced Rewrite Techniques
1. Pattern Matching with Regular Expressions
{
source: '/posts/(\\d{4})/(\\d{2})/(\\d{2})/:slug',
destination: '/archive/:slug',
}
This rewrite uses a regular expression to match requests like /posts/2023/05/18/my-post and rewrites them to /archive/my-post.
2. Combining Rewrites with API Routes
{
source: '/api/:path*',
destination: '/api-handler/:path*',
}
This rewrite maps all incoming API requests to a custom API handler file.
3. Rewrite Conditions and Source Filtering
{
source: '/secret/:path*',
destination: '/404',
has: [
{
type: 'cookie',
key: 'authed',
value: 'false',
},
],
}
This rewrite redirects requests to /secret/* to a 404 page if the user doesn’t have the authed cookie set to true.
Handling Specific Use Cases
1. Rewriting for Static Site Generation (SSG)
{
source: '/posts/:slug',
destination: '/blog/:slug',
}
This rewrite allows you to use the /posts path for statically generated blog posts while serving the content from the /blog directory.
2. Rewriting for Server-Side Rendering (SSR)
{
source: '/ssr-page',
destination: '/server-rendered-page',
}
This rewrite maps requests for /ssr-page to a server-rendered page at /server-rendered-page.
3. Rewriting for Incremental Static Regeneration (ISR)
{
source: '/isr-page/:slug',
destination: '/cached-page/:slug',
}
This rewrite helps serve cached pages for ISR while handling requests to the /isr-page path.
Best Practices and Considerations
While Rewrites are powerful, it’s good to follow best practices and consider potential implications
- Performance Implications: Rewrites can introduce additional server-side processing, so it’s important to optimize your rewrites and avoid unnecessary operations.
- Handling Conflicts and Overlapping Routes: If you have multiple rewrites that match the same path, Next.js will use the first matching rule. Be mindful of potential conflicts and prioritize your rewrites accordingly.
- Security Considerations: Rewrites can be vulnerable to open redirect attacks if not configured properly. Validate user input and avoid blindly redirecting to external URLs.
- Testing and Debugging: Test your rewrites thoroughly and use Next.js’s built-in debugging tools to identify and fix issues.
Rewrites vs. Other Next.jS Routing Features
Next.js offers several routing features, and it’s important to understand when to use Rewrites versus other options:
- Dynamic Routing: Next.js Dynamic Routing allows you to define pages with dynamic segments, like /posts/[slug]. Use Dynamic Routing when you want to render different pages based on the URL path.
- Redirects: Redirects are similar to Rewrites but change the browser’s URL. Use Redirects when you want to permanently or temporarily redirect users to a different URL.
- Rewrites: Use Rewrites when you want to map incoming requests to different destinations without changing the browser’s URL. Rewrites are particularly useful for SSR, SSG, and dynamic imports.
Conclusion
Next.js Rewrites are a powerful feature that allows advanced routing capabilities, server-side rendering, static site generation, and dynamic imports. By understanding the basics, advanced techniques, best practices, and real-world use cases, you can leverage Rewrites to build robust and scalable Next.js applications.
If you’re new to Next.js or want to learn more about Rewrites and other routing features, check out the official Next.js documentation and explore the vibrant Next.js community for additional resources and examples.
FAQs
What is the difference between Rewrites and Redirects in Next.js?
Rewrites and Redirects are both routing features in Next.js, but they have a key difference. Rewrites map an incoming request to a different destination without changing the browser’s URL. In contrast, Redirects change the browser’s URL and send the user to a new location.
Can I use regular expressions in Next.js Rewrites?
Yes, Next.js Rewrites support the use of regular expressions for pattern matching in the source property. This allows you to create more advanced and flexible rewrite rules.
Can I conditionally apply Rewrites based on specific criteria?
Yes, Next.js Rewrites support conditional logic using the has property. You can define conditions based on headers, cookies, query parameters, or other factors to selectively apply Rewrite rules.