mongoose Schema Design
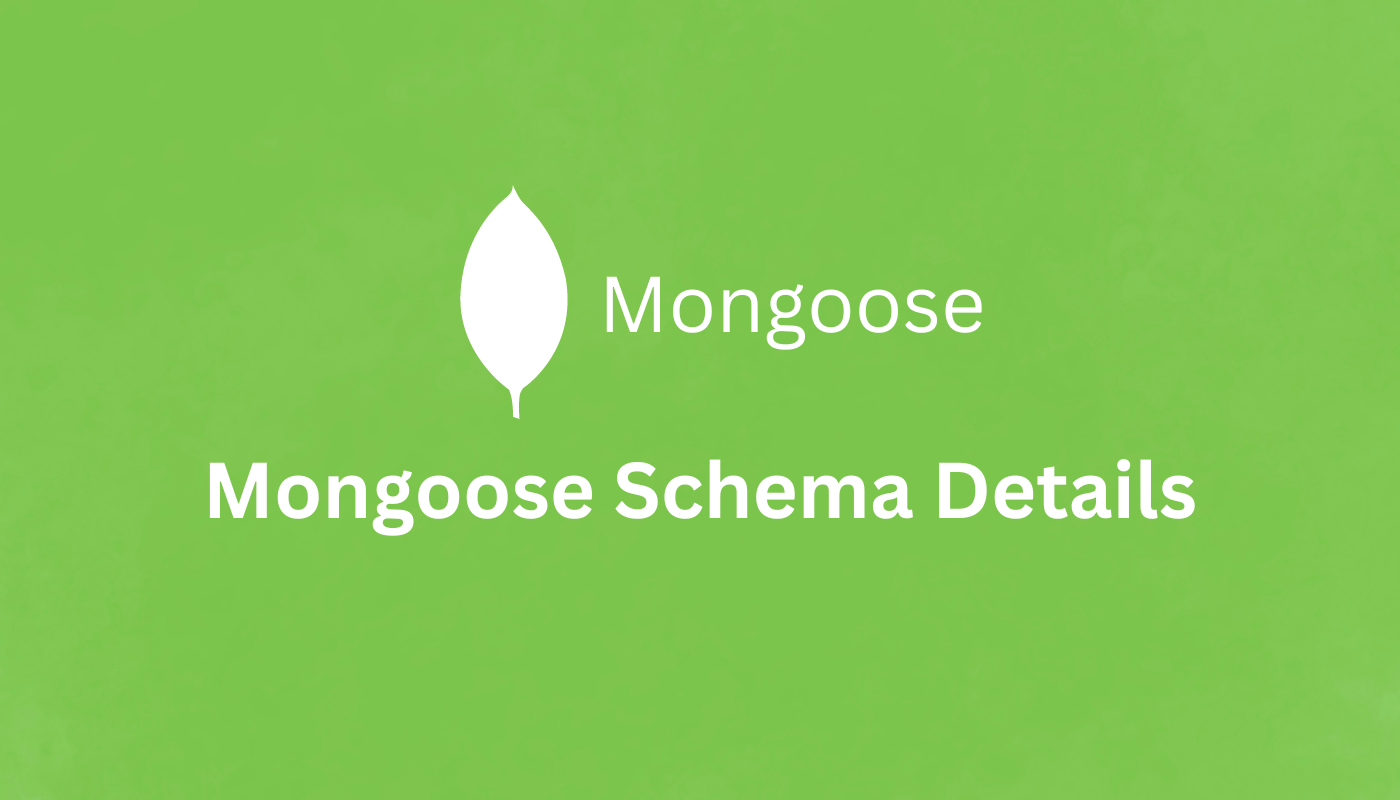
Introduction to Mongoose and MongoDB
When it comes to working with databases in JavaScript, MongoDB is one of the most popular NoSQL databases. Unlike traditional SQL databases, which store data in tables, MongoDB stores data in a flexible, document-based format, making it easier to work with large datasets that don’t always follow a strict schema.
