Next jS Interview Questions Explained: A Practical Guide for Success
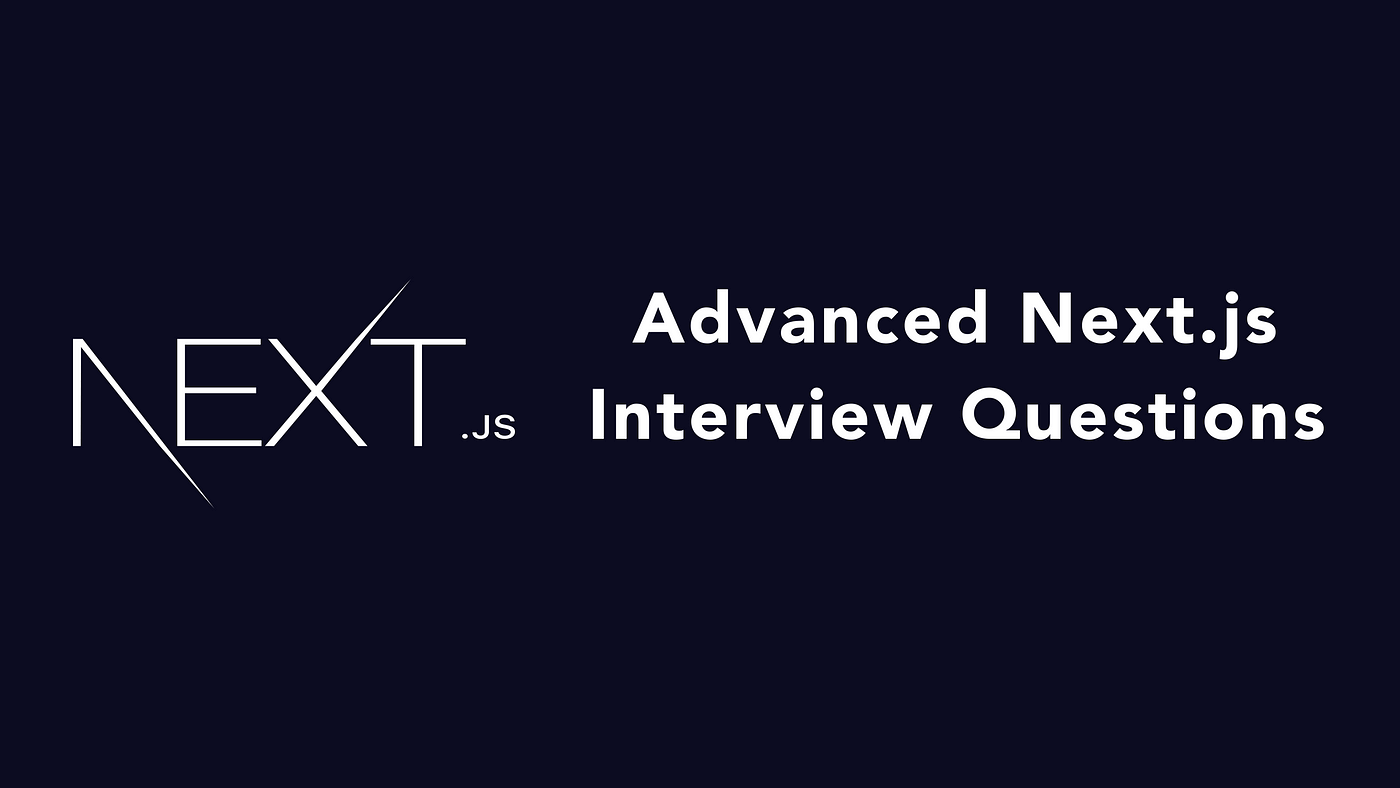
Before we jump into our guide about mastering Next JS interview questions. let’s talk about why Next JS is such a big deal in the world of web development and why knowing about it can really help you in interviews.
Next JS has become super popular because it makes building websites with React a easy job. It’s like a magic tool that takes care of a lot of complicated stuff for you that like making sure your website loads fast and looks great on different devices.
In now days a lot of companies are using Next JS to build their websites because it’s just so good at what it does. That means there’s a big demand for people who know how to use Next JS and if you can show that you are one of those people in your interviews. You will have a much better chance of landing a job.
So in this guide. we are going to cover all the important stuff you need to know about Next JS and we will give you some example questions that you might get asked in interviews. Whether you are brand new to Next JS or you have been using it for a while. Our goal is to help you feel confident and ready to tackle any Next JS interview that comes your way.
Table of Contents
What is Different between next JS and React JS ?
Next JS is a framework for building React applications. It’s like a toolbox that helps developers create web apps with React in a simpler and more organized way.
React by itself is just the engine. It’s like having a powerfull car without roads. You can make amazing things with it but you will have to set up everything from scratch like routing, server-side rendering and code splitting.
That’s where Next JS come in picture. It’s built on top of React that adding extra features and tools to make the development process smoother. Think of Next JS as the GPS system for your car that helps you navigate your project efficiently.
