How to Implement API Rate Limiting in a NodeJS Express Application
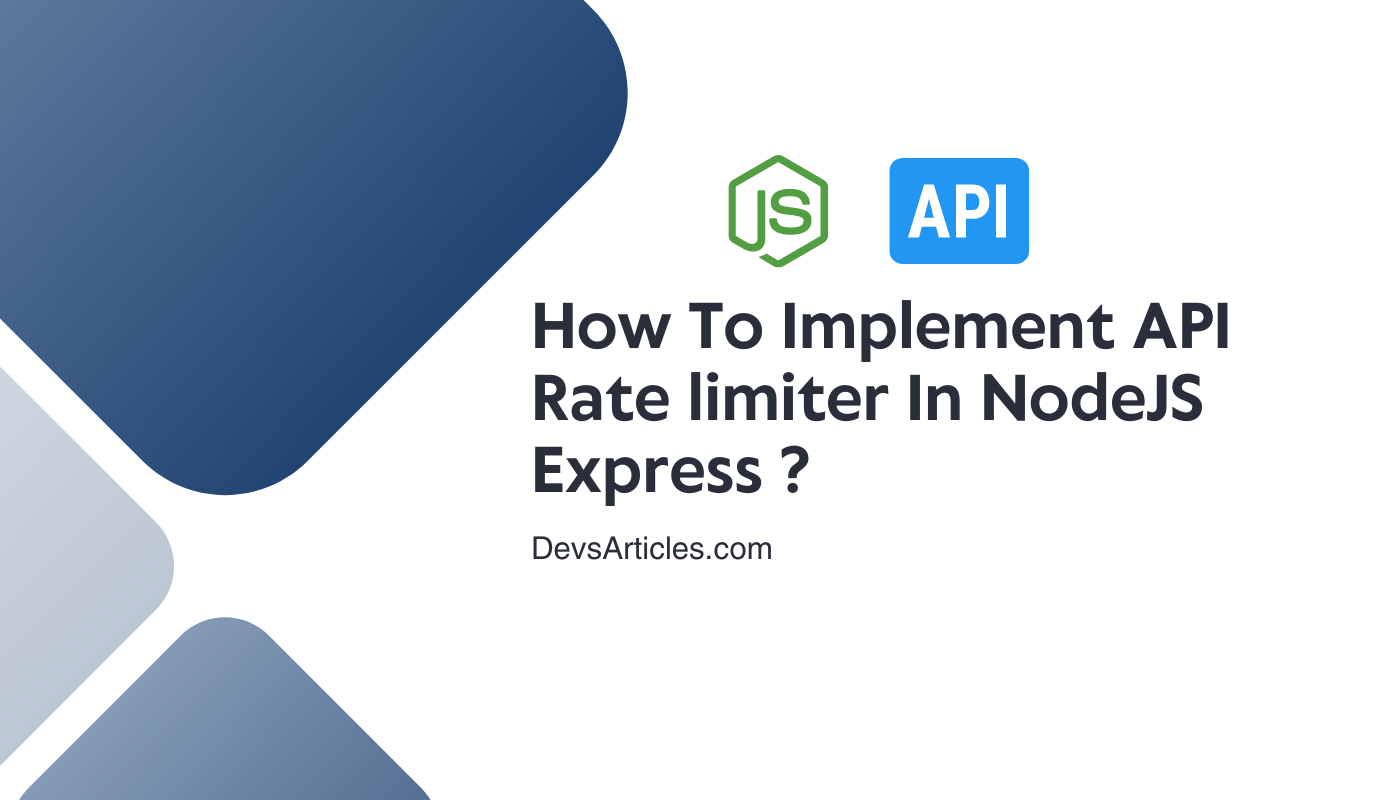
In the dynamic world of web development, rate Limiting stands as a critical pillar for maintaining stability and fairness within applications and websites. Basically, it functions as a regulatory mechanism, dictating the pace at which users can request data or services from your platform. By imposing this restriction, rate limiting shields your server from possible overload, safeguarding it against crashes or slowdowns that could result from an excessive inflow of requests.
Further very protection against server strain and rate limiting also serves as a challenging defense against malicious activities such as DDoS (Distributed Denial of Service) attacks. By restraining the rate at which requests can be made, it effectively raises the barrier against automated bots or scripts attempting to flood your server with malicious intent.
Table of Contents
Why Use Rate Limiting in NodeJS Express ?
In NodeJS Express applications, integrating rate limiting functionality is important for several effective reasons. Let’s dive into why implementing rate limiting is crucial in this context:
Protection Against Brute Force Attacks
Brute force attacks involve repeated, rapid login attempts with various username-password combinations to gain unauthorized access. By using rate limiting, NodeJS Express applications can reduce the impact of such attacks. Limiting the number of login attempts within a specific timeframe helps prevent brute force attempts, making it significantly more challenging for malicious actors to compromise user accounts.
