How to use Multithreading in Node.js: A Comprehensive Guide
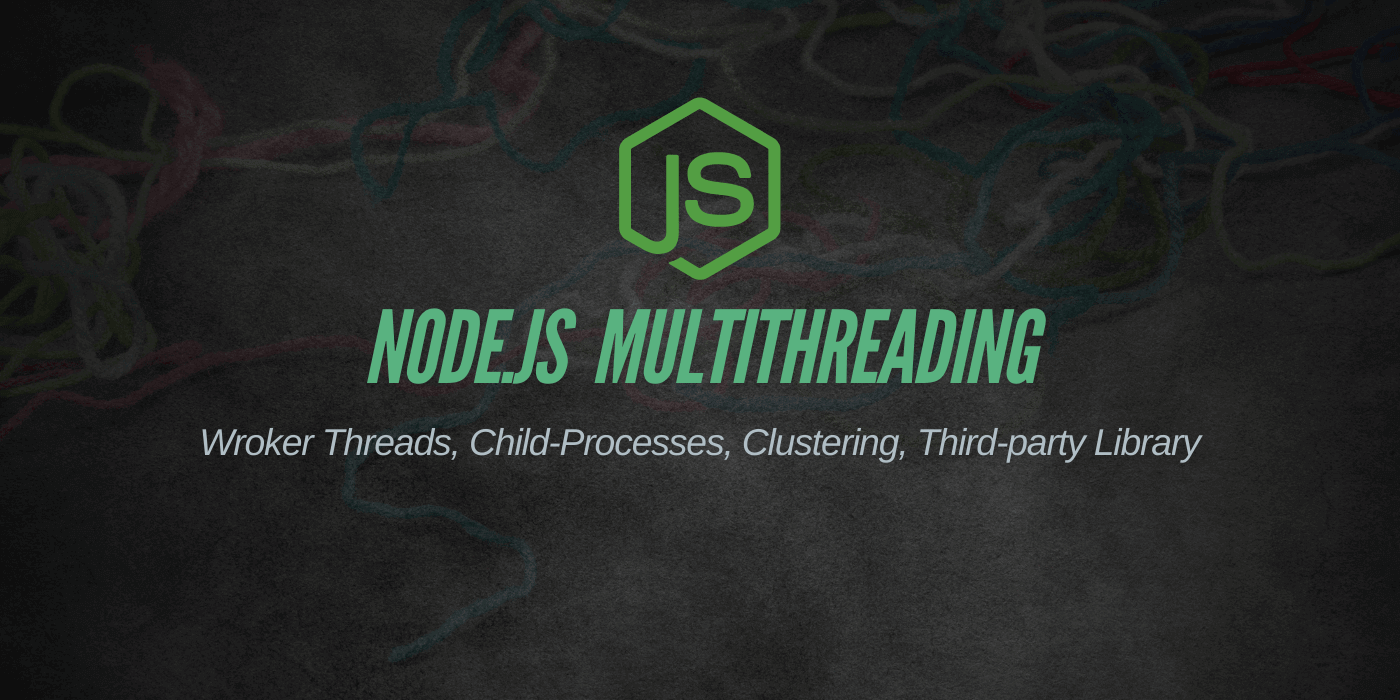
Introduction to Multithreading
Node.js has revolutionized server-side programming with its event driven Architecture, non-blocking I/O model. However, as applications grow more complex, developers often faces performance bottlenecks due to Node’s single-threaded nature. This is where multithreading comes into play, offering a powerful solution to improves your Node.js applications. This is not only for nodejs js specific , you can multithreading in javascript also. Node.js pre-built module is much more helpfull
What is Multithreading?
Multithreading is a programming concept that allows multiple threads (smaller units of a process) to run concurrently. Think of it as having multiple workers tackling different tasks simultaneously within the same application. multithreading concepts is all depends on processes and threads.
Why is Multithreading Important in Node.js?
While Node.js excels at handling asynchronous operations, CPU-intensive tasks can still block the main thread, potentially slowing down your entire application. Multithreading allows you to offload these heavy computations to separate threads, keeping your main application responsive.
