complete guide on modification of configuration with react-app-rewired
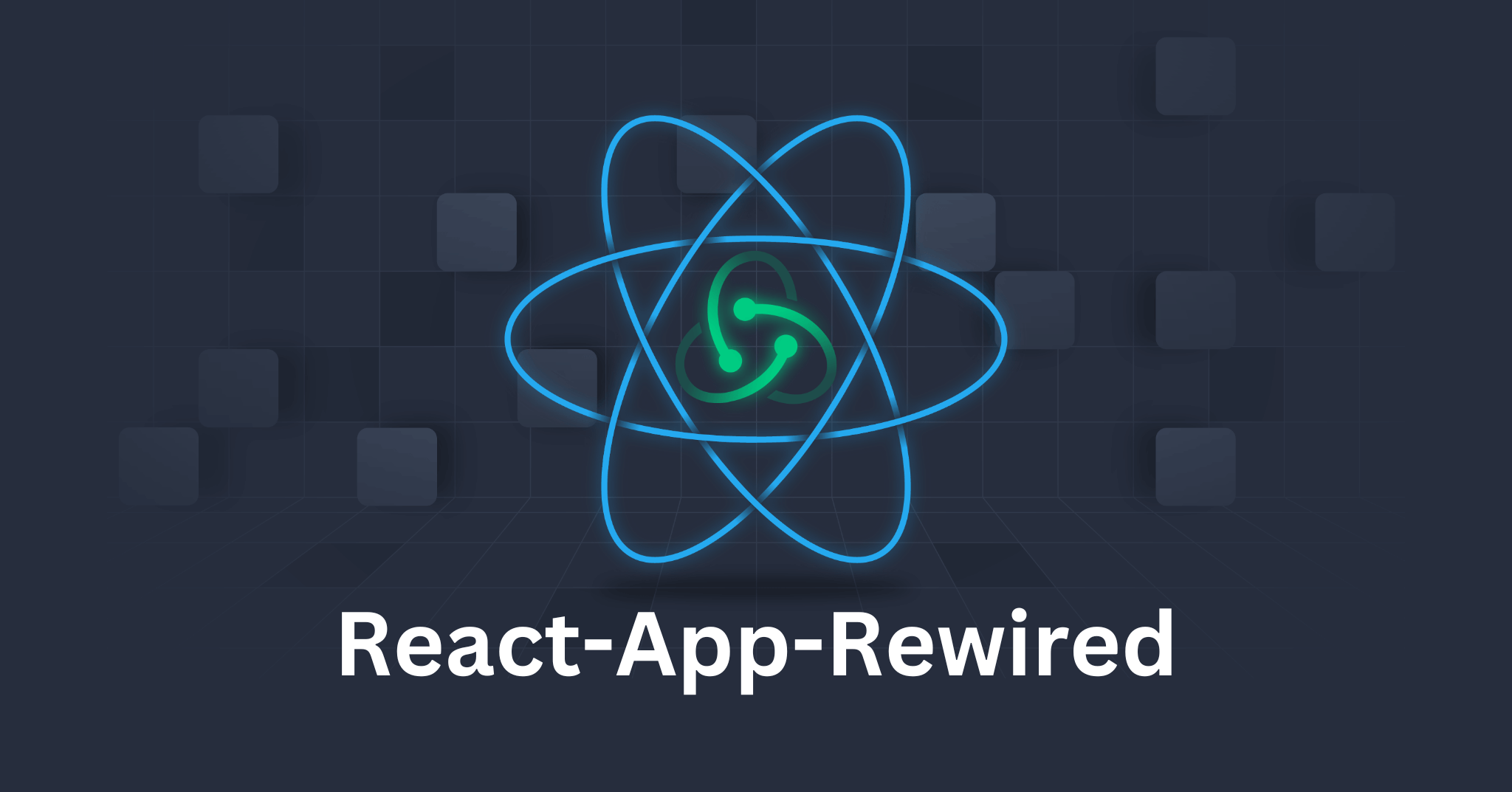
Explore complete guide for React
introduction to react-app-wired
React-app-rewired is a usefull tool for React developers that enables them to modify the configuration of their React applications without having to eject from Create React App.