Prevention of API Abuse
In a NodeJS Express application with exposed APIs, rate limiting acts as a shield against abuse. Without rate limiting, malicious users or automated bots could attack the server with an excessive volume of requests, leading to resource insufficiency, service degradation, or even downtime. By imposing restrictions on the frequency of API calls from individual clients, rate limiting secures fair access to server resources while safeguarding against abuse.
Optimization of Server Resources
Resource optimization is another clear cause for incorporating rate limiting into NodeJS Express applications. By controlling the rate of incoming requests, rate limiting helps distribute server resources more efficiently, preventing any single client from dominating available bandwidth, CPU, or memory. This promotes a fair allocation of resources, improving overall system performance and scalability.
how to implement a rate limiter in the Express app?
Implementing rate limiting in a NodeJS Express application is straightforward, thanks to popular rate-limiting middleware like express-rate-limit or by creating custom middleware. Let’s walk through the steps:
Install express-rate-limit
Start by installing the express-rate-limit package using npm or yarn:
npm install express-rate-limit
Let’s Configure Express-rate-limit
In your Express server file, typically named server.js
or index.js
, set up your Express server, and configure the rate limiting middleware provided by express-rate-limit. This middleware will restrict the number of requests allowed from each IP address within a specified time window.
const express = require('express');
const rateLimit = require("express-rate-limit");
const app = express();
const PORT = process.env.PORT || 3000;
// Define rate limit options
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
// Apply rate limiting middleware to all routes
app.use(limiter);
// Define routes
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this code block, we import express and express-rate-limit, set up an Express app, define rate limiting options, apply the rate limiting middleware to all routes, define some routes (in this case, just a root route), and start the server on a specified port.
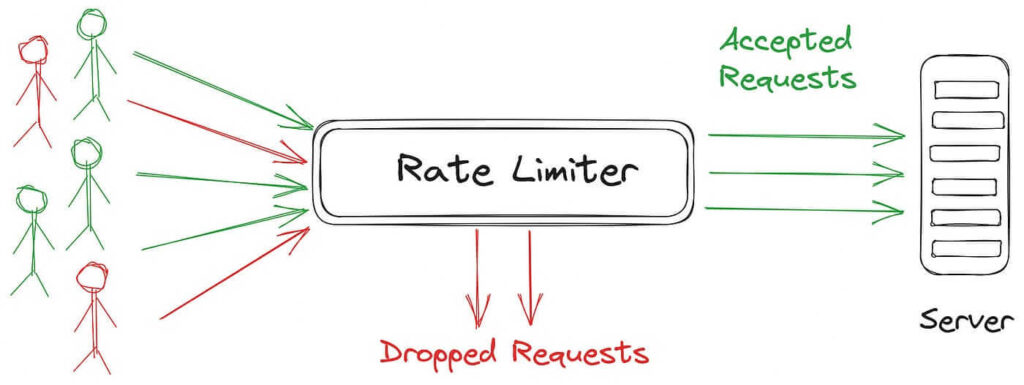
Express Rate Limit with Custom middleware
If you prefer more customization or control over the rate limiting logic, you can create custom middleware. This allows you to make the rate limiting behavior according to your specific requirements.
// customRateLimit.js
function customRateLimit(req, res, next) {
// Logic to track and limit requests
// Example: Store request counts in an object with IP addresses as keys
const ip = req.ip;
requestCounts[ip] = (requestCounts[ip] || 0) + 1;
// Check if request count exceeds the limit
if (requestCounts[ip] > maxRequests) {
return res.status(429).send("Too many requests, please try again later.");
}
next();
}
module.exports = customRateLimit;
In this custom middleware, we define the logic for rate limiting. We track the number of requests from each IP address and compare it against a predefined maximum limit. If the limit is exceeded, we return a “429 Too Many Requests” status code with a corresponding message.
use this middleware in your main file
// server.js
const express = require('express');
const customRateLimit = require('./customRateLimit');
const app = express();
const PORT = process.env.PORT || 3000;
// Apply custom rate limiting middleware
app.use(customRateLimit);
// Define routes
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Let’s Have a Short Discussion of Configuration options
- Limiting Based on IP Address or User Agent:
You can choose to set limits based on the IP address of the client making the request or the user agent (browser or device) used to access your application. This helps differentiate between different clients and restricts their request rates accordingly. - Limiting by Route:
You have the flexibility to apply rate limits selectively to specific routes or endpoints within your application. This allows you to prioritize critical routes or protect sensitive endpoints from excessive traffic. - Adjusting the Rate Limit Window:
The rate limit window specifies the duration over which requests are counted. You can adjust this window to suit your application’s requirements. For example, you might set a window of 15 minutes, meaning the rate limit applies to the number of requests made within that timeframe. - Setting Maximum Requests:
Define the maximum number of requests allowed within the specified rate limit window. This controls how many requests a client can make before being temporarily restricted. - Handling Different HTTP Status Codes:
Configure how your application responds when the rate limit is exceeded. Commonly used status codes include:- 429 Too Many Requests Indicates that the client has exceeded the rate limit and should try again later.