Overview of Node.js Single-threaded Nature
By default, Node.js operates on a single thread, utilizing an event loop to manage asynchronous operations. This model is efficient for I/O-bound tasks but can struggle with CPU-bound operations. Here’s a simple example of how Node.js typically handles operations:
console.log('Start');
setTimeout(() => {
console.log('Timer 1 finished');
}, 0);
console.log('End');
// Output:
// Start
// End
// Timer 1 finished
In this example, even though the timer is set to 0 milliseconds, it still runs after the main thread has finished, demonstrating Node’s non-blocking nature.
Understanding this single-threaded model is important as we explore multithreading options to overcome its limitations in certain scenarios.
The Need for Multithreading in Node.js
As powerful as Node.js is, its single-threaded nature can become a bottleneck in certain scenarios. Let’s explore why multithreading becomes necessary and how it can benefit your applications.
Limitations of Single-threaded Execution
Node.js excels at handling I/O operations efficiently, but it can struggle with CPU-intensive tasks. Here’s why:
- Blocking operations: CPU-bound tasks can block the event loop, preventing other operations from executing.
- Underutilization of resources: On multi-core systems, a single-threaded application can’t fully utilize all available CPU cores.
- Scalability issues: As the workload increases, a single thread may not be able to keep up with the demand.
Here’s a simple example to illustrate a blocking operation:
function blockingOperation() {
const start = Date.now();
while (Date.now() - start < 5000) {
// Simulate a CPU-intensive task
}
return 'Operation completed';
}
console.log('Starting blocking operation...');
console.log(blockingOperation());
console.log('This will be delayed');
In this example, the blockingOperation function will block the event loop for 5 seconds, delaying all subsequent operations.
CPU-intensive Tasks and Their Impact
CPU-intensive tasks that can benefit from multithreading include:
- Complex mathematical calculations
- Image or video processing
- Data encryption and decryption
- Large dataset operations
These tasks can significantly slow down your application if run on the main thread, leading to poor user experience and reduced performance.
Improving Performance and Scalability
Multithreading in Node.js can help overcome these limitations by:
- Parallel execution: Running CPU-intensive tasks in parallel with the main application logic.
- Better resource utilization: Leveraging multi-core processors more effectively.
- Enhanced responsiveness: Keeping the main thread free to handle I/O operations and user interactions.
- Improved scalability: Handling more concurrent operations as your application grows.
By implementing multithreading, you can significantly boost your Node.js application’s performance, especially for computationally heavy tasks. In the following sections, we’ll explore different approaches to achieve this, including child processes, worker threads, and more.
Multithreading Approaches in Node.js
Node.js offers several ways to implement multithreading, each with its own strengths and use cases. In this section, we’ll explore the main approaches available to developers.
Child Processes
Child processes are separate Node.js instances that run independently from the main application. They’re useful for CPU-intensive tasks that don’t require shared memory.
Key features:
- Full isolation between processes
- Can run different Node.js versions or even different programs
- Communicate via IPC (Inter-Process Communication)
Example of creating a child process:
const { spawn } = require('child_process');
const child = spawn('node', ['child-script.js']);
child.stdout.on('data', (data) => {
console.log(`Child process output: ${data}`);
});
child.on('exit', (code) => {
console.log(`Child process exited with code ${code}`);
});
Worker Threads
Worker threads are a more recent addition to Node.js. They provide a way to run JavaScript in parallel, sharing memory with the main thread.
Key features:
- Lighter than child processes
- Share memory with the main thread
- Ideal for CPU-intensive JavaScript operations
Example of creating a worker thread:
const { Worker, isMainThread, parentPort } = require('worker_threads');
if (isMainThread) {
const worker = new Worker(__filename);
worker.on('message', (msg) => {
console.log(`Received message from worker: ${msg}`);
});
worker.postMessage('Hello from main thread');
} else {
parentPort.on('message', (msg) => {
console.log(`Received in worker: ${msg}`);
parentPort.postMessage('Hello from worker');
});
}
Cluster Module
The cluster module allows you to create child processes that all share server ports. It’s particularly useful for creating multi-core HTTP servers.
Key features:
- Easy to scale across multiple CPU cores
- Built-in load balancing
- Ideal for web server applications
Example of using the cluster module:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
});
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Third-party Libraries
There are also third-party libraries for multithreading in Node.js that simplify process, offering additional features and abstractions. Some popular ones include:
These libraries often provide high-level APIs for creating and managing threads or processes, making it easier to implement multithreading in your applications.
