The Ultimate Guide to Node.js: Modules, Features, Pros & Cons, and More

Welcome to the ultimate guide on Node.js! 🌐 In this comprehensive blog, we’ll explore deep that what makes Node.js special, explore its core modules, compare it with other languages, and discuss its strengths and limitations. Whether you’re a beginner or an experienced developer, this guide will serve as your go-to reference for understanding Node.js inside and out.
Table of Contents
What is Node.js?
Node.js is an open-source, server-side JavaScript runtime built on Chrome’s V8 JavaScript engine. It was created to allow developers to run JavaScript code outside the browser, enabling them to build fast, scalable server applications with a single language across the full stack.
Unlike traditional server environments, which often require complex server setups, Node.js uses a lightweight, event-driven, non-blocking I/O model. This makes it ideal for building real-time applications where speed and responsiveness are important, such as chat applications, live streaming, and collaborative tools.
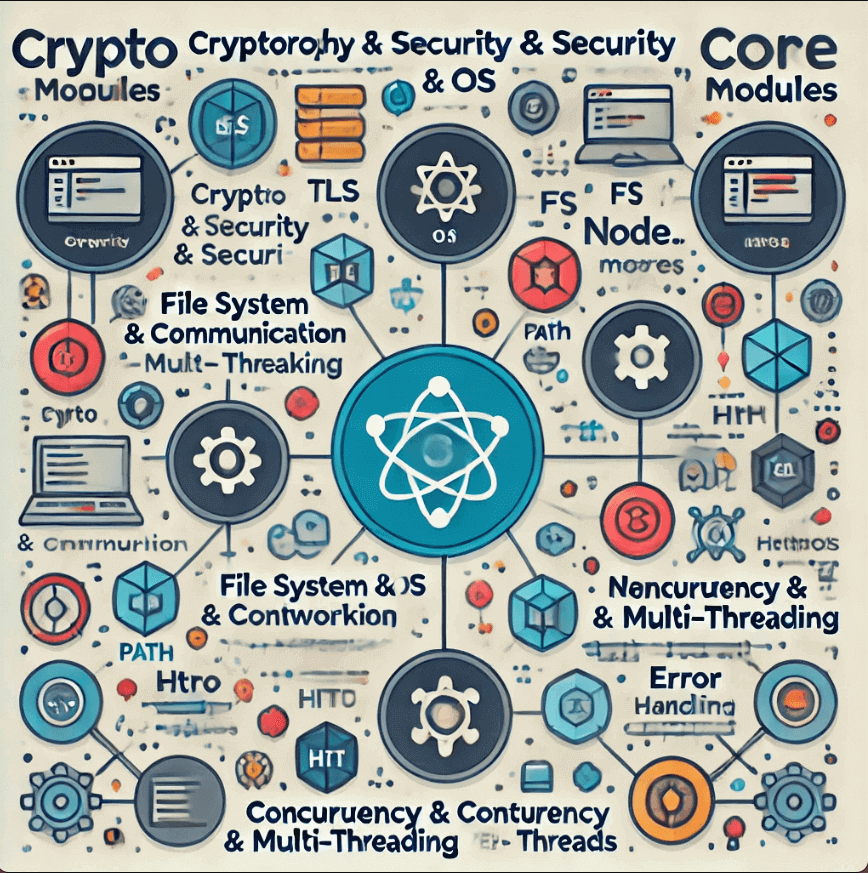
Key Features of Node.js
🚀 Let’s look at some defining features that make Node.js unique and powerful:
- Single-Threaded, Asynchronous I/O: Node.js operates on a single thread using non-blocking I/O, allowing it to handle multiple concurrent requests efficiently. This model suits real-time, high-concurrency applications.
- Event-Driven Architecture: Using an event loop, Node.js efficiently handles I/O operations without blocking the main thread. Events like HTTP requests or data updates trigger functions asynchronously, enabling smooth and fast processing.
- Rich Ecosystem with npm: The Node Package Manager (npm) hosts thousands of reusable packages, modules, and libraries. You can quickly add functionalities ranging from database access to authentication to your project with just a few commands.
- Cross-Platform Compatibility: Node.js runs on Windows, macOS, and Linux, making it highly versatile and accessible across different environments.
