Supabase and React: mastering Complete Fullstack Development
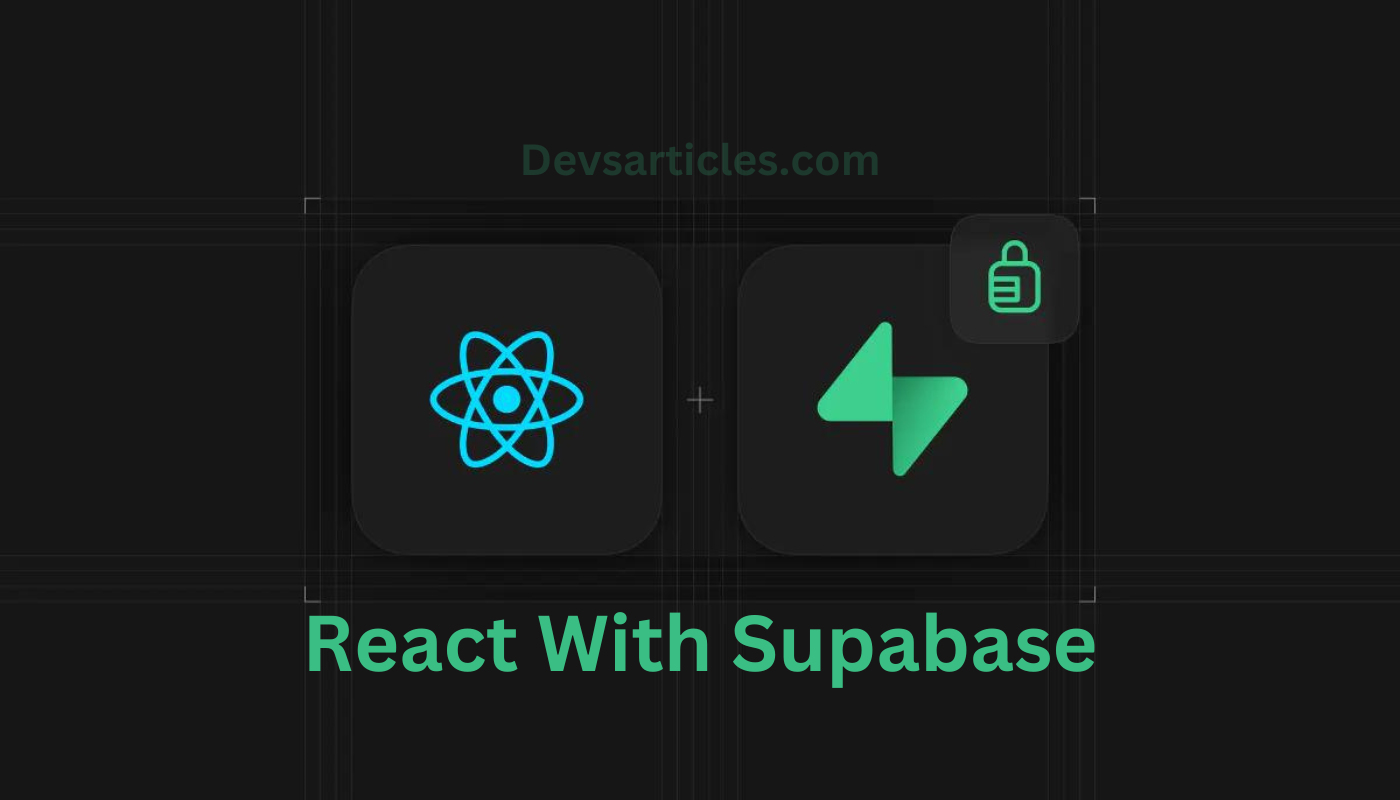
Introductions
In the ever-evolving world of web development, developers are constantly seeking efficient solutions to streamline their workflow and deliver high-quality applications rapidly. Enter Supabase, an open-source Firebase alternative that offers a wide suite of tools for building modern web and mobile applications. When combined with the powerful React framework, Supabase gives power to developers to create seamless and efficient fullstack applications with ease.
Table of Contents
What is Supabase?
Supabase is an open-source project that aims to provide a complete backend-as-a-service (BaaS) solution for developers. It combines various open-source tools, such as PostgreSQL for the database, Realtime for listening to changes, and Storage for managing user-uploaded files, into a single platform.

One of the key advantages of Supabase is its compatibility with existing tools and workflows. It can be seamlessly integrated into projects built with popular frameworks like React, Vue.js, and Angular, as well as backend languages like Node.js, Python, and Ruby.
Supabase offers a wide range of features that simplify backend development, including:
- PostgreSQL Database: Supabase provides a robust and scalable PostgreSQL database, allowing you to store and query data efficiently. It supports features like row-level security, partial/full-text search, and advanced data types.
- Auth: Supabase includes a built-in authentication system that supports email/password authentication, as well as integrations with popular providers like GitHub, Google, and Magic Link.
- Realtime: With Supabase’s Realtime functionality, you can subscribe to database changes and receive updates in real-time, allowing you to build responsive and collaborative applications.
- Storage: Supabase offers secure object storage for user-uploaded files, such as images, videos, and documents. It integrates seamlessly with the database, making it easy to manage and retrieve files.
- Edge Functions: Supabase supports serverless functions, allowing you to run custom code on the edge, closer to your users. This can be useful for tasks like data transformations, webhooks, and custom business logic.
- APIs: Supabase provides auto-generated APIs for your database, allowing you to interact with your data using RESTful or GraphQL interfaces.
- Pricing: Supabase offers a generous free tier, making it accessible for developers to build and launch projects without incurring costs. Paid tiers are available for larger-scale applications and additional features.