Child Processes in Node.js
Child processes are a powerful feature in Node.js that allow you to spawn new Node.js instances or other executables. They’re particularly useful for CPU-intensive tasks and for running system commands.
Brief Overview
Child processes in Node.js create separate instances of the V8 engine. This means they run independently from the main application, with their own memory space and event loop.
Use Cases
Child processes are ideal for:
- CPU-intensive computations
- Running system commands
- Executing scripts in other languages
- Isolating unstable or experimental code
Implementation Methods
Node.js provides several methods to create child processes:
- spawn(): Launches a new process with a given command.
- exec(): Spawns a shell and runs a command within that shell.
- execFile(): Similar to exec(), but doesn’t spawn a shell.
- fork(): A special case of spawn() for creating Node.js processes.
Pros For Child Process
- Full isolation between processes
- Can run different Node.js versions or even different programs
- Excellent for CPU-bound tasks and running system commands
Cons For Child Process
- Higher memory usage compared to threads
- Slower inter-process communication
- More complex to set up and manage for simple tasks
Best Practices For Child Process
- Use child processes for CPU-intensive tasks to avoid blocking the main thread.
- Implement proper error handling and logging for child processes.
- Be mindful of resource usage, especially when spawning multiple child processes.
- Use the appropriate method (spawn, exec, execFile, or fork) based on your specific needs.
- Consider using a process manager like PM2 for managing multiple Node.js processes in production.
Worker Threads in Node.js
NodeJS Worker Threads provide a way to run JavaScript code in parallel within a Node.js application. They are particularly useful for CPU-intensive tasks that can benefit from multi-core processing.
Introduction to Worker Threads
Worker Threads were introduced in Node.js version 10 and became stable in version 12. They allow you to create multiple threads within a single Node.js process, sharing memory and efficiently passing data between threads.
When to Use Worker Threads
- CPU-intensive computations
- Processing large datasets
- Parallel execution of JavaScript code
- Tasks that require shared memory access
Pros For Worker Threads
- Shared memory access
- Efficient for CPU-bound tasks
- Lighter than child processes
- Native to JavaScript/Node.js environment
Cons For Worker Threads
- Limited to JavaScript code
- Requires careful management to avoid race conditions
- Not suitable for I/O-bound tasks (use async operations instead)
Best Practices
- Use Worker Threads for CPU-intensive tasks that can be parallelized.
- Implement proper error handling for each worker.
- Be mindful of shared state and potential race conditions.
- Use appropriate data passing methods based on your needs (structured cloning vs. SharedArrayBuffer).
- Consider using a thread pool to manage multiple workers efficiently.
Cluster Module for Multithreading
The Cluster module is a built-in Node.js module that allows you to create child processes that share server ports. It’s particularly useful for taking advantage of multi-core systems to handle load.
Understanding the Cluster Module
The Cluster module enables you to create a small network of separate Node.js processes which can share the same server port. This is especially beneficial for web server applications, as it allows you to distribute incoming connections across multiple cores.
When to use Clustering Works in Node.js
- A master process that forks multiple worker processes
- Worker processes that handle incoming requests
- Automatic load balancing of connections among the workers
Pros For Clustering
- Easy to implement and scale across multiple CPU cores
- Built-in load balancing
- Ideal for web server applications
- Automatic restart of crashed workers
Cons For Clustering
- Limited to forking the main application
- Shared server ports, but not shared memory between processes
- Potential for increased memory usage compared to a single process
Best Practices For Clustering
- Use the Cluster module for web server applications to take advantage of multi-core systems.
- Implement proper error handling and logging for worker processes.
- Consider using a process manager like PM2 for additional features and easier management in production.
- Be mindful of shared resources (like databases) when scaling with clusters.
- Implement graceful shutdown mechanisms for worker processes.
Third-party Libraries for Multithreading
While Node.js provides built-in modules for multithreading, there are several third-party libraries that offer additional features and abstractions to simplify multithreading in Node.js applications.
Popular Libraries
Let’s explore some of the most popular third-party libraries for multithreading in Node.js:
1. Parallel.js
Parallel.js is a library that makes it easy to parallelize JavaScript code across multiple CPU cores.
key features :
- Simple API for parallel processing
- Works in Node.js and in the browser
- Supports map and reduce operations
2. Threads.js
Threads.js provides a straightforward way to create and manage worker threads in Node.js.
key features:
- Promise-based API
- Automatic thread pool management
- Support for transferable objects
3. Workerpool
Workerpool offers a pool of workers for both the browser and Node.js, providing an easy way to offload CPU-intensive tasks.
key features:
- Dynamic pool size
- Supports both synchronous and asynchronous tasks
- Works in Node.js and in the browser
Features and Use Cases
- Simplified APIs: They often provide higher-level abstractions over Node.js’s built-in multithreading capabilities.
