Axios Network Errors: Common Issues & Fixes | CORS, Timeouts, SSL Solutions
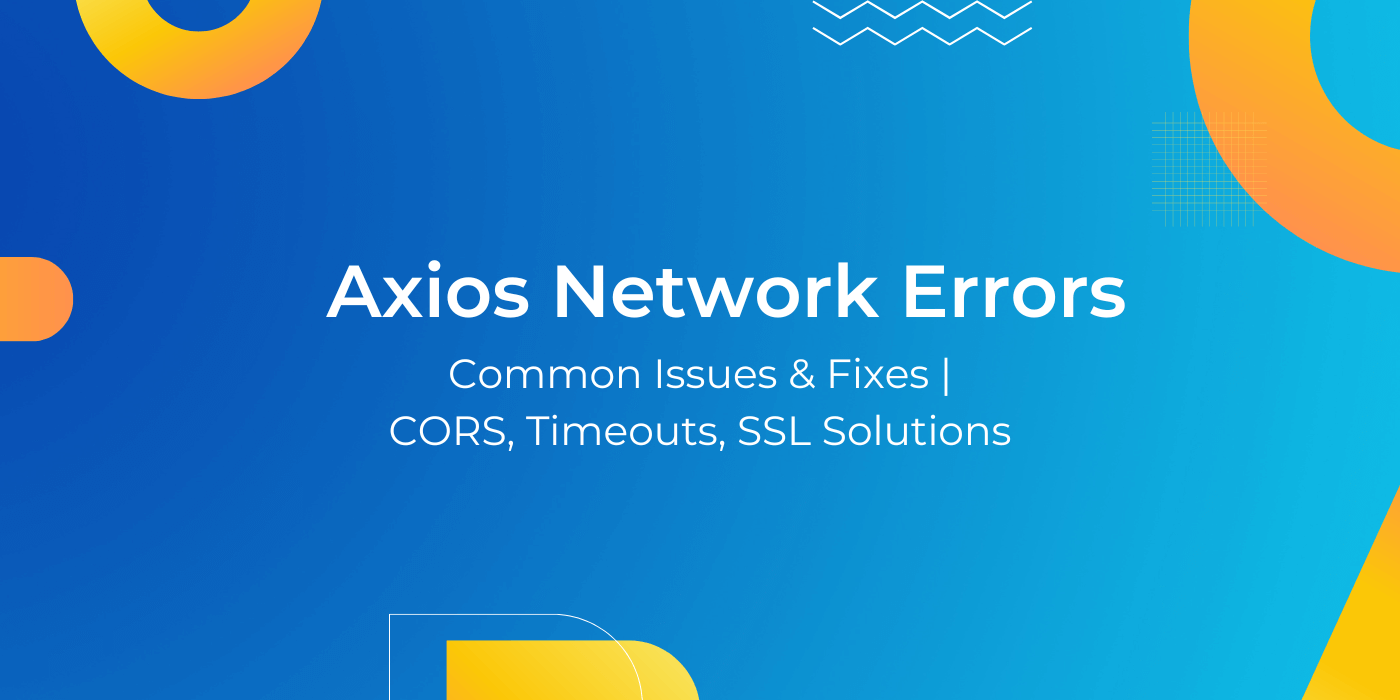
Introduction for Axios
Axios is a powerful JavaScript library for making HTTP requests, but vague “Network Error” messages can leave developers stuck. These errors often stem from server misconfigurations, client-side typos, or network restrictions. In this guide, we’ll decode the most common Axios network errors and provide actionable fixes to resolve them.
Table of Contents
1. CORS Errors: The Silent Request Blocker
What is CORS?
Cross-Origin Resource Sharing (CORS) is a security feature in browsers that blocks requests to external domains unless the server explicitly allows it. If your Axios request fails with a “Network Error” , CORS is often the culprit.
Server-Side Fix :
Add the Access-Control-Allow-Origin
header to your server’s response. For example:
// Node.js/Express example
app.use((req, res, next) => {
res.header("Access-Control-Allow-Origin", "*"); // Allow all domains
next();
});
Replace *
with your frontend URL (e.g., https://your-app.com
) in production
Test with Postman :
Use tools like Postman or curl
to confirm the server is reachable. If it works outside the browser, CORS is the issue.