MongoDB stores data in documents, which are like JSON objects. For example, if you’re storing user data, a document might look like this:
{
"name": "John",
"email": "john@example.com",
"age": 25
}
This flexibility is great, but sometimes we want a bit more structure to our data. That’s where Mongoose comes in. Mongoose is an Object Data Modeling (ODM) library for MongoDB, and it provides a way to define a schema for your data. A schema ensures that your documents follow a consistent structure and makes it easier to work with MongoDB in a structured and predictable way.
Why Use Mongoose?
- Schema Definitions: With Mongoose, you can define a schema for your documents, making sure the data you store has a consistent structure. For example, you might want to make sure that every user document has a name, email, and age field.
- Validation: Mongoose allows you to add rules to your schema. For example, you can specify that the email field must be unique or that the age field must be a number greater than 18.
- Querying Made Easy: Mongoose simplifies querying data from MongoDB. You can easily create, read, update, and delete documents using Mongoose methods.
- Middleware and Hooks: Mongoose allows you to run code before or after certain database operations, making it easy to add custom logic (like hashing passwords) at the right time.
Table of Contents
What is a Mongoose Schema?
A Mongoose schema is essentially a blueprint for your MongoDB documents. It defines the shape of the documents, specifying what fields they will have and what types of data each field will hold. While MongoDB itself doesn’t enforce a schema, Mongoose allows you to define one to make sure your documents follow a consistent structure
.Think of a schema as a rulebook for how your data should look. When you define a schema, you’re telling Mongoose exactly what kind of data to expect and how to handle it. For example, you can define that a user should have a name field of type String, an email field that is required, and an age field that must be a Number.
Here’s a basic example of a Mongoose schema for a user:
const mongoose = require('mongoose');
// Define the schema
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
This schema tells Mongoose that every User document should have:
- A name that is a string.
- An email that is a string.
- An age that is a number.
The Role of a Schema
- Defining Structure: The primary purpose of a Mongoose schema is to define the structure of the documents in a MongoDB collection. This makes it easier to work with your data because you always know what to expect.
- Adding Validations: A schema allows you to add validation rules, such as making certain fields required or enforcing that a field only accepts specific types of data (we’ll dive deeper into this later).
- Schema vs. Model: It’s important to note that a schema alone isn’t enough to interact with MongoDB. Once you define a schema, you’ll need to create a model from it. The model is what actually allows you to interact with the database, perform CRUD operations, and manipulate documents.
How to Create A Basic Mongoose Schema ?
Now that you understand what a schema is, let’s create one! In this section, we’ll go step-by-step to define a basic schema in Mongoose and explore how you can structure your data.
Step 1: Set Up Mongoose
Before creating a schema, you need to make sure Mongoose is installed and connected to your MongoDB database. If you haven’t already done this, here’s how you can install Mongoose and connect to MongoDB:
npm install mongoose
Then, you can create a connection:
const mongoose = require('mongoose');
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true
});
This will connect Mongoose to your local MongoDB database. Make sure MongoDB is running on your system.Or you can use mongoDB atlas for this
- explore complete mongoDB and mongoDB atlas
- complete guide for connecting mongoDB database with mongoose
Step 2: Define a Basic Schema
Let’s create a simple schema to represent users. A User document will have three fields: name, email, and age. Here’s how you can define the schema:
// Define a basic user schema
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
- name: The user’s name, stored as a string.
- email: The user’s email address, stored as a string.
- age: The user’s age, stored as a number.
Step 3: Create a Model from the Schema
Once you’ve defined the schema, you need to create a model. The model allows you to interact with the MongoDB database, perform CRUD (Create, Read, Update, Delete) operations, and work with documents.
Here’s how you create a model:
// Create a model based on the user schema
const User = mongoose.model('User', userSchema);
With the User model, you can now create and manage user documents in the users collection of your MongoDB database.
Step 4: Create and Save a Document
Now that we have our model, let’s create and save a user document in the database.
// Create a new user document
const newUser = new User({
name: 'Alice Smith',
email: 'alice@example.com',
age: 30
});
// Save the document to the database
newUser.save()
.then(() => console.log('User saved successfully!'))