- Automatic Thread Management: Many libraries handle thread creation, termination, and pooling automatically.
- Cross-platform Compatibility: Some libraries work in both Node.js and browser environments.
- Advanced Features: Features like automatic load balancing, promise-based APIs, and support for transferable objects are common.
Choosing the Right Library
- Project Requirements: Assess your specific needs for parallelism and concurrency.
- API Simplicity: Look for libraries with intuitive APIs that align with your coding style.
- Performance: Evaluate the performance overhead of the library.
- Community Support: Choose libraries with active maintenance and a supportive community.
- Documentation: Comprehensive documentation and examples are crucial for effective implementation.
Best Practices
- Benchmark: Compare the performance of different libraries for your specific use case.
- Error Handling: Implement robust error handling mechanisms when working with threads.
- Resource Management: Be mindful of resource usage, especially when creating large numbers of threads.
- Keep It Simple: Use multithreading only when necessary. For I/O-bound tasks, Node.js’s built-in asynchronous capabilities are often sufficient.
- Stay Updated: Keep your chosen library up-to-date to benefit from performance improvements and bug fixes.
Conclusion
Thank you for your patience. We’ve now covered all the main sections of our comprehensive guide on multithreading in Node.js. The next step would be to wrap up the blog post with a conclusion. Let’s do that now.
Multithreading in Node.js opens up a world of possibilities for building high-performance, scalable applications. Throughout this guide, we’ve explored various approaches to implement multithreading, each with its own strengths and use cases.
Key Takeaways
- Understanding Node.js’s Single-Threaded Nature: We started by recognizing the limitations of Node.js’s single-threaded model, which set the stage for why multithreading is sometimes necessary.
- Choosing the Right Tool: Each approach has its ideal use cases. Child processes for isolated tasks, worker threads for shared memory operations, the cluster module for web servers, and third-party libraries for specific needs.
- Performance Considerations: We’ve emphasized the importance of benchmarking, proper resource management, and avoiding oversubscription of CPU cores.
- Best Practices: We’ve outlined crucial practices like error handling, graceful shutdowns, and continuous monitoring to ensure robust multithreaded applications.
The Future of Multithreading in Node.js
As Node.js continues to evolve, we can expect further improvements in its multithreading capabilities. The introduction of worker threads was a significant step, and future versions may bring even more efficient ways to handle parallel processing.
Final Thoughts
Multithreading is a powerful tool in a Node.js developer’s arsenal, but it’s not a silver bullet. Always evaluate whether the added complexity of multithreading is justified by the performance gains for your specific use case. In many scenarios, Node.js’s asynchronous, event-driven model is sufficient and can be easier to manage.
remember :
- Start with a single-threaded approach and optimize your code.
- Use profiling tools to identify genuine bottlenecks.
- Implement multithreading only when necessary and where it provides clear benefits.
- Always follow best practices for error handling, resource management, and testing.
By understanding the concepts and techniques covered in this guide, you’re now well-equipped to leverage multithreading in your Node.js applications effectively. Whether you’re building a high-traffic web server, processing large datasets, or performing complex computations, you have the knowledge to choose the right multithreading approach and implement it successfully.
FAQs
Is Node.js truly single-threaded?
Node.js operates on a single main thread for executing JavaScript code. However, it uses additional threads for certain operations, like file I/O, through its libuv library. The introduction of Worker Threads has also added true multithreading capabilities to Node.js.
Can multithreading solve all performance issues in Node.js?
No, multithreading is not a universal solution. It’s most effective for CPU-bound tasks. For I/O-bound operations, Node.js’s asynchronous model is often more efficient. Always profile your application to identify the real bottlenecks before implementing multithreading.
How can I share data between threads?
Worker Threads can share data through SharedArrayBuffer for direct memory sharing, or by passing serializable JavaScript objects between threads. Child Processes can communicate through inter-process communication (IPC) channels.
3 Comments
Comments are closed.