Proxy Workaround :
Set up a proxy server (e.g., using Nginx or Cloudflare) to bypass CORS restrictions during development.
2. Invalid Base URL or Typos
Why It Happens
A typo in your API’s baseURL
(e.g., htp://api.com
instead of http://api.com
) or missing environment variables can break requests
Quick Fixes For Invalid Base URL or Typos
Double-check the baseURL in your Axios instance:
const api = axios.create({
baseURL: process.env.REACT_APP_API_URL, // Use environment variables
});
Use ping
or online tools like IsItDownRightNow to verify the server is online
3. SSL Certificate Errors
Common Scenarios
- Self-signed certificates in development.
- Expired SSL certificates on the server.
Solutions For SSL Certificate
Temporarily disable SSL verification (not recommended for production):
// React Native example
axios.get("https://your-api.com", {
httpsAgent: new https.Agent({ rejectUnauthorized: false }),
});
Use a trusted certificate from providers like Let’s Encrypt.
4. Timeout Errors
Slow servers or poor internet connections can cause Axios to abort requests.
How to Handle Timeouts
Set a Timeout :
axios.get("/data", { timeout: 5000 }) // 5-second timeout
.catch(error => console.log("Request timed out"));
Retry Failed Requests :Use the axios-retry library to auto-retry failed requests:
import axiosRetry from "axios-retry";
axiosRetry(axios, { retries: 3 });
Server-Side Errors (4xx/5xx)
- 4xx Errors :(e.g., 404 Not Found, 401 Unauthorized): Invalid endpoints, expired tokens, or missing permissions.
- 5xx Errors :(e.g., 500 Internal Server Error): Server crashes or misconfigurations.
How to Handle Them
Use Axios interceptors to catch errors globally and provide user feedback:
// axios-interceptors.js
axios.interceptors.response.use(
response => response,
error => {
if (error.response) {
// Server responded with 4xx/5xx
console.error(`Server Error: ${error.response.status}`);
alert(`Oops! ${error.response.statusText}`);
} else {
// Network error (e.g., CORS)
console.error("Network Error:", error.message);
}
return Promise.reject(error);
}
);
For deeper insights, check out our guide on Implementing Axios Interceptors.
6. React Native-Specific Fixes
React Native (Android 9+) blocks insecure HTTP requests by default. Fix this by adding:
<!-- android/app/src/main/AndroidManifest.xml -->
<application
android:usesCleartextTraffic="true"
... >
</application>
For iOS, update Info.plist
with:
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
Prevent man-in-the-middle attacks by pinning SSL certificates:
import { Network } from "react-native-network";
Network.setRequestInterceptors(
(config) => {
config.httpsAgent = new https.Agent({
ca: fs.readFileSync("path/to/cert.pem"),
});
return config;
},
(error) => Promise.reject(error)
);
Best Practices for Debugging
Use interceptors (as shown earlier) to log errors consistently. This helps track issues without repeating try/catch
blocks.
Check Network Connectivity
- Use browser DevTools (Network Tab ) to inspect failed requests.
- Test APIs with tools like Postman or Hoppscotch .
Avoid technical jargon in error alerts. Example:
if (error.code === "ERR_NETWORK") {
alert("Check your internet connection and try again.");
} else if (error.response?.status === 404) {
alert("The requested resource was not found.");
}
Conclusion
Axios network errors are frustrating but solvable. By mastering CORS fixes, leveraging interceptors, and following debugging best practices, you’ll build resilient apps.
FAQs
Why am I getting a “Network Error” in Axios even when the server is running?
This often happens due to CORS misconfigurations. Browsers block requests if the server doesn’t include the Access-Control-Allow-Origin header. Check your server logs to confirm the request is reaching the backend, and test the endpoint with Postman to rule out client-side issues.
Why does my Axios request time out?
Timeouts occur due to slow server responses or unstable networks. Set a timeout value in your Axios config (e.g., timeout: 5000 for 5 seconds) and implement retry logic using libraries like axios-retry.
Can I ignore CORS errors in Axios?
No, CORS is a browser security feature. Fix it by configuring the server to allow your domain or use a proxy during development.
How do I debug Axios errors effectively?
Use browser DevTools to inspect network requests, check server logs, and validate API endpoints with tools like Hoppscotch. Centralized error handling with interceptors also simplifies debugging.
2 Comments
Comments are closed.