.catch(error => console.log('Error saving user:', error));
When you run this code, Mongoose will create a new document in the users collection, and you’ll see a message in the console confirming that the document was saved successfully.
Key Points:
- Schema Definition: We used String and Number as basic data types for fields.
- Model Creation: The User model allows you to interact with the database.
- Saving Documents: The save() method inserts a new document into MongoDB.
Schema Types and Data Validation
One of the most powerful features of Mongoose is the ability to define schema types and apply validation rules to your data. Schema types allow you to specify what kind of data is stored in each field, while validation ensures that your data meets certain criteria before it’s saved to the database.
Common Schema Types
Mongoose supports a variety of schema types that allow you to model your data accurately. Let’s look at some of the most commonly used types:
- String: Used to store text data.
- Number: Used to store numeric data.
- Boolean: Used for true or false values.
- Date: Used to store date and time information.
- Array: Used to store lists of data.
- ObjectId: Used for referencing other documents in the database.
- Mixed: Allows any type of data to be stored. It’s a flexible schema type but should be used with caution as it lacks strict validation.
Validation in Mongoose
Mongoose allows you to add validation rules to each field in the schema. For example, you might want to require that the name field is always present, or that the age field must be greater than 18.
const userSchema = new mongoose.Schema({
name: {
type: String,
required: true, // Name is required
},
email: {
type: String,
required: true, // Email is required
unique: true // Email must be unique
},
age: {
type: Number,
min: 18, // Minimum age is 18
max: 65 // Maximum age is 65
}
});
Let’s break this down:
- required: Ensures that the field is not empty when creating a document.
- unique: Ensures that no two documents have the same value for the email field.
- min/max: Ensures that the age field is within a certain range.
Custom Validation
Sometimes you need to write custom validation logic for fields. Mongoose allows you to define custom validators using the validate option.
const userSchema = new mongoose.Schema({
name: String,
age: {
type: Number,
validate: {
validator: function(value) {
return value % 2 === 0; // Age must be an even number
},
message: props => `${props.value} is not an even number!`
}
}
});
If a user tries to save an odd number for age, they’ll receive an error message saying that the value is not valid.
Default Values
You can also set default values for fields. If a field is not provided when creating a document, Mongoose will use the default value.
const userSchema = new mongoose.Schema({
name: String,
isActive: {
type: Boolean,
default: true // Default value is true
}
});
Working with Embedded Documents and Subdocuments
In MongoDB, you can store related data within a document using embedded documents or subdocuments. Mongoose makes it easy to define these relationships within a schema, allowing you to organize your data in a more hierarchical structure.
What Are Embedded Documents?
Embedded documents are documents stored inside other documents. This structure is useful when you want to represent data that naturally belongs together. For example, a User document may contain an embedded Address document.
Instead of storing the Address in a separate collection and referencing it, you can embed it directly inside the User document. This simplifies the data model when the relationship between documents is one-to-one or one-to-few.
Defining Subdocuments in Mongoose
You can define subdocuments within a Mongoose schema by embedding another schema inside a parent schema.
const addressSchema = new mongoose.Schema({
street: String,
city: String,
postalCode: String
});
const userSchema = new mongoose.Schema({
name: String,
email: String,
address: addressSchema // Embedded address schema
});
In this case, the address field is an embedded subdocument inside the User schema. When a new user is created, the address can be included directly in the document.
Working with Subdocuments
Once you have defined subdocuments, you can work with them just like you would with regular fields.
const User = mongoose.model('User', userSchema);
// Create a new user with an embedded address
const newUser = new User({
name: 'John Doe',
email: 'john@example.com',
address: {
street: '123 Main St',
city: 'New York',
postalCode: '10001'
}
});
newUser.save()
.then(() => console.log('User with embedded address saved!'))
.catch(error => console.log('Error:', error));
When saved, this will create a document in the users collection with the following structure:
{
"_id": "some-id",
"name": "John Doe",
"email": "john@example.com",
"address": {
"street": "123 Main St",
"city": "New York",
"postalCode": "10001"
}
}
Nested Subdocuments
Mongoose also allows you to nest subdocuments within other subdocuments, creating deeply structured data.
