REST API vs GraphQL: An In-Depth Comparison for Modern Web Development
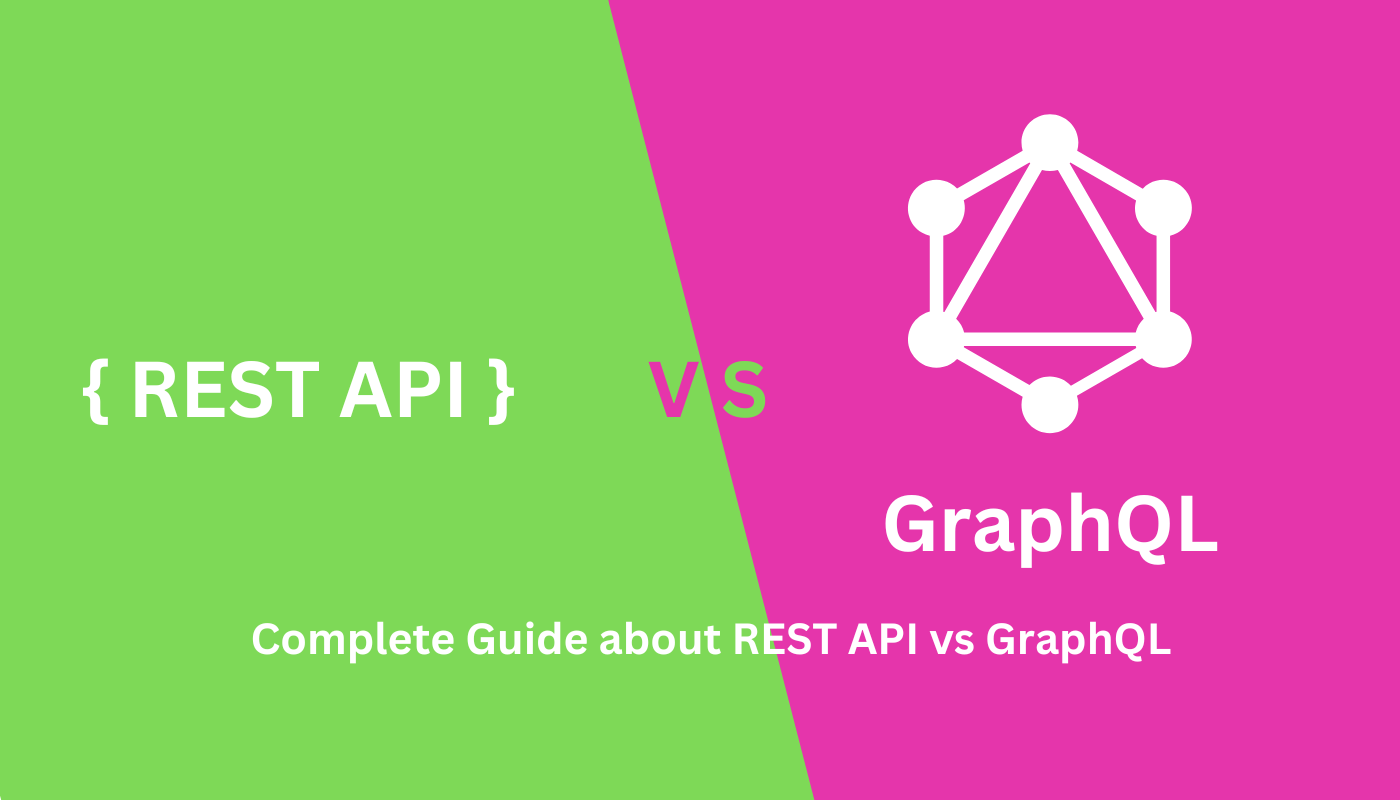
Introduction
In the world of web development, two dominant approaches have appeared for building APIs (Application Programming Interfaces): REST API and GraphQL. Both architectures aim to facilitate efficient data transfer between clients and servers, but they differ significantly in their design principles and implementation details. In this comprehensive blog post, we’ll dive deep into the complexities of each approach, exploring their advantages, disadvantages, and practical use cases.
