Understanding the “Objects are not valid as a React child” Error in React
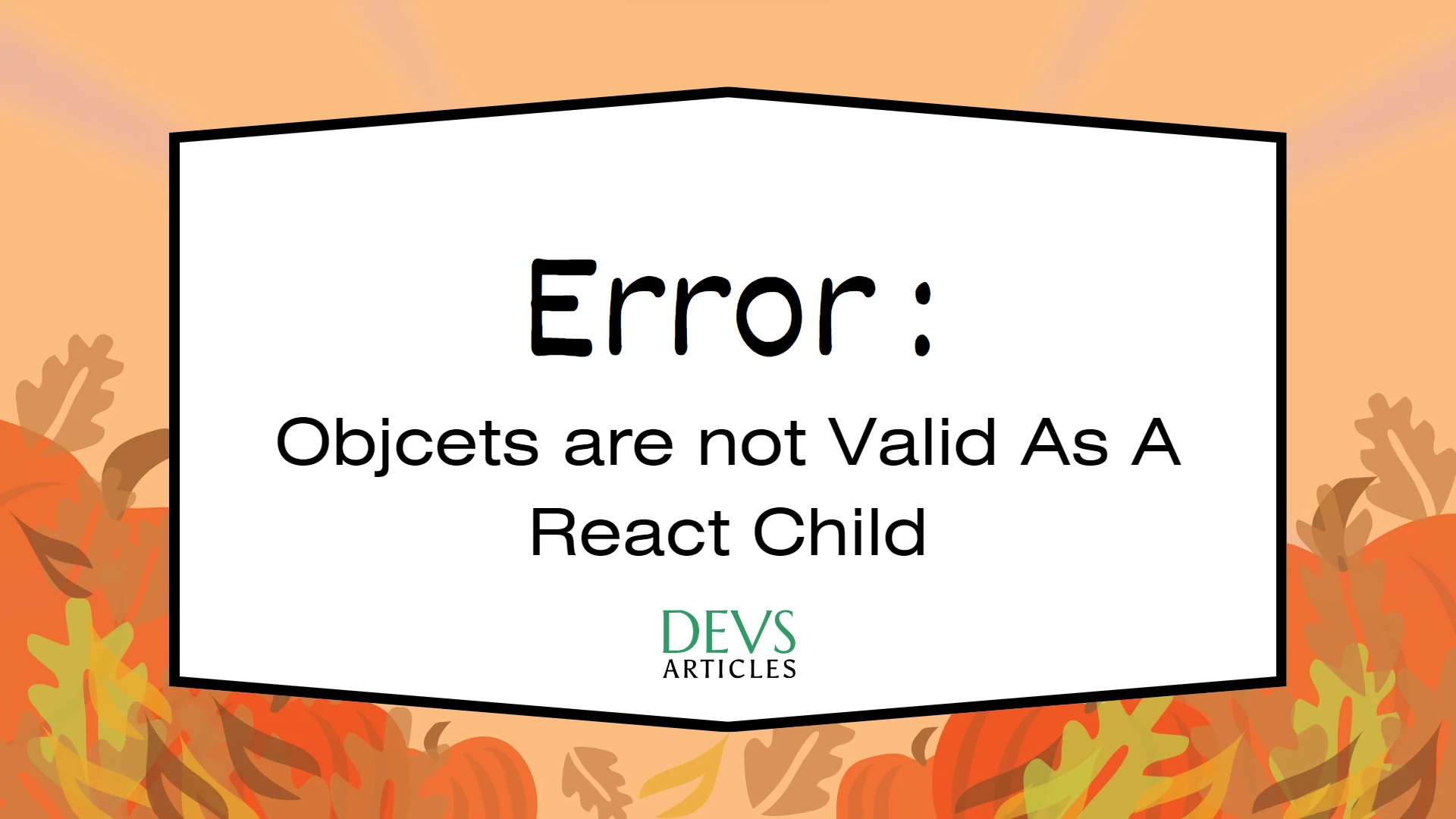
React is a one of the JavaScript library for building user interfaces. React is a component based architecture with this developers to create reusable and modular UI elements. when working with React you may face the “Objects are not valid as a React child” error. In this blog post we will explore the common scenarios leading to this error and discuss how to troubleshoot and resolve it.
Table of Contents
Why objects are not valid as a react child error occurs ?