For example, let’s say you want to store a list of Orders within a User document, and each order includes a list of Products:
const productSchema = new mongoose.Schema({
productName: String,
price: Number
});
const orderSchema = new mongoose.Schema({
orderDate: Date,
products: [productSchema] // Array of products
});
const userSchema = new mongoose.Schema({
name: String,
email: String,
orders: [orderSchema] // Array of orders
});
In this case, you have a User document with an array of Order subdocuments, and each order contains an array of Product subdocuments.
You can create a new user with nested subdocuments like this:
const User = mongoose.model('User', userSchema);
const newUser = new User({
name: 'Alice Smith',
email: 'alice@example.com',
orders: [
{
orderDate: new Date(),
products: [
{ productName: 'Laptop', price: 1000 },
{ productName: 'Mouse', price: 50 }
]
}
]
});
newUser.save()
.then(() => console.log('User with nested orders and products saved!'))
.catch(error => console.log('Error:', error));
The resulting document will look like this:
{
"_id": "some-id",
"name": "Alice Smith",
"email": "alice@example.com",
"orders": [
{
"orderDate": "2024-09-20T12:00:00Z",
"products": [
{ "productName": "Laptop", "price": 1000 },
{ "productName": "Mouse", "price": 50 }
]
}
]
}
Schema Methods and Statics
Mongoose offers powerful ways to extend the functionality of your schemas. By using methods and statics, you can add custom logic to your models and make them easier to work with. This is particularly useful when you want to encapsulate specific behavior related to your data.
complete guide of mongoose schema methods and statics in full details
Schema Methods
Schema methods are functions that you can define on an individual document. This means that when you create a document (like a user or product), you can call methods directly on that instance. These methods are great for performing actions specific to a particular document.
To define a method in Mongoose, you add a function to the methods property of the schema. For example, let’s add a method to a User schema that returns the full name of a user:
const userSchema = new mongoose.Schema({
firstName: String,
lastName: String
});
// Adding a method to get the full name
userSchema.methods.getFullName = function() {
return `${this.firstName} ${this.lastName}`;
};
const User = mongoose.model('User', userSchema);
// Creating a new user and using the method
const user = new User({
firstName: 'John',
lastName: 'Doe'
});
console.log(user.getFullName()); // Output: 'John Doe'
In this example, we created a method called getFullName that combines the firstName and lastName fields into a full name. The method is called directly on the document instance.
Schema Statics
Unlike methods, which work on individual documents, statics are functions that are called directly on the model itself. They are useful for actions that apply to the model as a whole, such as querying the database or performing some operation on multiple documents.
You can define statics on a schema by adding a function to the statics property of the schema. Here’s how you can create a static function that finds a user by their email:
const userSchema = new mongoose.Schema({
name: String,
email: String
});
// Adding a static method to find a user by email
userSchema.statics.findByEmail = function(email) {
return this.findOne({ email });
};
const User = mongoose.model('User', userSchema);
// Example usage:
User.findByEmail('john@example.com').then(user => {
console.log(user);
});
Here, the static findByEmail function is added to the User model. Instead of working on a single document, this method queries the collection to find a document with the given email.
Virtuals in Mongoose
In Mongoose, virtuals are fields that are not stored in the MongoDB database but are computed dynamically. They allow you to define additional properties on your documents without actually saving them to the database, making them ideal for cases where you want to display or use data that’s derived from existing fields.
What Are Virtuals?
Virtuals are essentially “virtual” properties that exist only in your application code. They don’t get persisted in the database. For example, you may want to display a user’s full name by combining their first name and last name without needing a dedicated fullName field in the database.
How to Define a Virtual ?
