Understanding Processes and Threads in Node.jS
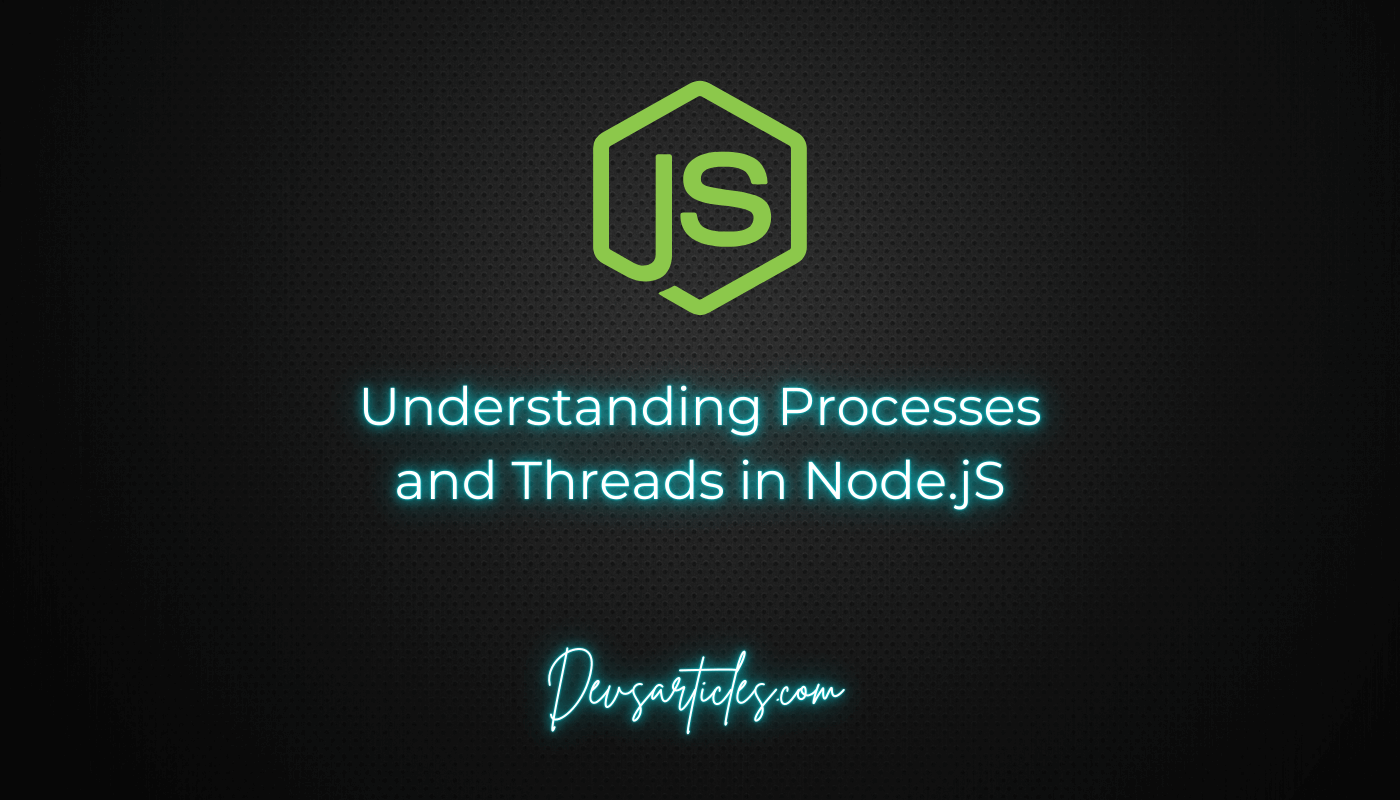
Node.js is a powerful, event-driven JavaScript runtime that has gained huge popularity for building scalable network applications. However, to fully achive its capabilities, it’s important to understand how NodeJS handles processes and threads. In this blog, we’ll dive deep into these concepts, explaining them in a way that anyone, regardless of their background, can easily grasp.
explore event driven concept in node.js
Table of Contents
What is a Process in Node.jS?
In computing, a process is an instance of a program that is being executed. It includes the program code, its current activity, and a set of resources like memory, file handles, and security attributes.
When you run a Node.js application, the operating system creates a new process for it. This process has its own memory space and is isolated from other processes. In Node.js, the process object is a global object that provides information about and control over the current process.
Key Points:
- Process: An instance of a running program.
- Isolation: Each process has its own memory space, isolated from other processes.
- Global Process Object: Node.js provides the process object to interact with the current process.