- Full Stack Development: With Node.js, you can use JavaScript on both the frontend and backend, simplifying development and maintenance.
Pros and Cons of Node.js
✅ Pros of Node.js
- High Performance with V8 Engine: Powered by Google’s V8 engine, Node.js converts JavaScript code into fast, optimized machine code. This boosts performance and efficiency, especially for I/O-heavy tasks.
- Scalability: Thanks to its asynchronous, non-blocking nature, Node.js can handle a large number of simultaneous requests, making it highly scalable for microservices and cloud-native applications.
- Single Language for the Entire Stack: JavaScript on both the client and server sides means developers can work across the stack without switching languages.
- Rich Package Ecosystem (npm): npm has over a million packages, making it easy to add almost any functionality to a Node.js project with minimal configuration.
- Community Support: With an active and growing community, finding solutions, tutorials, and resources for Node.js is straightforward, and new improvements are regularly contributed.
❌ Cons of Node.js
- Single-Threaded Bottlenecks: While Node.js can handle multiple requests, CPU-bound tasks (e.g., heavy computations) can bottleneck its single-threaded model, impacting performance.
- Callback Hell: Heavy reliance on callbacks, while useful for asynchronous code, can lead to complicated, hard-to-read code structures. This is somewhat mitigated by async/await, but the issue still arises in some cases.
- Not Ideal for CPU-Intensive Tasks: Node.js is better suited to I/O-intensive applications. Applications that require significant computation or processing are better off with languages like Python or C++.
- Maturity of npm Modules: While npm offers a vast library, not all modules are well-maintained or secure, requiring developers to carefully vet dependencies.
Node.js Compared to Other Languages
🔄 To understand Node.js’s place in the development world, here’s a table comparing its strengths and weaknesses with other popular backend technologies:
Feature | Node.js | Python | Java | PHP |
---|---|---|---|---|
Concurrency Model | Asynchronous, event-driven | Synchronous, threads | Multithreaded | Synchronous |
Best for | Real-time applications,Web development | Data science, AI | Enterprise apps | Web development |
Speed | Very fast for I/O tasks | Moderate | High | Moderate |
Ease of Learning | Easy (JavaScript-based) | Easy to moderate | Moderate | Easy |
Community | Large, active | Very large | Large, enterprise-focused | Large |
Scalability | High | Moderate | Very high | Moderate |
Special Features of Node.js
- Streaming and Buffering: Node.js handles streams efficiently, making it a strong choice for media and data streaming applications. You can manage streaming I/O operations (such as processing data chunk-by-chunk) rather than loading everything into memory.
- Microservices Architecture Support: Node.js pairs well with microservices because of its lightweight runtime and high scalability. By separating functionalities into microservices, you can scale specific parts of the app independently, enhancing efficiency and stability.
- Built-in REPL (Read-Eval-Print Loop): Node.js offers a REPL environment that allows you to quickly test and experiment with JavaScript code snippets. This is especially useful for prototyping or debugging small pieces of code.
- Real-time Capabilities with WebSockets: Node.js is known for its real-time web application capabilities. With WebSocket libraries like socket.io, you can easily build real-time chat applications, multiplayer games, and collaborative platforms.
Categories of Node.js Modules
🌐Node.js comes with a powerful collection of built-in modules, which make it incredibly versatile. Here’s a breakdown of these modules, organized by category, to give you a clear view of what each one offers and how it can be used.
1. Cryptography and Security 🔒
These modules are essential for handling security and cryptography in your applications. They enable secure data handling, encrypted connections, and other protective measures.
- crypto: Provides cryptographic functionalities for encrypting and decrypting data, generating hashes, and securing passwords. Common methods include generating secure random numbers and creating HMACs (hash-based message authentication codes).
- tls: Stands for Transport Layer Security. This module helps you set up secure connections between the client and server, protecting data from eavesdropping.
- https: Built on top of http, this module helps create HTTPS servers with SSL/TLS encryption, essential for transmitting sensitive information securely.
2. File System and Operating System 🗄️
These modules let you interact directly with the file system and access system-level information, making it easier to manage files, directories, and system resources.
