Connect MongoDB with NextJS Complete guide with mongoose
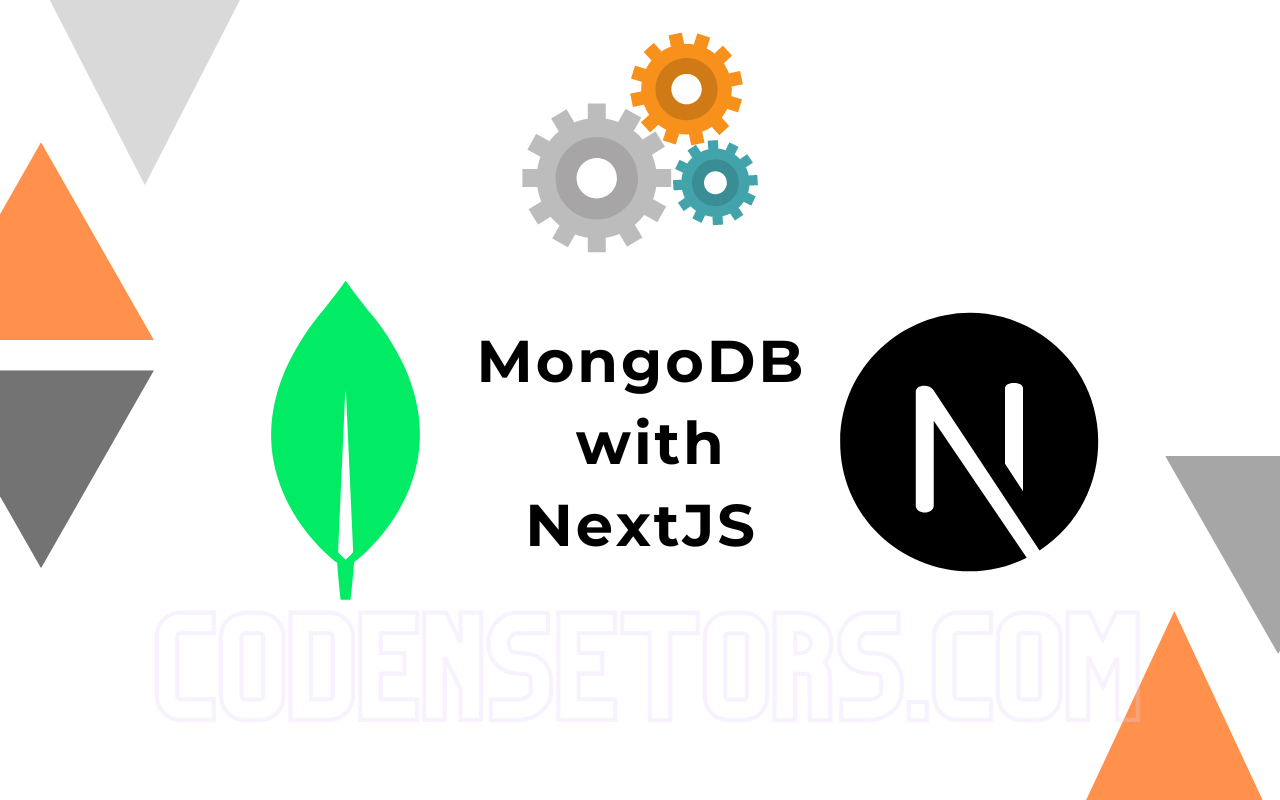
In the world of modern web development, MongoDB and NextJS are a powerful combination for building scalable and robust applications. MongoDB, a NoSQL database, provides flexibility, while NextJS offers a complete framework for building React applications with server-side rendering and routing capabilities. In this guide, we will go through the process of connecting MongoDB with NextJS, using the default MongoDB driver, and additional libraries like Mongoose. By following these steps, you will be able to easily integrate MongoDB into your NextJS projects, allowing for efficient data storage and retrieval.
Table of Contents
Basic Steps For Connecting mongoDB with NextJS
Setting up MongoDB
The MongoDB Atlas platform simplifies NextJS database connections by enabling users to create and manage MongoDB databases on the cloud through a user-friendly graphical interface. If you don’t have account than sign up and create new database
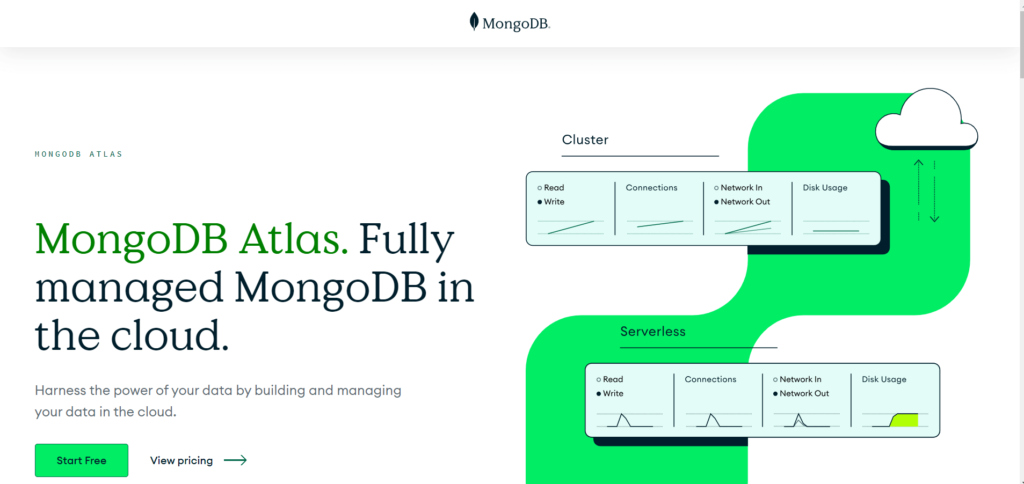
Or, Make sure you have MongoDB installed on your system. If you haven’t installed it yet, you can download it from the official website (https://www.mongodb.com/). Once you have installed it, you can start the MongoDB server by running the appropriate command for your operating system.