Threads: The Building Blocks of a Process
A thread is the smallest unit of execution within a process. Each process can contain multiple threads, which share the same memory space but can execute different parts of the code simultaneously. This is important for performing tasks like I/O operations without blocking the main execution.
Node.js and Single-Threaded Model:
Node.js is often described as single-threaded, which means it uses a single thread to execute JavaScript code. However, this doesn’t mean Node.js can’t handle multiple tasks simultaneously.
The Event Loop:
Node.js uses an event-driven, non-blocking I/O model that allows it to perform multiple operations simultaneously. The event loop is at the heart of this model, that allows Node.js to perform tasks asynchronously. When you perform an asynchronous operation like reading a file or making an HTTP request, Node.js doesn’t wait for the operation to complete. Instead, it continues executing other code and uses the event loop to check if the operation has completed, then triggers the corresponding callback.
Worker Threads:
Since Node.js v10.5.0, it introduced worker threads. Worker threads allow you to run JavaScript code in parallel on multiple threads, which is useful for CPU-intensive tasks that would otherwise block the event loop.
key Points:
- Thread: A unit of execution within a process.
- Single-Threaded: Node.js primarily runs on a single thread for executing JavaScript code.
- Event Loop: Manages asynchronous operations in a non-blocking manner.
- Worker Threads: Introduced to handle CPU-bound tasks without blocking the main thread.
Processes vs. Threads in Node.jS
Understanding the difference between processes and threads is important for designing efficient Node.js applications.
- Processes are independent and have their own memory space, while threads share the same memory space within a process.
- In Node.js, the main thread handles the execution of JavaScript code, while I/O operations are managed asynchronously by the event loop.
- For tasks requiring parallel execution, such as CPU-bound operations, worker threads or child processes can be used.
Key Points:
- Memory Isolation: Processes are isolated, whereas threads within a process share memory.
- Concurrency: Node.js uses an event loop to handle concurrency.
- Parallelism: Worker threads and child processes allow parallel execution.
Child Processes in Node.jS
While Node.js is single-threaded for executing JavaScript code, it can spawn child processes to handle tasks concurrently. Child processes are independent and can run in parallel with the main process.
The child_process module in Node.js provides methods to create child processes:
- spawn(): Starts a new process.
- exec(): Runs a command in a shell and buffers the output.
- fork(): Spawns a new Node.js process and establishes a communication channel with the parent.
Key Points:
- Child Processes: Independent processes that run in parallel.
- child_process Module: Provides methods like spawn(), exec(), and fork() to create and manage child processes.
Practical Use Cases
- Handling CPU-Intensive Tasks: For CPU-bound tasks like image processing or data encryption, using worker threads or child processes is ideal. This prevents the main thread from being blocked, this make sure that application remains responsive.
- Scalable Network Applications: For I/O-bound tasks like handling multiple HTTP requests, Node.js excels with its event-driven, non-blocking I/O model. The event loop make sure that the application can handle thousands of connections simultaneously without performance degradation.
- Real-Time Applications: Applications like chat servers or live data feeds benefit from Node.js’s ability to handle multiple concurrent connections efficiently, thanks to the event loop.
Key Points:
- Worker Threads/Child Processes: Ideal for CPU-bound tasks.
- Event Loop: Efficiently handles I/O-bound tasks, making Node.js suitable for scalable network applications.
- Real-Time Applications: Node.js is well-suited for real-time applications due to its non-blocking architecture.
Conclusion
Understanding processes and threads in Node.js is important for building scalable, efficient applications. While Node.js is single-threaded for executing JavaScript, its event-driven model allows it to handle multiple tasks simultaneously. For CPU-intensive tasks, worker threads and child processes provide the necessary parallelism without blocking the main thread.
By taking of the advantage of these concepts, you can design Node.js applications that are both performant and scalable, capable of handling a wide range of tasks from simple I/O operations to complex CPU-bound computations.
FAQs
What is the difference between a process and a thread in Node.jS?
A process in Node.js is an independent execution unit with its own memory space, while a thread is a smaller execution unit within a process that shares the same memory space with other threads. Node.js is single-threaded for executing JavaScript but can manage multiple processes using child processes.
Why is Node.jS called single-threaded if it can handle multiple tasks simultaneously?
Node.js is considered single-threaded because it uses a single thread to execute JavaScript code. However, it handles multiple tasks simultaneously using its event-driven, non-blocking I/O model and event loop, which allows it to manage I/O operations asynchronously.
How do I create a child process in Node.jS?
You can create a child process in Node.js using the child_process module, which provides methods like spawn(), exec(), and fork(). These methods allow you to start new processes, execute commands in a shell, or create a new Node.js process with a communication channel to the parent.