Table of Contents
What is REST API?
REST (Representational State Transfer) is an architectural style for building web services that rely on HTTP requests to transfer data between client and server. In a REST API, each resource (such as a user, post, or comment) is identified by a unique URI (Uniform Resource Identifier), and clients interact with these resources using HTTP methods like GET, POST, PUT, and DELETE.
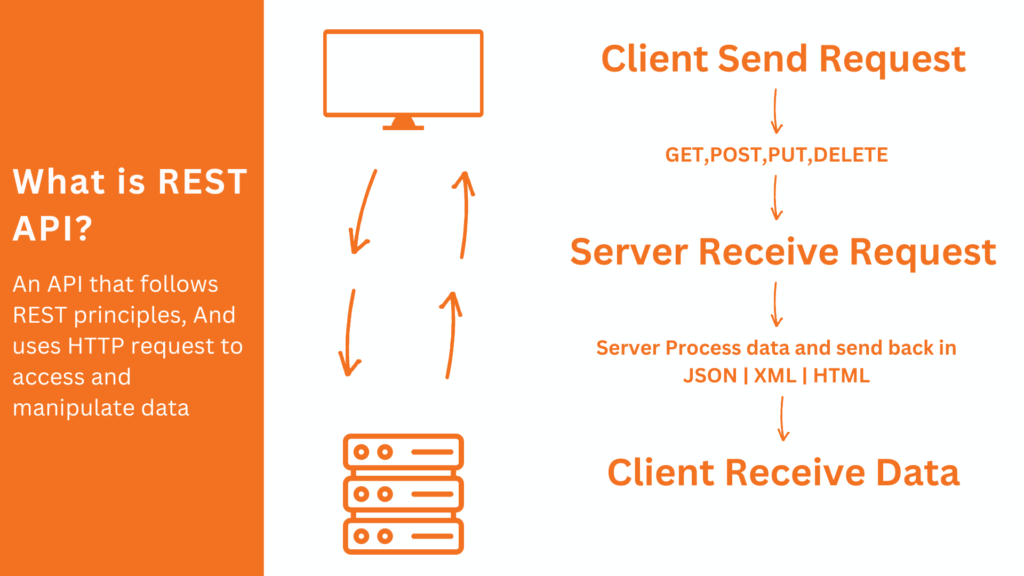
REST API Principles
- Client-Server Architecture: The client and server are separate entities, allowing for their independent development and scaling.
- Stateless: Each request contains all the necessary information, making it easier to scale and manage server resources.
- Cacheable: REST APIs can leverage HTTP caching mechanisms, improving performance and reducing server load.
- Layered System: Intermediates, such as load balancers or proxy servers, can be introduced without affecting the client or server.
- Code on Demand (Optional): Servers can temporarily extend or customize the client’s functionality by transferring executable code.
Advantages and Disadvantages of REST API
Advantages of REST API
- Simple and lightweight: REST APIs use plain text formats like JSON or XML for data transfer, making them easy to understand and work with.
- Caching: REST APIs can leverage HTTP caching mechanisms, improving performance and reducing server load.
- Wide adoption and compatibility: REST APIs are widely adopted and compatible with various programming languages and frameworks.
- Scalability: REST APIs are designed to be scalable, making them suitable for large-scale applications.
Disadvantages of REST API
- Over-fetching and under-fetching: Clients often need to make multiple requests to fetch related data, leading to over-fetching (retrieving more data than needed) or under-fetching (requiring additional requests for missing data).
- Lack of flexibility: REST APIs follow a fixed data structure, making it challenging to adapt to changing client requirements.
- Versioning complexity: Maintaining multiple versions of a REST API can become unmanageable as the API evolves.
- Limited query capabilities: REST APIs have limited query capabilities, making it difficult to perform complex data filtering or transformation on the server side.
What is GraphQL?