Now let’s break that down this. When you create a React app using Create React App. It sets up a bunch of configurations behind the scenes to make your development process smooth. But sometimes, you might want to customize these configurations to add new features or tweak existing ones. This is where React-app-rewired comes into play.
Instead of ejecting your app from Create React App (which basically means taking control of all the configurations yourself which can be a bit complex) now you can use React-app-rewired to override certain configurations without the need for ejecting.
So why is this usefull ? Well imagine you want to use a newer version of a tool like Babel or Webpack in your React app but Create React App hasn’t updated to that version yet. With React-app-rewired you can easily update these tools without having to deal with the complexities of ejecting.
In simple words React-app-rewired makes it easier for React developers to customize their projects without going deep into the messy details of configuration management. It’s like having a little helper that lets you tweak things without making a big mess.
Table of Contents
let’s get started with react app rewired
first install react app rewired to your react app
First you need to install React-app-rewired and its related dependencies. Open your terminal or command prompt and navigate to your Create React App project directory. Then run the following command:
npm install react-app-rewired customize-cra --save-dev
This will install React-app-rewired along with Customize-CRA which is a package that allows you to customize Create React App configurations.
Create Config file
Next you need to create a configuration file. In the root directory of your project create a file called config-overrides.js
. This file will contain the customizations you want to make to your Create React App configurations.
Configure Babel, Webpack etc.
Inside config-overrides.js
you can import functions from customize-cra to customize various aspects of your project. For example if you want to add a new Babel plugin you can do so like this:
const { addBabelPlugin } = require('customize-cra');
module.exports = function override(config, env) {
// Add a new Babel plugin
config = addBabelPlugin(['@babel/plugin-proposal-decorators', { legacy: true }]);
return config;
};
This is just an example. You can customize other aspects of your project such as Webpack configuration, ESLint rules etc. By using the functions provided by Customize-CRA.
Update Scripts in package.json
Finally, you need to update the scripts in your package.json
file to use React app rewired instead of the default react-scripts
. In the scripts section of your package.json
modify the start
, build
and test
scripts like this:
"scripts": {
"start": "react-app-rewired start",
"build": "react-app-rewired build",
"test": "react-app-rewired test",
...
}
And that’s it. You have successfully set up and configured React app rewired in your Create React App project. Now you can easily customize your project’s configurations without having to eject from Create React App.
Customizing Webpack Configurations with React-app-rewired
Adding Loaders for loading cSS module
Loaders are used to process files in your project. For example if you want to add support for loading CSS modules. You can use the addWebpackModuleRule function provided by Customize-CRA.
const { addWebpackModuleRule } = require('customize-cra');
module.exports = function override(config, env) {
// Add support for loading CSS modules
config = addWebpackModuleRule({
test: /\.module\.css$/,
use: ['style-loader', 'css-loader']
})(config);
return config;
};
Adding Plugins to app
Plugins extend the functionality of Webpack. For Example if you want to use the HtmlWebpackPlugin to generate an HTML file for your project you can add it using the addWebpackPlugin function.
const HtmlWebpackPlugin = require('html-webpack-plugin');
const { addWebpackPlugin } = require('customize-cra');
module.exports = function override(config, env) {
// Add HtmlWebpackPlugin to generate HTML file
config = addWebpackPlugin(new HtmlWebpackPlugin({
template: 'public/index.html',
filename: 'index.html'
}))(config);
return config;
};
Modify Existing Settings of app
You can also modify existing settings in the Webpack configuration. For example, if you want to change the output directory for your build files, you can do so by accessing the output property of the configuration object.
module.exports = function override(config, env) {
// Change output directory for build files
config.output.path = path.resolve(__dirname, 'custom-output');
return config;
};
Advanced Customizations
React-app-rewired allows for more advanced customizations such as modifying optimization settings, configuring aliases for module imports and more. You can explore the full range of options provided by Customize-CRA to make Webpack configurations to your specific needs.