One big difference between NextJS and React is how they handle routing and server-side rendering. With React you typically use libraries like React Router for routing and server-side rendering can be a bit complex to set up. But with Next JS these things come built-in. You don’t have to worry about setting up routes or configuring server-side rendering because Next JS does it for you automatically. It is like having a built-in navigation system and a smooth road ahead.
Another difference is in the deployment process. With React you might need to set up a separate server to host your app. Which can be a bit of a hassle But with NextJS you can easily deploy your app to platforms like Vercel or Netlify with just a few clicks. It’s like having a dedicated driver to take care of the journey for you.
What are the main features of Next JS ?
- Automatic Code Splitting : With Next JS you don’t have to manually split your code into smaller chunks to improve performance. It automatically splits your code into smaller bundles so your users only download what they need when they need it. This helps reduce initial loading times and improves the overall performance of your app.
- Server-side Rendering (SSR) : One of the main features of Next JS is its built-in support for server-side rendering. This means that your React components can be rendered on the server before being sent to the client. Which can improve SEO, performance and user experience. No need to set up complex server-side rendering configurations.
- Static Site Generation (SSG) : In addition to server-side rendering. Next JS also supports static site generation. This allows you to pre-render pages at build time. which can be incredibly usefull for content-heavy websites or blogs. With SSG you can generate static HTML files for each page of your app that resulting in faster load times and better SEO.
- File-based Routing : Next JS simplifies routing by allowing you to create routes based on the file system. You can organize your pages into folders and files and Next JS will automatically generate routes based on their file names. This makes it easy to create and manage routes without the need for complex routing configurations.
- API Routes : Next JS provides built-in support for creating API routes that allow you to build backend functionality directly into your Next JS app. You can create API endpoints to handle data fetching, form submissions, authentication and more. All without the need for an external server.
- Automatic Static Optimization : Next JS automatically optimizes your app for static hosting by pre-rendering pages as static HTML files. This means that your app can be served directly from a CDN (Content Delivery Network) and in back that resulting in faster load times and better scalability.
How does routing work in Next JS ?
pages/
├── index.js
├── about.js
├── products/
│ ├── index.js
│ └── [id].js
├── blog/
│ ├── [...slug].js
- File-based Routing : In Next JS routes are based on the file system. Each
.js
,.jsx
,.ts
or.tsx
file inside the pages directory represents a route in your application. In Example block you can see a file namedabout.js
inside the pages directory. It will be accessible at the/about
route. In new version of Next JS there is another router call app router - Nested Routes : Next JS supports nested routes by organizing files into nested folders inside the pages directory. File structure like
pages/products/index.js
andpages/products/[id].js
that you can see in example block. Next JS will automatically generate routes like/products
and/products/:id
respectively.index.js
is represent main route of folder name. - Dynamic Routes : Next JS allows you to create dynamic routes by using brackets
[]
in file names. that you can see in example a file namedproducts/[id].js
would match any route like/products/mobile
and/products/leptop
etc. - Catch-All Routes : Next JS supports catch-all routes that denoted by using three dots (…) in dynamic route filenames. This allows you to match any number of segments in a URL and handle them dynamically. In last example a file named
pages/blog/[…slug].js
would match routes like/blog/post-1
and/blog/post-1/comments
etc.
What is Server-side Rendering (SSR) and how does Next JS support it ?
Server-side Rendering (SSR) is a technique used in web development where web pages are generated on the server and then sent to the client’s browser as fully-rendered HTML documents. This means that when a user requests a page the server dynamically generates the HTML content for that page. Including any data or dynamic content before sending it to the client. SSR is beneficial because it can improve the performance of web applications by reducing the amount of client-side rendering required which can lead to faster initial page loads and better search engine optimization (SEO) since search engine crawlers can easily index the fully-rendered HTML content.
Next JS has built-in support for Server-side Rendering (SSR) that making it easy for developers to implement SSR in their React applications without the need for complex configuration. here are some points
- Automatic SSR Setup : With Next JS setting up SSR is seamless. When you create a new Next JS project SSR is already configured out of the box so you can start building SSR enabled pages on the go without needing to set up any additional code or configuration.
- getServerSideProps : Next JS provides a special function called
getServerSideProps
that allows you to fetch data on the server side and pass it as props to your React components before rendering them. This function runs at request time on the server and can be used to fetch data from APIs, databases or other sources. The data returned fromgetServerSideProps
is serialized into the HTML response sent to the client and make sure that the page is fully rendered before it is delivered to the browser.