GraphQL is an open-source data query language and runtime developed by Facebook. Instead of defining fixed endpoints, GraphQL allows clients to describe the data they need using a query language. The server then responds with exactly the requested data, eliminating over-fetching and under-fetching issues.
query {
user(id: 1) {
name
email
posts {
title
content
}
}
}
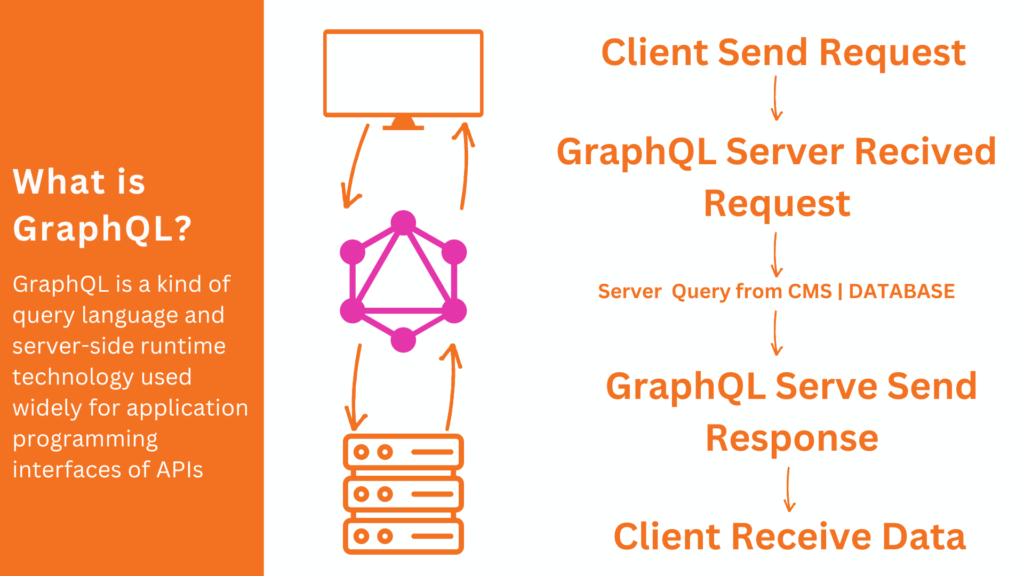
GraphQL Principles
- Single Endpoint: GraphQL uses a single endpoint for all queries, simplifying API versioning and maintenance.
- Hierarchical Data Fetching: Clients can specify the data they need, including related resources, in a single query.
- Strong Typing: GraphQL uses a strongly typed schema, which helps catch errors during development and improves code documentation.
- Introspection: GraphQL APIs are self-documenting, allowing clients to query the schema and understand the available data and types.
Advantages and Disadvantages of GraphQL
Advantages of GraphQL
- Flexible data fetching: Clients can specify exactly what data they need, reducing over-fetching and under-fetching issues.
- Single endpoint: GraphQL uses a single endpoint for all queries, simplifying API versioning and maintenance.
- Strong typing: GraphQL uses a strongly typed schema, which helps catch errors during development and improves code documentation.
- Efficient data transfer: GraphQL minimizes the amount of data transferred over the network by allowing clients to request only the required data.
- Evolving APIs: GraphQL APIs can grow more easily without breaking existing clients, thanks to its strong typing and introspection capabilities.
Disadvantages of GraphQL
- Steep learning curve: GraphQL has a steeper learning curve compared to REST APIs, especially for developers new to the concept of query languages.
- Caching complexity: Caching GraphQL responses can be more complex due to the dynamic nature of queries.
- Server-side complexity: Implementing a GraphQL server requires more code and complexity than a simple REST API.
- Limited browser support: GraphQL queries cannot be executed directly in the browser due to security restrictions, requiring additional client-side code or proxy servers.
When to Use REST API vs GraphQL?
The choice between REST API and GraphQL depends on your project’s requirements and the trade-offs you’re ready to make. Here are some general guidelines:
Use Rest API if:
- Your API is relatively simple and has well-defined data structures.
- You need to support a wide range of clients, including legacy systems or browsers with limited capabilities.
