Comprehensive Guide to Cryptography & Security in Node.jS: Protect Your Applications Like a Pro!
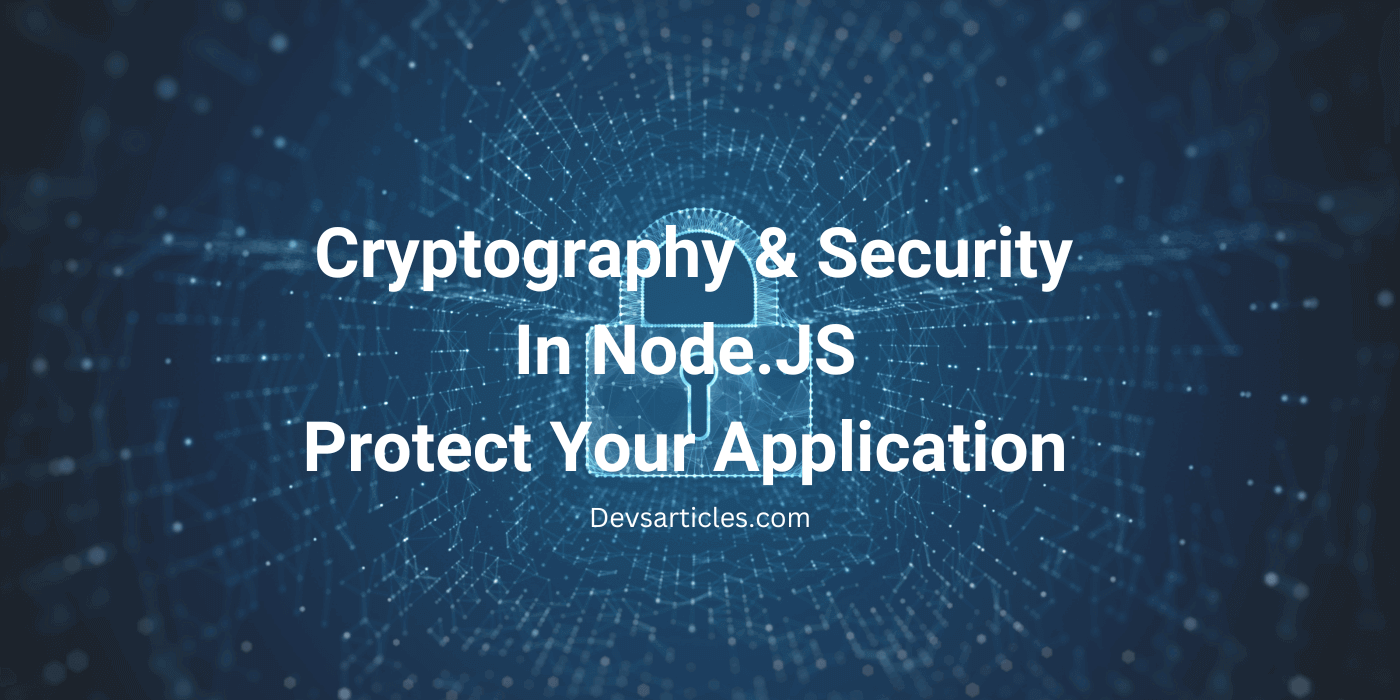
As more applications move online, security has become a critical priority for developers. Using Node.js for backend development is popular due to its speed and scalability, but securing a Node.js application requires a thoughtful approach. Here, we’ll explore how to use Node.js’s Cryptography & Security modules to keep your application and its data safe from modern threats. 🛡️
Table of Contents
🌟 Why Is Cryptography Important?
In simple terms, cryptography is the art of securing information, ensuring it’s only accessible to those intended. Cryptography enables:
- Data Privacy: Only authorized users can read the data.
- Authentication: Verifying that users are who they claim to be.
- Data Integrity: Ensuring data hasn’t been tampered with.
In Node.js, built-in security modules provide encryption, decryption, password hashing, and secure connections.