- fs (File System): Essential for file operations such as reading, writing, renaming, or deleting files. You can work with both synchronous and asynchronous file methods for maximum flexibility.
- path: This module simplifies working with file and directory paths, offering methods to resolve absolute paths, join paths, and parse file extensions, which helps you avoid path errors.
- os: Allows you to retrieve information about the operating system, such as CPU load, memory usage, uptime, and network interfaces. This data is particularly useful for monitoring and optimizing server performance.
3. Networking and Communication 🌐
Node.js includes modules to help you create and manage network-based applications, from simple HTTP servers to more complex protocols.
- http: Provides functionality to create HTTP servers and clients. This module is the backbone of web applications built on Node.js.
- https: The secure counterpart to http, enabling you to create HTTPS servers that encrypt data in transit.
- net: Supports low-level networking and TCP/IP connections, allowing you to build custom network protocols. Ideal for creating custom servers outside of HTTP/HTTPS.
- dns: Allows DNS (Domain Name System) operations, such as performing DNS lookups, resolving domains, and handling domain-related configurations.
- dgram: Implements UDP (User Datagram Protocol) sockets, which are useful for applications that prioritize speed over reliability, like live gaming or real-time communication.
4. Concurrency and Multi-Threading 🧵
These modules help you execute code concurrently in Node.js (Multithreading In Node.js), overcoming the limitations of its single-threaded architecture, especially for CPU-intensive tasks.
- cluster: Cluster Module Allows you to create child processes that share the same server ports, enabling load balancing across CPU cores for better performance.
- worker_threads: worker threads Provides support for running JavaScript code in multiple threads, making it ideal for handling heavy computation without blocking the main thread.
- child_process: Child process Allows you to spawn sub-processes, enabling you to execute shell commands, scripts, or other programs. Useful for offloading CPU-intensive tasks to other processes.
5. Error Handling and Domain Management ⚠️
Modules in this category help you manage errors, isolate code execution, and handle unexpected exceptions gracefully.
- errors: This module helps you customize error types, making it easier to handle specific error scenarios and provide detailed information in your code.
- domain: Offers a way to handle and manage exceptions across multiple I/O operations. Although now deprecated, it was once used for handling errors within isolated scopes, keeping the main application safe.
6. Events and Streams 🎬
Node.js’s event-driven architecture is powered by these modules, enabling asynchronous operations and handling data streams.
- events: Provides an event emitter class, which is core to Node.js’s asynchronous design. This module allows you to emit and handle custom events across your application.
- stream: Facilitates handling streaming data, which can be processed chunk-by-chunk rather than loading it all into memory. Essential for working with large files or data sources.
- buffer: Allows you to work with binary data in Node.js. Buffers are used when handling raw data, such as when working with files or networking streams.
- repl (Read-Eval-Print Loop): Provides a command-line interface to quickly test and debug JavaScript code, very useful for prototyping or experimenting with code snippets.
7. File Handling, Compression, and Data Manipulation 📄
Modules in this category focus on handling files, compressing data, and managing URLs in a more structured way.
- zlib: Provides compression and decompression utilities, supporting formats like gzip and deflate. Useful for reducing file sizes in data transfer.
- url: Parses and formats URLs, making it easy to work with URL strings and manipulate their components. Ideal for routing and handling query strings.
- querystring: Helps parse and format URL query strings, converting them to JavaScript objects and back to string format, useful for handling parameters in HTTP requests.
8. Utilities and General Purpose Modules 🛠️
Node.js also includes a variety of utility modules that assist with debugging, timing, and general-purpose functions, enhancing flexibility.
- util: Offers utilities like debugging functions and type-checking helpers. It includes useful methods like util.promisify() to convert callback-based functions into promises.
- timers: Provides functions such as setTimeout, setInterval, and setImmediate to manage the execution timing of code blocks.
- assert: A simple set of assertion tests, used primarily in debugging and testing. It verifies assumptions in code, throwing an error if a condition is not met.
9. TLS/SSL and Security Protocols 🔐
These modules support the creation and management of secure connections, vital for building applications that handle sensitive data.
- tls/ssl: Handles the low-level security protocols for secure network connections. It is essential for applications that need encryption, like secure web servers or data exchanges.