Explore a complete guide on what is MongoDB and MongoDB atlas?
Create new NextJS Project
Let’s create a new NextJS project. Open your terminal and run the following commands:
npx create-next-app my-next-app
cd my-next-app
This will create a new NextJS project and navigate you into the project directory.
Install Dependency
Now, we need to install the MongoDB NodeJS driver, which will enable us to interact with MongoDB from our NextJS application. Open your terminal and type the following command:
npm install mongodb
Connect MongoDB with NextJS
Now that we have our NextJS project set up and the MongoDB driver installed, let’s make a connection to our MongoDB database. Create a new file named “mongodb.js” in the “lib” directory of your NextJS project. In this file, add the following code:
import { MongoClient } from 'mongodb';
const uri = 'mongodb://localhost:27017'; // Replace with your MongoDB connection string
const client = new MongoClient(uri);
let cachedDb = null;
export async function connectToDatabase() {
if (cachedDb) {
return cachedDb;
}
if (!client.isConnected()) {
await client.connect();
}
const db = client.db('my-database'); // Replace 'my-database' with your database name
cachedDb = db;
return db;
}
Replace mongodb://localhost:27017
with your MongoDB local or MongoDB cloud atlas connection string, and my-database
with the name of your MongoDB database.
Now, we can establish a connection to our MongoDB database from any file in our NextJS project by using the connectToDatabase function.
Querying Data from MongoDB
With Successful connection of MongoDB with NextJS, let’s query some data from a MongoDB collection. For demonstration purposes, let’s think we have a collection of named users in our MongoDB database, containing documents with fields “name” and “email”.
In your NextJS page or API route file, import the connectToDatabase function and query data from the user’s collection:
import { connectToDatabase } from '../lib/mongodb';
export default async function handler(req, res) {
const db = await connectToDatabase();
const users = await db.collection('users').find({}).toArray();
res.json(users);
}
This example shows how to retrieve all documents from the users collection and return them as JSON.
Connect MongoDB with NextJS Using Mongoose
In this section, we’ll explore an alternative method to integrating MongoDB with NextJS using Mongoose, a popular MongoDB object modeling library for NodeJS.
Mongoose simplifies interactions with MongoDB by providing a schema-based solution and utilities for data manipulation and validation.
Installing Mongoose
To use Mongoose in a NextJS project, install it as a dependency using the command in the terminal:
npm install mongoose
Create MongoDB Schema
When using Mongoose, we define schemas that represent the structure of documents within MongoDB collections. For example, to create a User schema:
// models/User.js
import mongoose from 'mongoose';
const userSchema = new mongoose.Schema({
name: String,
email: String,
});
export default mongoose.models.User || mongoose.model('User', userSchema);
Connecting to MongoDB with Mongoose In NextJS
Mongoose connects to MongoDB using a URI in a separate file, such as mongoose.js:
// lib/mongoose.js
import mongoose from 'mongoose';
const uri = 'mongodb://localhost:27017/my-database'; // Replace with your MongoDB connection string and database name
mongoose.connect(uri, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
export default mongoose.connection;
Querying Data with Mongoose
Once the connection is established, we can perform CRUD (Create, Read, Update, Delete) operations using Mongoose. For example, to fetch all users:
// pages/api/users.js
import db from '../../lib/mongoose';
import User from '../../models/User';
export default async function handler(req, res) {
await db.once('open', async () => {
const users = await User.find({});
res.json(users);
});
}
Conclusion
We’ve explored the process of connecting MongoDB with NextJS using the default MongoDB driver. By following these steps, you can integrate MongoDB into your NextJS projects, allowing efficient data storage and retrieval. With MongoDB’s flexibility and NextJS powerful capabilities, you can build robust and scalable web applications with ease.
Mongoose simplifies MongoDB integration with NextJS by providing schema-based modeling and utilities for database operations. While it outlines away some of the complexities of direct MongoDB interactions, it offers a structured approach for managing data within NextJS applications. Developers can choose between the default MongoDB driver or Mongoose based on project requirements and understanding of the respective guidelines.
Links For Reference From Official Documents
MongoDB, MongoDB Atlas, Mongoose
FAQs
Why integrate MongoDB with NextJS?
Integrating MongoDB with NextJS allows developers to use the flexibility of a NoSQL database for storing and retrieving data in their web applications. This combination offers scalability and performance benefits, allowing for the creation of robust and efficient applications.
Is MongoDB Atlas necessary for connecting MongoDB with NextJS?
While MongoDB Atlas simplifies database management by offering a cloud-based solution, it is not necessary to connect MongoDB with NextJS. Developers can use a locally installed MongoDB instance or any other MongoDB hosting provider for integration with NextJS.