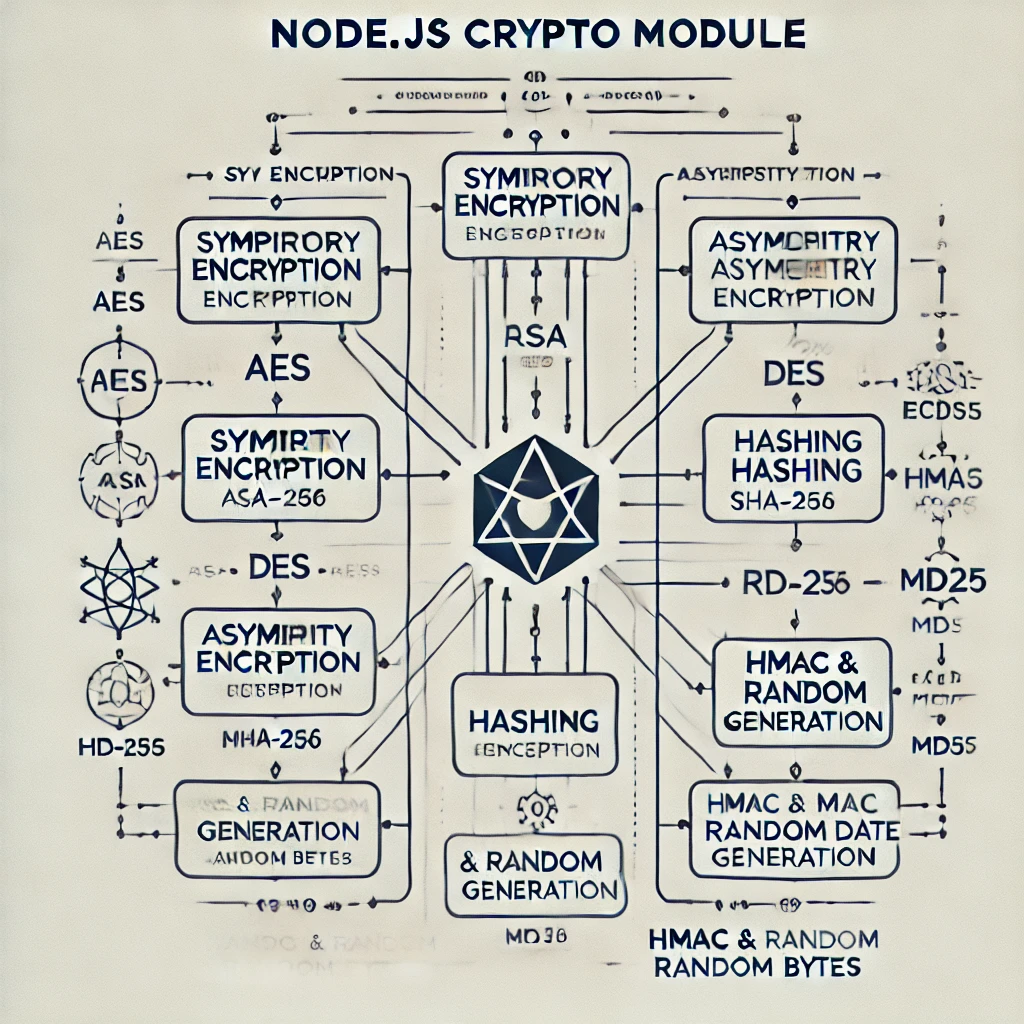
🔍 Node.js Security Modules: A Deep Dive
Here’s an overview of core Node.js modules and libraries focused on cryptography and security:
Module | Purpose | Key Uses |
---|---|---|
crypto | Encrypts, decrypts, and hashes data | Data security, user credentials |
tls / https | Manages SSL/TLS connections | Secure client-server connections |
bcrypt | Hashes passwords securely | User authentication |
helmet | Secures Express apps by modifying headers | Protection from common vulnerabilities |
jsonwebtoken (JWT) | Manages authentication tokens | Token-based authentication |
Understanding the crypto Module in Depth 🔒
The crypto module in Node.js is powerful, offering both symmetric (one-key) and asymmetric (two-key) encryption.
Hashing and Encryption Basics
- Hashing: Transforms data into a fixed-length hash, which is irreversible. Perfect for storing sensitive information, like passwords.
- Encryption: Turns readable data into an unreadable format and is reversible when using the correct key.