- https: Already mentioned but worth repeating here, as it’s the primary way to create a secure HTTP server using SSL/TLS.
Category | Module | Description |
---|---|---|
Cryptography & Security | crypto, https, tls | Handles encryption, secure connections, and secure data transfer. |
File System & OS | fs, path, os | Manages files, directories, and OS-level information. |
Networking | http, https, net | Supports server creation, networking, and HTTP/HTTPS connections. |
Concurrency | cluster, worker_threads, child_process | Enables multi-threading and concurrency handling. |
Error Handling | errors, domain | Provides error management and exception handling. |
Events & Streams | events, stream, buffer | Manages events, streams, and binary data handling. |
File Compression | zlib | Compresses and decompresses files in formats like gzip. |
Utilities | util, timers, assert | Provides debugging, type-checking, and timing functions. |
Security Protocols | tls/ssl, https | Secures data transfers and connections. |
🛠️ Common Use Cases of Node.js
Node.js is widely used across industries for various types of applications. Here are a few popular use cases:
- Real-Time Applications: Due to its non-blocking I/O model, Node.js is excellent for real-time apps like chat systems, collaboration tools, and live-streaming platforms.
- RESTful APIs: Node.js provides quick and efficient API creation, making it perfect for data-driven applications.
- Microservices: Its lightweight architecture makes it easy to break down complex applications into independently deployable services.
- Server-Side Rendering (SSR): With frameworks like Next.js, Node.js enables fast server-side rendering for SEO-optimized, high-performance web applications.
- IoT and Embedded Systems: Node.js is increasingly used in IoT due to its lightweight, event-driven model, which is ideal for devices with limited resources.
conclusion
Node.js is a powerhouse for building efficient, scalable applications thanks to its asynchronous, non-blocking architecture and a wide range of powerful core modules. Whether you’re managing complex cryptographic tasks, handling files and operating system functionalities, creating network applications, or performing advanced computations, Node.js provides the tools you need to do it effectively.
In this blog, we’ve taken a high-level tour of Node.js’s capabilities, categorizing the essential modules that make Node.js a flexible choice for developers in areas like security, file management, and networking. Each of these modules has unique functionalities designed to help streamline development, making Node.js ideal for creating everything from basic web servers to sophisticated, real-time applications.
Stay tuned for our upcoming posts, where we’ll dive deep into each module category—exploring specific use cases, examples, and best practices. With Node.js, the possibilities are vast, so keep exploring, experimenting, and building with confidence! 🚀
Important Links
- Understanding Processes and Threads in Node.js
- Event Driven Architecture in Node.js : Comprehensive Guide
- How to Implement API Rate Limiting in a NodeJS Express Application
- Mastering Asynchronous JavaScript: Callbacks, Promises, and Async/AwaitHow to use Multithreading in Node.js: A Comprehensive GuideHow to use Multithreading in Node.js: A Comprehensive Guide
FAQs
What is Node.js, and why is it popular?
Node.js is an open-source runtime environment that allows developers to execute JavaScript code on the server side. Its popularity comes from its non-blocking, asynchronous nature, making it highly efficient and perfect for handling multiple requests simultaneously, such as in web applications.
How does Node.js handle asynchronous operations?
Node.js uses an event-driven, non-blocking I/O model, which means it handles asynchronous operations with an event loop and callback functions. This architecture allows Node.js to manage tasks like file reading, network requests, and database operations without blocking the main thread.
What’s the difference between Node.js and JavaScript?
JavaScript is a programming language typically used on the client side (in browsers), while Node.js allows JavaScript to run on the server side. Node.js includes built-in modules for handling server-side tasks, like working with the file system, networks, and databases, which aren’t available in browser-based JavaScript.
Can I use Node.js for front-end development?
Not directly. Node.js is designed for backend development, but it’s commonly used alongside frontend frameworks like React, Angular, and Vue for full-stack applications. Developers often use Node.js for creating APIs, managing data, and handling the server side of applications.
What’s the difference between npm and Node.js?
Node.js is the runtime environment that allows JavaScript to run on the server, while npm (Node Package Manager) is a tool bundled with Node.js. npm lets you install and manage libraries and packages that you can use in your Node.js projects.