- You have strict requirements for caching and can take advantage of existing HTTP caching mechanisms.
- Your team is already familiar with REST principles and doesn’t want to adopt a new paradigm.
use GraphQL if:
- Your API needs to handle complex data relationships and varying client requirements.
- You want to reduce over-fetching and under-fetching issues, improving performance and bandwidth usage.
- You prioritize developer productivity and are ready to invest time in learning and implementing GraphQL.
- Your application requires real-time updates or subscriptions to data changes.
- You need to develop your API without breaking existing clients.
Real World Example
REST APIs are widely used in various domains, including e-commerce platforms (like Amazon), social media applications (like Twitter), and cloud services (like AWS). They are particularly suitable for scenarios where data structures are well-defined and client requirements are relatively stable.
On the other hand, GraphQL has gained popularity in modern web and mobile applications with complex data requirements, such as Facebook, GitHub, and Shopify. It shines in scenarios where client requirements are diverse, and data relationships are intricate.
Aspect | REST API | GraphQL |
Architecture | Multiple endpoints, each representing a resource | Single endpoint for all queries |
Data Fetching | Fixed data structure, clients may over-fetch or under-fetch data | Flexible data fetching, clients specify exactly what data they need |
Query Language | Uses HTTP methods (GET, POST, PUT, DELETE) | Uses a query language to describe data requirements |
Caching | Can leverage HTTP caching mechanisms | Caching can be more complex due to dynamic queries |
Versioning | Maintaining multiple versions can be cumbersome | Easier to evolve without breaking existing clients |
Typing | Typically uses loosely-typed data formats (JSON, XML) | Uses a strongly-typed schema |
Documentation | Documentation is separate from the API | Self-documenting through introspection |
Learning Curve | Relatively simple, based on well-established REST principles | Steeper learning curve due to query language concept |
Performance | Can lead to over-fetching or under-fetching, impacting performance | Efficient data transfer by requesting only required data |
Use Cases | Suitable for simple APIs with well-defined data structures | Ideal for complex data requirements and varying client needs |
This table provides a high-level overview of the key differences between REST API and GraphQL in terms of their architecture, data fetching mechanisms, caching, versioning, typing, documentation, learning curve, performance, and typical use cases. It can help developers quickly compare and contrast the two approaches, aiding in the decision-making process for their specific project requirements.
Conclusion
In conclusion, both REST API and GraphQL have their strengths and weaknesses. REST APIs are simpler and more widely adopted, while GraphQL offers more flexibility and efficiency for handling complex data requirements. Ultimately, the choice depends on your project’s specific needs, team expertise, and willingness to adopt new technologies. In some cases, a hybrid approach combining the strengths of both architectures may be the optimal solution.
Checkout Best practice for NodeJS API
FAQs
Is GraphQL replacing REST API entirely?
Not necessarily. While GraphQL offers many advantages over REST API in certain scenarios, REST API still has its place, especially in projects with simpler data structures and where compatibility with a wide range of clients is important. The decision to use GraphQL or REST API depends on the specific requirements of each project.
How does GraphQL handle security compared to REST API?
GraphQL and REST API handle security in similar ways, typically through authentication and authorization mechanisms such as JWT (JSON Web Tokens) or OAuth. Both architectures require careful consideration and implementation of security measures to protect against common vulnerabilities like CSRF (Cross-Site Request Forgery) and XSS (Cross-Site Scripting).
Can I use GraphQL with existing REST APIs?
Yes, you can use GraphQL alongside existing REST APIs. Many developers adopt an incremental migration approach, where they start by implementing GraphQL for specific features or data requirements while maintaining the existing REST API for the rest of the application. This allows for a smooth transition without disrupting the entire system.
Which one is more suitable for real-time updates: REST API or GraphQL?
GraphQL is better suited for real-time updates due to its ability to handle subscriptions, which allow clients to receive real-time notifications about changes to data. While it’s possible to implement real-time features with REST API using technologies like WebSockets, GraphQL offers a more straightforward and integrated solution.