Example: Secure Hashing with crypto
Hashing allows you to store passwords securely by converting them into a “digest.” For example:
const crypto = require('crypto');
const password = 'superSecretPassword';
const hash = crypto.createHash('sha256').update(password).digest('hex');
console.log(hash); // Outputs a secure hash of the password
Symmetric Encryption Example
This example shows how symmetric encryption works, where both encryption and decryption require the same key.
const crypto = require('crypto');
const key = crypto.randomBytes(32);
const iv = crypto.randomBytes(16);
function encrypt(text) {
let cipher = crypto.createCipheriv('aes-256-cbc', key, iv);
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return { iv: iv.toString('hex'), encryptedData: encrypted };
}
function decrypt(encryptedData, iv) {
let decipher = crypto.createDecipheriv('aes-256-cbc', key, Buffer.from(iv, 'hex'));
let decrypted = decipher.update(encryptedData, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
🛠️ TLS/SSL for Encrypted Connections
TLS (Transport Layer Security) is a protocol for establishing encrypted links between a server and a client. It safeguards data in transit, making it essential for applications handling personal or sensitive information.
Setting up HTTPS with Node.js
To use HTTPS, you’ll need an SSL/TLS certificate:
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('path/to/private-key.pem'),
cert: fs.readFileSync('path/to/certificate.pem')
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end("Secure Connection Established!");
}).listen(443);
🔐 Password Hashing with bcrypt
One of the most widely used libraries for hashing passwords is bcrypt. It’s designed to be computationally slow, which protects against brute-force attacks.
Example of hashing a password with bcrypt:
const bcrypt = require('bcrypt');
const password = 'myPassword123';
const saltRounds = 10;
bcrypt.hash(password, saltRounds, (err, hash) => {
if (err) throw err;
console.log(hash); // Hashed password
});
Benefits of bcrypt:
- Salted Hashing: Adds a unique salt to each password, making it harder to crack.
- Computationally Intensive: Intentionally slow to defend against brute-force attacks.
🛡️ Securing Express Apps with helmet
Helmet is a middleware for Express.js that adds extra security headers to your HTTP responses. It helps protect against common vulnerabilities, including Cross-Site Scripting (XSS) and Clickjacking.
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet()); // 🛡️ Adds security headers to all responses
app.listen(3000, () => console.log("Server running securely!"));
Key Features of Helmet:
- Content Security Policy: Prevents unwanted scripts from running on your website.
- X-Frame-Options: Prevents clickjacking by restricting iframe embedding.
⚖️ Pros and Cons of Node.js Security Modules
Module | Advantages | Disadvantages |
---|---|---|
crypto | Native to Node.js, extensive cryptographic functions | Complex to configure for beginners |
tls / https | Essential for data in transit | Requires setup and maintenance of certificates |
bcrypt | Highly secure for password hashing | Slower due to encryption complexity |
helmet | Easy to use, boosts security with minimal setup | Limited to Express |
jsonwebtoken (JWT) | Provides token-based authentication | Tokens can be stolen if not securely stored |
💡 Best Practices for Node.js Security
- Use HTTPS: Always use HTTPS in production to secure data during transmission.
- Environment Variables: Store sensitive keys and configurations in environment variables.
- Regularly Update Dependencies: Avoid vulnerabilities by keeping packages up-to-date.
- Implement Rate Limiting: Prevent brute-force attacks by setting request limits.
- Use Secure Authentication: Opt for token-based authentication (e.g., JWT) for stateless, scalable security.
Conclusion: Securing Your Node.js Application 🌐
Understanding and implementing cryptography and security modules in Node.js isn’t optional anymore—it’s a necessity. With crypto, tls, bcrypt, helmet, and other essential tools, you can fortify your applications against a wide range of cyber threats. By integrating these modules and following best practices, you’ll ensure your application is robust, reliable, and secure. 🚀
FAQs
What is the difference between hashing and encryption?
Hashing is one-way and ideal for data like passwords. Encryption is reversible and perfect for secure data transmission.
Is bcrypt better than SHA algorithms?
Yes, bcrypt is designed to be slower, making it more secure against brute-force attacks, unlike faster hashing algorithms like SHA.
Why should I use Helmet in my Express app?
Helmet automatically sets HTTP headers that help prevent well-known security vulnerabilities, making it easier to secure Express applications.
How often should I update my dependencies?
Regularly, especially if you see vulnerabilities reported in libraries. Using npm audit can help you identify and update at-risk packages.
Can I use JWT alone for securing my app?
JWT is ideal for stateless authentication, but make sure to secure token storage and use HTTPS to prevent token theft.
Why is HTTPS important for my application?
HTTPS secures data between the client and server, preventing eavesdropping and tampering. It’s essential for applications handling sensitive information.