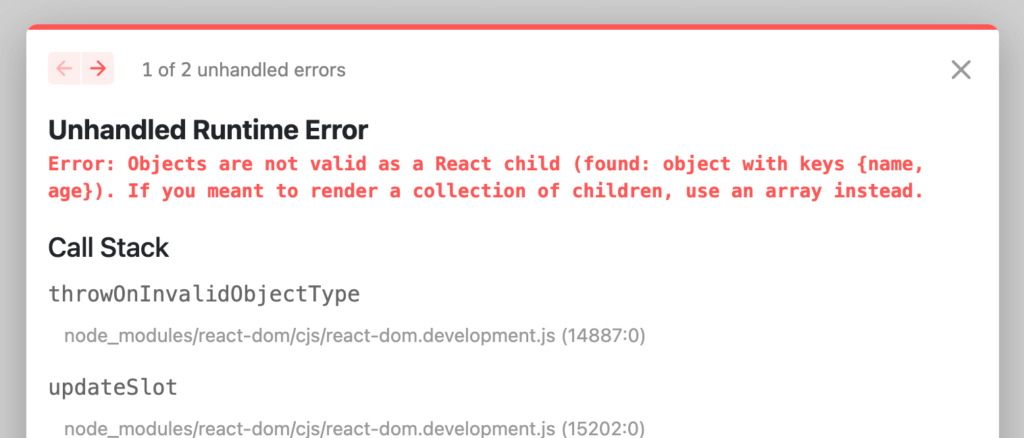
When we try to render an object directly
The “Objects are not valid as a React child” error in React typically occurs when you try to render an object directly as a child component or within JSX. React expects child elements to be valid JSX expressions such as strings, numbers or React components but not plain JavaScript objects.
Here’s an example of what might trigger this error:
const myObject = { key: 'value' };
const MyComponent = () => {
return (
<div>
{myObject} {/* This will cause the error */}
</div>
);
};
In this code {myObject}
is trying to render an object directly. Which React doesn’t allow. To solve this issue you need to ensure that you’re rendering valid JSX within the curly braces. You might want to access specific properties of the object or convert it to a string or another suitable format for rendering.
For Solving this Error
const myObject = { key: 'value' };
const MyComponent = () => {
return (
<div>
{myObject.key} {/* Accessing a specific property of the object */}
</div>
);
};
if you want to render the entire object as a string:
const myObject = { key: 'value' };
const MyComponent = () => {
return (
<div>
{JSON.stringify(myObject)} {/* Converting the object to a string */}
</div>
);
};
make sure that the content you are rendering within JSX curly braces is a valid React child element. If you still encounter issues then double-check your JSX code and ensure that you are not trying to render an object directly.
when we try to using object is in array mapping
When dealing with array mapping in React and trying to render objects directly without handling them appropriately. You might encounter error “objects are not valid as a react child. Here’s a list format with code blocks explaining this:
const myArray = [{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }];
// This will cause the error
{myArray.map(item => <div key={item.id}>{item}</div>)}
Mapping an array of objects directly without handling them properly can result in the “Objects are not valid as a React child” error.
const myArray = [{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }];
// Rendering specific properties
{myArray.map(item => <div key={item.id}>{item.name}</div>)}
// Converting the object to a string
{myArray.map(item => <div key={item.id}>{JSON.stringify(item)}</div>)}
- Map through the array and render specific properties of each object.
- Convert the object to a suitable format for rendering like a string.
some methods for debug
check your render method
why Check render methods ?
- Render Method Purpose: The render method is a fundamental part of a React component. It decide what gets displayed on the UI when the component is rendered.
- Hierarchy of Components: In React app components are often nested within each other to create a component hierarchy. The render method of each component contributes to the final UI.
how to check render method ?
- Review Component Structure: Start by examining the structure of your component. Look at the JSX code within the render method to understand how your UI is being constructed.
- Identify Where the Error Occurs: Locate the specific line where you are attempting to render content. Pay close attention to any variables or data being used.
conclusion
The “Objects are not valid as a React child” error is a common Error in React development. By understanding the underlying causes and following best practices. you can easily resolve this issue and build robust and error-free React applications.
FAQs
What does the error “Objects are not valid as a React child” mean in React?
This error occurs when attempting to render an object directly within JSX or as a child component. React expects child elements to be valid JSX expressions like strings, numbers or other React components not plain JavaScript objects.
How can I debug the “Objects are not valid as a React child” error?
Review the component structure and JSX code within the render method. Identify where the error occurs paying attention to variables or data being used. Use console.log statements or debugging tools to log data and variables within the render method.