By combining these features into a single platform, Supabase aims to simplify backend development and reduce the need for complex infrastructure setup and maintenance. Developers can focus on building their applications while taking advantage of Supabase’s scalable and secure backend services.
Getting Started with Supabase and React
Before we dive into the specifics of building our application, let’s set up our development environment by following these steps:
Sign up for supabase and create new project
Head over to the Supabase website and create a new account or log in if you already have one. Now, Once logged in, navigate to the Supabase Dashboard and create a new project by clicking the “New Project” button.
install supabase CLI
Open your terminal and run the following command to install the Supabase CLI globally
npm install -g @supabase/cli
Set up React project and install supabase client
Create a new React project using your preferred method (e.g., Create React App, Vite, or Next.js). Once created project, in your React project directory, run the following command to install the Supabase client library:
npm install @supabase/supabase-js
Now that our development environment is set up, let’s start building our application!
create a simple todo application using React and Supabase
In this section, we’ll create a simple todo application using React and Supabase. We’ll cover the following steps:
Initialize Supabase client
Import the Supabase client library in your React component and initialize it with your Supabase project URL and an anonymous key (available in your Supabase project settings).
import { createClient } from '@supabase/supabase-js'
const supabaseUrl = 'YOUR_SUPABASE_URL'
const supabaseKey = 'YOUR_SUPABASE_ANON_KEY'
const supabase = createClient(supabaseUrl, supabaseKey)
Create a todo table
Navigate to the Supabase Dashboard, click on the “Table Editor,” and create a new table called “todos” with the following columns: id (auto-incremented UUID), task (text), and is_complete (boolean).
Fetch todos from Supabase
In your React component, create a function to fetch todos from the Supabase database using the supabase.from('todos').select()
method.
const fetchTodos = async () => {
let { data: todos, error } = await supabase
.from('todos')
.select('*')
if (error) console.log('error', error)
else setTodos(todos)
}
Add a new todo
Implement a function to add a new todo to the Supabase database using the supabase.from('todos').insert()
method.
const addTodo = async (taskText) => {
let task = taskText.trim()
if (task.length) {
let { data: todo, error } = await supabase
.from('todos')
.insert({ task, is_complete: false })
if (error) console.log('error', error)
else setTodos([...todos, todo[0]])
}
}
Update a todo
Create a function to update the is_complete status of a todo in the Supabase database using the supabase.from('todos').update()
method.
const toggleCompleted = async (id, is_complete) => {
let { data: todo, error } = await supabase
.from('todos')
.update({ is_complete: !is_complete })
.eq('id', id)
if (error) console.log('error', error)
else setTodos(todos.map(t => (t.id === id ? { ...t, is_complete: !t.is_complete } : t)))
}
Delete a todo
Implement a function to delete a todo from the Supabase database using the supabase.from('todos').delete()
method.
const deleteTodo = async (id) => {
let { data: todo, error } = await supabase
.from('todos')
.delete()
.eq('id', id)
if (error) console.log('error', error)
else setTodos(todos.filter(t => t.id !== id))
}
Render the todo list
In your React component’s JSX, map over the todos array and render each todo as a list item, displaying its task and completion status. Provide buttons or checkboxes to toggle the completion status and delete the todo.
return (
<div>
<h1>Todo List</h1>
<input
type="text"
placeholder="Add a new todo"
value={newTaskText}
onChange={(e) => setNewTaskText(e.target.value)}
onKeyDown={(e) => {
if (e.key === 'Enter') {
addTodo(newTaskText)
setNewTaskText('')
}
}}
/>
<ul>
{todos.map(todo => (
<li key={todo.id}>
<input
type="checkbox"
checked={todo.is_complete}
onChange={() => toggleCompleted(todo.id, todo.is_complete)}
/>
<span style={{ textDecoration: todo.is_complete ? 'line-through' : 'none' }}>
{todo.task}
</span>
<button onClick={() => deleteTodo(todo.id)}>Delete</button>
</li>
))}
</ul>
</div>
)
That’s it! You now have a fully functional todo application built with React and Supabase. You can further improve this application by adding authentication, real-time updates, and more advanced features provided by Supabase.
Conclusion
- Supabase Documentation: Comprehensive documentation on Supabase’s features and usage.
Building applications with Supabase and React offers a seamless and efficient development experience, allowing you to focus on creating high-quality user experiences without worrying about complex backend setups. By leveraging the power of these technologies, you can streamline your development process and deliver modern, scalable applications quickly and efficiently.
Supabase is an open-source project, which means that the community can contribute to its development, report issues, and suggest new features. This collaborative approach make sure that Supabase continues to grow and meet the needs of developers.
With its comprehensive set of features and open-source nature, Supabase has become a popular choice for developers building modern web and mobile applications, particularly those leveraging React, Vue.js, and other popular frontend frameworks.
FAQs
What is Supabase, and how does it differ from Firebase?
Supabase is an open-source Firebase alternative that provides a suite of backend tools for building modern web and mobile applications. Unlike Firebase, which is a proprietary service, Supabase is built on open-source technologies like PostgreSQL, making it more customizable and cost-effective for developers.
Is Supabase only compatible with React, or can it be used with other frameworks?
Supabase is framework-agnostic, which means it can be integrated with various frontend frameworks and libraries, including React, Vue.js, Angular, and even vanilla JavaScript. Its client libraries and APIs are designed to work seamlessly across different tech stacks.
How does Supabase handle authentication and authorization?
Supabase provides a built-in authentication system that supports email/password authentication, as well as integrations with popular providers like GitHub, Google, and Magic Link. It also offers row-level security features that allow you to control access to your data based on user roles and permissions.