Node jS connect to MongoDB using mongoose
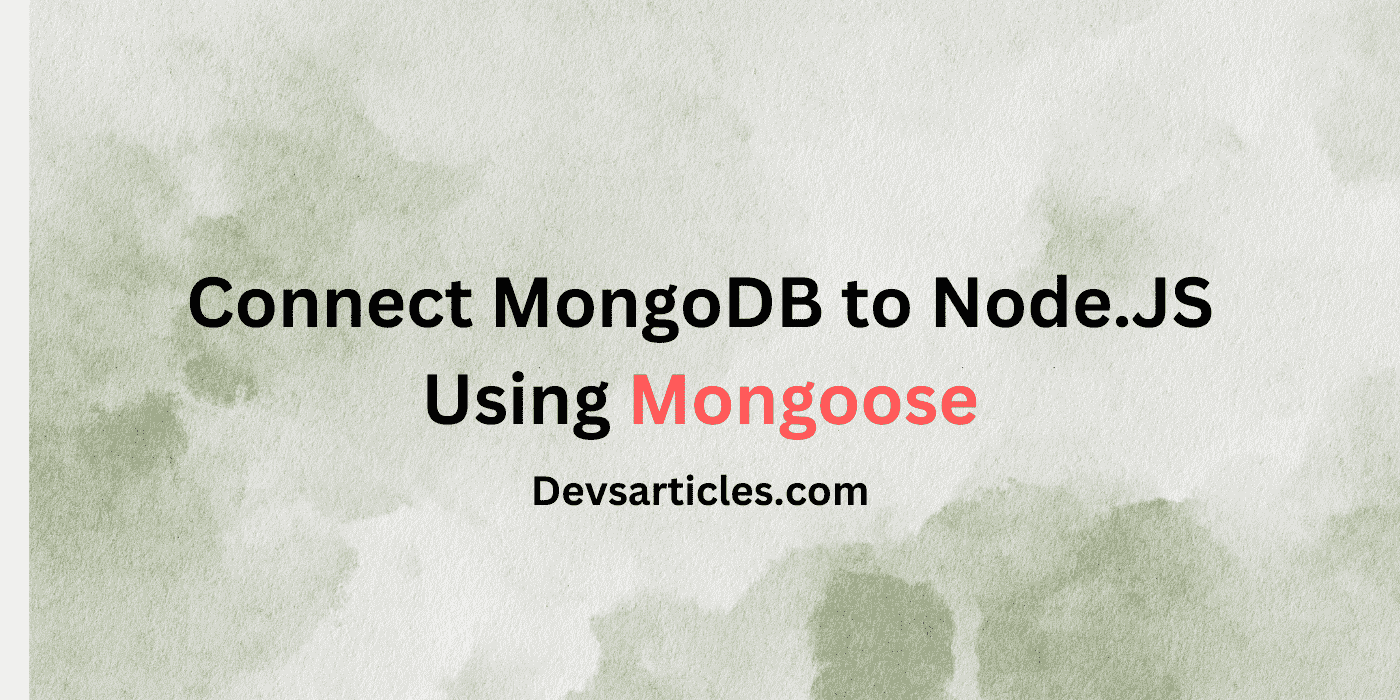
Introductions
Hey there! Are you ready to learn how to connect your Node.js application to MongoDB using Mongoose? Great! Let’s break it down in simple terms.
What are Node.js, MongoDB, and Mongoose?
First, let’s quickly go over what these technologies are:
- Node.js: It’s a platform that lets you run JavaScript on your computer, not just in a web browser.
- MongoDB: This is a popular database that stores data in a format similar to JSON.
- Mongoose: Think of it as a helper tool that makes it easier for your Node.js app to talk to MongoDB.
Why is connecting to a database important?
Imagine you’re building a cool web app. You’ll need a place to store user information, posts, or any other data your app uses. That’s where connecting to a database comes in handy!
By connecting Node.js to MongoDB:
- Your app can save data for later use
- You can fetch data to show to users
- Your data stays safe and organized
In this guide, we’ll focus on how to set up this connection. It’s like building a bridge between your Node.js app and MongoDB, and Mongoose is going to be our trusty construction tool.
Table of Contents
Setting Up the Environment
Before we can connect Node.js to MongoDB using Mongoose, we need to set up our work environment. Don’t worry, it’s easier than it sounds! Let’s go through this step-by-step.
Please make sure you have node.js installed in your local machine , and mongoDB installed on local machine or you have created database in mongoDB atlas.
Creating a New Node.js Project
First Open your terminal or command prompt. than navigate to where you want to create your project. Create a new folder for your project:
mkdir my-mongodb-project
cd my-mongodb-project
Initialize a new Node.js project:
npm init -y
This creates a package.json file in your project folder.
Installing Mongoose
Now that we have our Node.js project set up, it’s time to add Mongoose to the mix. Mongoose is like a friendly helper that makes talking to MongoDB much easier. Let’s get it installed!
Using npm to Install Mongoose
npm (Node Package Manager) comes bundled with Node.js, and it’s super helpful for installing packages like Mongoose. Here’s how to use it:
Open your terminal or command prompt. Make sure you’re in your project folder (my-mongodb-project).Type the following command and hit Enter:
npm install mongoose
This command tells npm to download and install Mongoose in your project.