- 403 Forbidden Indicates that the client is not allowed to access the resource due to exceeding the rate limit.
- Excluding Certain Requests:
Optionally, you can exclude certain requests from being subject to rate limiting. For example, you may want to exempt requests from internal services or administrative users.
Configuration Option | Description |
windowMs | The time window in milliseconds during which requests are counted for rate limiting. |
max | The maximum number of requests allowed within the specified time window. |
message | Custom message to be sent in the response when the rate limit is exceeded. |
statusCode | HTTP status code to be sent in the response when the rate limit is exceeded. |
skip | A function that determines whether to skip rate limiting for specific requests. |
keyGenerator | A function that generates a key for grouping requests, useful for limiting based on specific criteria (e.g., IP, User Agent). |
handler | A function to handle requests that exceed the rate limit, allowing for custom error responses or additional processing. |
headers | Boolean value indicating whether to include rate limit headers in the response (X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset). |
skipFailedRequests | Boolean value indicating whether to skip rate limiting for failed requests (responses with status codes 4xx or 5xx). |
Let’s explore some common use cases of rate limiter
- API Development:
In API development, rate limiting helps secure fair usage and protects against abuse or misuse. For example, a public API might implement rate limiting to restrict the number of requests made by each client, preventing them from overpowering the server. This confirms that the API remains responsive for all users and maintains optimal performance. - Authentication Systems:
Rate limiting can be applied to authentication systems to prevent brute force attacks. For instance, when users attempt to log in, rate limiting can restrict the number of login attempts from a single IP address within a specific time frame. This helps thwart malicious players attempting to guess passwords by repeatedly trying different combinations. - Content Delivery Networks (CDNs):
CDNs often use rate limiting to manage the delivery of content to end-users. By limiting the number of requests from each client, CDNs can prevent excessive bandwidth consumption and assure fair distribution of resources. This is particularly important during traffic spikes or DDoS attacks, where rate limiting helps mitigate the impact on CDN infrastructure. - Data Analysis and Reporting:
In applications that involve data analysis or reporting functionalities, rate limiting can be used to control the frequency of data return or processing requests. For instance, a dashboard application that fetches real-time data from external APIs may implement rate limiting to prevent excessive querying and optimize resource utilization. - Payment Processing Systems:
Rate limiting plays a important role in payment processing systems to prevent fraudulent activities such as card testing attacks. By limiting the number of payment authorization attempts from a single source, rate limiting helps detect and block suspicious transactions, reducing the risk of financial losses and providing the security of payment processing infrastructure. - Subscription-Based Services:
Subscription-based services often utilize rate limiting to implement usage quotas for different subscription tiers. For example, a software-as-a-service (SaaS) platform may impose rate limits on API usage or feature access based on the subscription plan of each user. This assures that users stay within their allocated limits and encourages them to upgrade to higher-tier plans for increased usage.
Conclusion
Rate limiting is necessary for maintaining stability and fairness in applications and websites. By regulating incoming requests, it safeguards servers from overload and provides consistent performance.In NodeJS Express applications, rate limiting serves multiple purposes. Firstly, it protects against threats like brute force attacks by limiting login attempts within set timeframes. Additionally, it prevents API abuse by restricting request frequencies, preserving server resources.
Moreover, rate limiting acts as a defense against malicious activities like DDoS attacks, hindering automated bots from overwhelming servers. Its implementation, whether through middleware or custom solutions, is straightforward.In essence, rate limiting is key in modern web development, providing reliability and efficiency while reduce risks. Its integration into NodeJS Express applications is important for upholding these principles and delivering outstanding user experiences.
What is rate limiting, and why is it important in web development?
Rate limiting is a mechanism used to control the rate at which clients can make requests to a server. It is important in web development to prevent server overload, maintain stability, and ensure fair usage of resources.
How can I implement rate limiter in my NodeJS Express application?
You can implement rate limiter using middleware such as express-rate-limit or by creating custom middleware. These allow you to configure options such as limiting based on IP address, adjusting rate limit windows, and customizing error responses.