To create a virtual in Mongoose, you use the schema.virtual() method. Let’s walk through a simple example of defining a virtual for a user’s full name.
const userSchema = new mongoose.Schema({
firstName: String,
lastName: String
});
// Defining a virtual for full name
userSchema.virtual('fullName').get(function() {
return `${this.firstName} ${this.lastName}`;
});
const User = mongoose.model('User', userSchema);
// Example usage
const user = new User({
firstName: 'John',
lastName: 'Doe'
});
console.log(user.fullName); // Output: 'John Doe'
In this example, the fullName virtual field is computed on the fly based on the firstName and lastName fields. It doesn’t get stored in MongoDB but is available for use when you need it.
Mongoose Middleware
Middleware in Mongoose are functions that are executed at specific stages of a document’s lifecycle. They allow you to perform actions before or after certain operations, such as saving, updating, or deleting documents. This feature is useful for adding custom validation, logging, or modifying data during these operations.
Types of Middleware
Mongoose supports several types of middleware, primarily categorized into two groups: document middleware and model middleware.
- Document Middleware: This middleware operates on individual documents and can be used for actions like save, validate, and remove.
- Model Middleware: This middleware operates on the model level and is used for actions like init and aggregate.
Mongoose Population
Population in Mongoose is a powerful feature that allows you to replace references to documents in one collection with the actual documents from another collection. This is particularly useful in cases where you have related data stored in separate collections, and you want to retrieve that data in a single query.
Why Use Population?
When designing a database schema, it’s common to reference documents from one collection in another. For instance, you might have a User collection and a Post collection, where each post references a user. Instead of manually querying the user data after retrieving posts, you can use population to fetch the related data in a single call.
Error Handling in Mongoose
Effective error handling is crucial for building robust applications. Mongoose provides several ways to manage errors that may arise during operations such as saving, querying, or validating documents. Understanding how to handle these errors can help you provide better feedback to users and maintain application stability.
Common Error Types
- Validation Errors: These occur when a document fails to pass validation defined in the schema.
- Cast Errors: These happen when a value is not of the expected type, often due to invalid _id formats.
- Duplicate Key Errors: These arise when trying to save a document with a unique field value that already exists in the database.
- Database Connection Errors: These occur when there are issues connecting to MongoDB.
Mongoose Aggregation
Aggregation in Mongoose is a powerful way to process and analyze your data in MongoDB. It allows you to perform operations such as filtering, grouping, sorting, and reshaping data, enabling you to extract meaningful insights from your collections.
What is Aggregation?
Aggregation is a framework that processes data records and returns computed results. You can think of it as a pipeline where data flows through a series of stages, each performing a specific operation. Mongoose provides an elegant API for working with aggregation pipelines.
Basic Aggregation Stages
The aggregation pipeline consists of multiple stages, each represented as an object. Here are some of the most common stages:
- $match: Filters the documents to pass only the ones that match the specified condition.
- $group: Groups documents by a specified key and can perform accumulations (e.g., count, sum).
- $sort: Sorts the documents based on a specified field.
- $project: Reshapes each document by including, excluding, or adding new fields.
Conclusion
Mongoose is a powerful ODM (Object Data Modeling) library that streamlines working with MongoDB by providing structured data management through schemas. This ensures consistent document structure and facilitates data validation and integrity.
With Mongoose, you can create organized data models that support features like validation, middleware, and efficient data handling methods. It also allows for embedded and subdocuments, enabling complex relationships without sacrificing clarity.
Additionally, Mongoose supports virtuals—derived properties that aren’t stored in the database—keeping your data model lean while enhancing functionality.
Overall, Mongoose is essential for JavaScript developers who want to utilize MongoDB’s flexibility while maintaining structure and rigor. Its straightforward API simplifies CRUD operations and error handling, making it a preferred choice for building robust applications.
FAQs
Why should I use Mongoose with MongoDB?
Mongoose provides a structured way to define your data models, ensuring consistency, validation, and easier management of relationships between data.
Is Mongoose compatible with TypeScript?
Yes, Mongoose has TypeScript definitions, allowing you to use it with TypeScript for type safety and better developer experience.
How do I perform CRUD operations with Mongoose?
Mongoose provides simple methods for creating, reading, updating, and deleting documents using model instances, making it easy to manage your data.
2 Comments
Comments are closed.