Establishing a Connection
Now that we have Mongoose installed, it’s time for the exciting part – connecting our Node.js application to MongoDB! Let’s break this down into easy steps.
Importing Mongoose in Your Node.js Application
First, we need to tell our application to use Mongoose. Create a new file called app.js in your project folder and add this line at the top:
const mongoose = require('mongoose');
This line imports Mongoose into our application, making all its features available to us.
Writing the Connection String
To connect to MongoDB, we need a connection string. Think of it as the address of your database. Here’s what it looks like:
const connectionString = 'mongodb://localhost:27017/myDatabase';
Let’s break down this connection string:
mongodb://
is the protocollocalhost
is the server where MongoDB is running (in this case, on your own computer)27017
is the default port for MongoDBmyDatabase
is the name of your database (you can change this to whatever you like)
Connecting to MongoDB using Mongoose.connect()
Now, let’s use Mongoose to connect to our database:
mongoose.connect(connectionString, {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => console.log('Connected to MongoDB!'))
.catch(err => console.error('Could not connect to MongoDB...', err));
This code does a few things:
- It tries to connect to MongoDB using our connection string.
- The options useNewUrlParser and useUnifiedTopology are recommended by Mongoose for using the latest MongoDB driver features.
- If the connection is successful, it logs a success message.
- If there’s an error, it logs the error message.
Handling Connection Events
Mongoose also lets us handle connection events more specifically:
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', function() {
console.log('We are connected to MongoDB!');
});
This code sets up listeners:
- If there’s an error, it will be logged to the console.
- When the connection is successfully opened, it logs a message.
And that’s it! You’ve now set up a basic connection between your Node.js application and MongoDB using Mongoose.
Connection Options
Now that we’ve established a basic connection, let’s explore some useful options that can make our MongoDB connection more robust and efficient.
Common Connection Options
Mongoose allows us to customize our connection with various options. Here are some commonly used ones:
const options = {
useNewUrlParser: true,
useUnifiedTopology: true,
serverSelectionTimeoutMS: 5000,
autoIndex: false, // Don't build indexes
maxPoolSize: 10, // Maintain up to 10 socket connections
socketTimeoutMS: 45000, // Close sockets after 45 seconds of inactivity
family: 4 // Use IPv4, skip trying IPv6
};
mongoose.connect(connectionString, options);
Let’s break down these options:
- useNewUrlParser and useUnifiedTopology: These are typically recommended for using the new MongoDB driver features.
- serverSelectionTimeoutMS: This sets how long Mongoose will try to connect before giving up.
- autoIndex: Setting this to false can speed up initial connection time.
- maxPoolSize: This controls how many connections Mongoose will make to MongoDB.
- socketTimeoutMS: This sets how long Mongoose will wait before closing inactive connections.
- family: This specifies whether to use IPv4 or IPv6.
Configuring Connection Pooling
Connection pooling helps manage multiple database connections efficiently. Here’s how you can configure it:
const options = {
maxPoolSize: 10, // Maximum number of sockets to keep open
minPoolSize: 5, // Minimum number of sockets to keep open
socketTimeoutMS: 30000, // Close sockets after 30 seconds of inactivity
};
mongoose.connect(connectionString, options);
This setup keeps between 5 and 10 connections open, closing inactive ones after 30 seconds.
Best Practices for Connection Management
Now that we know how to connect to MongoDB and configure our connection, let’s talk about some best practices. These tips will help keep your database connection secure and well-managed.
Using Environment Variables for Connection Strings
It’s not a good idea to put your database connection string directly in your code, especially if you’re sharing your code or using version control. Instead, use environment variables. Here’s how:
Create a file named .env
in your project root:
MONGODB_URI=mongodb://localhost:27017/myDatabase
Install the dotenv package:
npm install dotenv
In your app.js
, use it like this:
require('dotenv').config();
const mongoose = require('mongoose');
mongoose.connect(process.env.MONGODB_URI);
Conclusion
Congratulations! You’ve just learned how to connect your Node.js application to MongoDB using Mongoose. Let’s recap what we’ve covered:
- We started by understanding what Node.js, MongoDB, and Mongoose are, and why connecting to a database is crucial for your web applications.
- We set up our development environment, creating a new Node.js project and installing Mongoose.
- We learned how to establish a connection to MongoDB, including writing the connection string and handling connection events.
- We explored various connection options that can help optimize our database interactions.
- Finally, we discussed best practices for connection management, such as using environment variables for sensitive information.
By following these steps, you’ve built a solid foundation for your Node.js and MongoDB projects. Remember, the connection is just the beginning with this setup, you’re now ready to start creating, reading, updating, and deleting data in your MongoDB database using Mongoose.
As you continue to develop your applications, keep these key points in mind:
- Always secure your connection strings using environment variables.
- Configure your connection options based on your specific needs.
- Handle connection errors gracefully to make your application more robust.
FAQs
What’s the difference between MongoDB and Mongoose?
MongoDB is the database itself, while Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. Mongoose provides a straightforward, schema-based solution to model your application data.
Is it safe to put my MongoDB connection string in my code?
No, it’s not recommended. Always use environment variables to store sensitive information like connection strings, especially if you’re pushing your code to a public repository.
How do I close a Mongoose connection?
You can close a connection using mongoose.connection.close()
. It’s a good practice to close your connection when your application is shutting down.