Best Practices, Tips and Tricks for Using React-app-rewired
best practices for react app rewired
- Keep Configuration Separate : Maintain a clear separation between your application code and configuration overrides. Place your
config-overrides.js
file in the root directory of your project and keep it focused on customizing Webpack configurations. - Use Modular Customizations : Break down your customizations into small and modular functions using the functions provided by Customize-CRA. This makes it easier to manage and understand your configuration overrides and allows for better reusability.
- Test Changes Thoroughly : Always test your configuration changes thoroughly to make sure they work as expected. Run your application in development and production modes and verify that any customizations you applied behave correctly.
- Document Customizations : Document any customizations you make to your project’s configurations. Include comments in your
config-overrides.js
file to explain the purpose of each customization and any dependencies or considerations. - Stay Updated : Keep React-app-rewired and Customize-CRA up to date with the latest versions to benefit from bug fixes, performance improvements and new features. Regularly check for updates and consider updating your project accordingly.
Tips and Tricks for react-app-rewired
- Explore Available Functions : Familiarize yourself with the functions provided by Customize-CRA such as
addWebpackModuleRule
,addWebpackPlugin
and override. Explore the documentation to discover additional customization options and possibilities. - Utilize Community Packages : Take advantage of community packages and plugins that extend the functionality of React-app-rewired. There are many third-party packages available for common use cases such as optimizing images, configuring environment variables and more.
- Use Conditional Customizations : Apply conditional logic in your
config-overrides.js
file to customize configurations based on specific conditions such as the environment or build target. This allows you to create more flexible and dynamic configurations.
Potential Pitfalls to Avoid in react-app-rewired
- Overcomplicating Configurations : Avoid overcomplicating your configuration overrides with unnecessary customizations. Keep your configurations simple and focused on addressing specific requirements to avoid complexity and potential issues.
- Breaking Changes with Updates : Be cautious when updating React-app-rewired or Customize-CRA. As updates may introduce breaking changes or require adjustments to your configuration overrides. Always review release notes and test updates in a controlled environment before applying them to your project.
- Ignoring Create React App Updates : Don’t ignore updates to Create React App itself. While React-app-rewired provides flexibility for customizing configurations. It’s important to stay up to date with Create React App updates to benefit from improvements, security patches and new features.
Comparisons with Alternatives: React-app-rewired vs. Other npm Packages for Customizing Webpack Configurations
When it comes to customizing webpack configurations in Create React App projects. developers have a variety of npm packages to choose from. Let’s compare React-app-rewired with other popular alternatives
Feature / Aspect | React-app-rewired | craco | Custom webpack configurations | Ejecting from Create React App |
Integration with Create React App | Yes | Yes | No | Yes |
Ease of Use | Simple and straightforward API | Moderate | Complex | Moderate |
Compatibility with Create React App | Fully compatible with default configuration | Fully compatible with default configuration | Compatible but requires manual setup | Full control, but removes default configuration |
Extensibility and Modularity | Limited extensibility and modularity | More modular and extensible | Fully extensible | N/A |
Learning Curve | Low | Moderate | High | Moderate |
Maintenance Effort | Low | Moderate | High | Moderate |
Flexibility and Control | Limited compared to custom webpack configurations | Provides a balance between flexibility and control | Maximum flexibility and control | Maximum flexibility and control |
Applicability | Best suited for Create React App projects | Best suited for Create React App projects | Suitable for any project with webpack | Suitable for any project with webpack |
conclusion
In final conclusion React-app-rewired offers a user-friendly solution for React developers who want too personalize their projects without getting lost in the complexities of configuration management. It acts as a helpful companion that enable easy adjustments without creating chaos. By bypassing the need for extensive reconfiguration it simplifies the customization process and making it more approachable. However it’s essential to stay updated to recommended practices to make sure smooth operations. With React-app-rewired make your React application becomes a straightforward and accessible task.
FAQs
What is React-app-rewired ?
React-app-rewired is a tool designed for React developers to modify the configuration of their React applications without needing to eject from Create React App.
Can I revert changes made with React-app-rewired ?
Yes, since React-app-rewired only overrides specific configurations so you can revert changes by adjusting or removing the customizations made in the config-overrides.js
file.