// pages/example.js
import React from 'react';
const ExamplePage = ({ data }) => (
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
export async function getServerSideProps(context) {
// Fetch data from an API or database
const res = await fetch('https://api.example.com/data');
const data = await res.json();
// Pass data as props to the component
return {
props: {
data
}
};
}
export default ExamplePage;
What are the different methods for data fetching in Next JS ?
data fetching with getStaticProps
This method is used for pre-rendering pages at build time. It fetches data during the build process and passes it as props to the page component. The fetched data is static and will not change between requests. This method is ideal for data that doesn’t change frequently and can be safely cached.
export async function getStaticProps() {
// Fetch data from an API or database
const res = await fetch('https://api.example.com/data');
const data = await res.json();
// Return data as props
return {
props: {
data
}
};
}
data fetching with getServerSideProps
This method is used for pre-rendering pages on each request. It fetches data at request time on the server and passes it as props to the page component. The fetched data is dynamic and can change on each request. This method is ideal for data that needs to be fetched server-side or for pages that require access to user-specific data.
export async function getServerSideProps() {
// Fetch data from an API or database
const res = await fetch('https://api.example.com/data');
const data = await res.json();
// Return data as props
return {
props: {
data
}
};
}
data fetching with getStaticPaths and getStaticProps
This method is used in conjunction with getStaticProps
for dynamic routes with pre-rendering. It specifies which paths should be pre-rendered at build time. The getStaticPaths
function returns an array of possible values for dynamic parameters in the URL.
export async function getStaticPaths() {
// Fetch possible values for dynamic parameter
const paths = ['/posts/1', '/posts/2', '/posts/3'];
// Return possible paths
return {
paths,
fallback: false // or 'blocking' or true
};
}
data fetching on client side
This method involves fetching data directly on the client-side. typically using built-in browser APIs like fetch or third-party libraries like Axios. Client-side data fetching is usefull for where data needs to be fetched in response to user interactions or for data that changes frequently on the client-side.
import { useEffect, useState } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const res = await fetch('/api/data');
const json = await res.json();
setData(json);
} catch (error) {
setError('Error fetching data');
}
};
fetchData();
}, []);
if (error) return <div>{error}</div>;
if (!data) return <div>Loading...</div>;
return (
<div>
{data.map(item => (
<div key={item.id}>{item.title}</div>
))}
</div>
);
}
benefits of server-side rendering in Next JS
- Improved Performance : SSR can significantly improve the performance of web applications by reducing the time it takes to render the initial page. Since the HTML content is generated on the server and sent to the client’s browser as a fully-rendered page so users can see content more quickly that make better user experience.
- Better Search Engine Optimization (SEO) : Search engines like Google can easily index the content of SSR pages because the HTML content is available in the initial response. This helps improve the discoverability of web pages and can lead to higher search engine rankings.
- Enhanced Social Sharing : When users share links to SSR pages on social media platforms the shared links contain fully-rendered HTML content. This make sure that the shared links display correctly and provide a preview of the content which can increase engagement and traffic to the website.
- Consistent User Experience : SSR make sure that users receive a consistent experience across different devices and browsers since the initial HTML content is the same for all users. This helps reduce inconsistencies and website behaves predictably for all users.
- Accessibility : SSR make sure that the content of web pages is accessible to users with disabilities or those using assistive technologies. Since the HTML content is available in the initial response, screen readers and other assistive technologies can easily parse and interpret the content and making it more accessible to all users.
- Security : SSR can help improve security by reducing the risk of client-side vulnerabilities such as cross-site scripting (XSS) attacks. Since SSR generates HTML content on the server. There is less dependent on client-side JavaScript that reducing the attack surface and potential security risks.
How do you optimize performance in a Next JS application ?
- Use Server-side Rendering (SSR) : using SSR in Next JS can improve performance by pre-rendering pages on the server and sending fully-rendered HTML to the client’s browser. This reduces the time it takes to render the initial page and improves perceived performance for users.
- Implement Incremental Static Regeneration (ISR) : ISR is a feature in Next JS that allows you to update static pages at runtime without redeploying the entire application. By using ISR you can make sure that your static pages remain up-to-date while minimizing downtime and improving overall performance.
- Optimize Images and Media : Use optimized images and media files to reduce file sizes and improve loading times. Next JS provides built-in support for image optimization using the
next/image
component which automatically optimizes images for different device sizes and screen resolutions. - Code Splitting : Break your code into smaller and more manageable chunks and use code splitting to only load the code that is needed for each page. This helps reduce initial loading times and improves overall performance by minimizing the amount of code that needs to be downloaded and executed by the browser.
- Bundle Size Analysis : Analyze your bundle size using tools like Webpack Bundle Analyzer or Next JS built-in tooling to identify and eliminate unnecessary dependencies, unused code or large libraries that may bloat your bundle size. Keeping your bundle size small helps reduce loading times and improves performance.
- Lazy Loading : Lazy load resources such as images, JavaScript and CSS to defer loading until they are needed. Next JS supports lazy loading out of the box that allowing you to dynamically load components, routes or other resources only when they are required and decrease initial page load times.
- Optimize Server-side Rendering (SSR) Data Fetching : Use efficient data fetching methods like caching, batching or optimizing database queries to reduce server response times and improve SSR performance. Avoid unnecessary data fetching operations and optimize database queries to make sure fast and efficient data retrieval.
- Cache Optimization : Implement caching strategies to cache static assets, API responses and other data to reduce server load and improve response times. Use caching mechanisms like HTTP caching, client-side caching or server-side caching to store and serve frequently accessed data from cache and reducing the need for repeated fetches and improving overall performance.
summery
In this articles we discuss about main questions for Next JS but after reading this complete article you can able to answer so many question . there is so many other question and answer you can find in this article
hope you like this content about